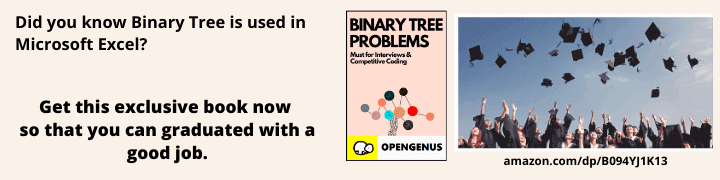
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Inheritance is a characteristic in which an object belonging to one class acquires the characteristics of another class and can pass on those characteristics to other classes. This type of relationship between class frameworks is known as inheritance. Multilevel inheritance is a form of inheritance where a base or child class has multiple parent classes and can be extended to any level. Hence, similar to other inheritance features such as hierarchical inheritance and multiple inheritances, we can deduce that base classes with multiple parent classes are referred to as Multilevel inheritance in C++.
To illustrate Multilevel inheritance in C++, let's consider the scenario where Grandfather is the base class. In this case, the derived class, Father, inherits the characteristics of Grandfather. Additionally, there is another derived class called Child, which inherits all the features of Father as it is derived from the subclass Father.
Syntax of Multilevel inheritance
class A // base class
{
...........
};
class B : acess_specifier A // derived class
{
...........
} ;
class C : access_specifier B // derived from derived class B
{
...........
} ;
This image demonstrates the concept:
Benfits of multilevel inheritance:
The primary benefit of multilevel inheritance is the ability to reuse code, which greatly enhances code reusability. By creating derived classes from existing derived classes, it becomes easier to develop specialized classes without the need to start from scratch. Moreover, multilevel inheritance supports the Polymorphism and Modularity features of C++, enabling objects to exhibit multiple forms.
This form of inheritance improves code efficiency, organization, and flexibility. However, it is important to exercise caution while designing class hierarchies to ensure they are easily maintainable.
Implementation
Take into account the provided C++ code that demonstrates the comprehensive implementation of multi-level inheritance:
#include <iostream>
using namespace std;
// Base class
class Grandfather {
public:
void displayGrandfather() {
cout << "I am the Grandfather." << endl;
}
};
// Derived class from Grandfather
class Father : public Grandfather {
public:
void displayFather() {
cout << "I am the Father." << endl;
}
};
// Derived class from Father
class Child : public Father {
public:
void displayChild() {
cout << "I am the Child." << endl;
}
};
int main() {
// Creating an object of Child class
Child obj;
// Calling member functions of all three classes
obj.displayGrandfather(); // Inherited from Grandfather class
obj.displayFather(); // Inherited from Father class
obj.displayChild(); // Defined in Child class
return 0;
}
Certainly! Here's an example code that illustrates the implementation of multilevel inheritance in C++:
#include <iostream>
using namespace std;
// Base class
class Grandfather {
public:
void displayGrandfather() {
cout << "I am the Grandfather." << endl;
}
};
// Derived class from Grandfather
class Father : public Grandfather {
public:
void displayFather() {
cout << "I am the Father." << endl;
}
};
// Derived class from Father
class Child : public Father {
public:
void displayChild() {
cout << "I am the Child." << endl;
}
};
int main() {
// Creating an object of Child class
Child obj;
// Calling member functions of all three classes
obj.displayGrandfather(); // Inherited from Grandfather class
obj.displayFather(); // Inherited from Father class
obj.displayChild(); // Defined in Child class
return 0;
}
In this example, we have a base class called Grandfather
, which is then inherited by the derived class Father
. Similarly, the derived class Child
inherits from the Father
class. Each class has its own member function to display its identity.
Inside the main()
function, we create an object of the Child
class named obj
. We can then call the member functions displayGrandfather()
, displayFather()
, and displayChild()
on this object to demonstrate the inheritance hierarchy. The output of the program will display the messages corresponding to each class's member function.
Note that the derived classes inherit the member functions from their base classes, allowing for code reuse and specialization at each level of the inheritance hierarchy.
Multi-level inheritance and multiple inheritance are two distinct concepts in C++.
- Multi-level Inheritance:
- Multi-level inheritance involves deriving a class from another derived class, forming a hierarchical inheritance structure.
- In this type of inheritance, a derived class becomes the base class for another derived class, creating multiple levels of inheritance.
- It promotes code reusability and allows for specialization at each level of the inheritance hierarchy.
- Example:
class Animal {
// Base class
};
class Mammal : public Animal {
// Derived class from Animal
};
class Dog : public Mammal {
// Derived class from Mammal
};
- Multiple Inheritance:
- Multiple inheritance involves deriving a class from more than one base class.
- In this type of inheritance, a derived class inherits the features and characteristics of multiple base classes.
- It allows for combining functionalities from different classes into a single derived class.
Example:
class Shape {
// Base class
};
class Color {
// Base class
};
class ColoredShape : public Shape, public Color {
// Derived class from Shape and Color
};
In summary, multi-level inheritance focuses on creating a hierarchical structure of derived classes, while multiple inheritance allows a derived class to inherit from multiple base classes simultaneously. Both inheritance types have their own advantages and considerations, and their usage depends on the specific requirements of the program or problem at hand.
Conclusion
In this article at OpenGenus, we explored the concept of multilevel inheritance, its syntax, and its implementation in C++. We also gained insights into the distinctions between multilevel inheritance and multiple inheritance in C++. Well done on reaching this point! Now, take a moment to acknowledge your progress and give yourself a well-deserved applause.