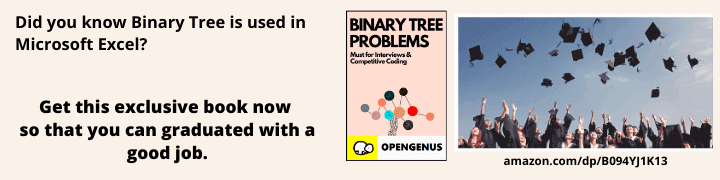
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | coding time: 15 minutes
C++ uses the concept of stream and stream classes to implement its I/O operation with the console and disk files. A stream is a sequence of bytes.There two types of stream in C++ :
- Input stream
- Output stream
A program extracts btyes from input stream and inserts btyes into output stream.
Output Stream: The stream used for output is the output stream. If the direction of flow of btyes is from main memory to device( example :display screen ) then this process is called output.
Header files available in C++ for Input – Output operation are:
- iostream: iostream stands for standard input output stream. This header file contains definitions to objects like cin, cout, cerr etc.
- iomanip: iomanip stands for input output manipulators. The methods declared in this files are used for manipulating streams. This file contains definitions of setw, setprecision etc.
- fstream: This header file mainly describes the file stream. This header file is used to handle the data being read from a file as input or data being written into the file as output.
Unformatted Output
We have cout which is an instance if ostream class.cout is used to produce output on the standard output device i.e the display screen. The data needed to be displayed on the screen is inserted in the standard output stream (cout) using the insertion operator (<<).The (<<) operator is overloaded to recognise all the basic C++ types.
Syntax
cout<<item_1<<item_2<<item_3.....<<itemN;
Example
char out1[] = "Welcome ";
cout << out1 <<"\n"<< "You are a part of GSSOC";
Output
Welcome
You are a part of GSSOC
Un-buffered standard error stream (cerr):
cerr is the standard error stream which is used to output the errors. The predefined object cerr is an instance of ostream class. The cerr object is said to be attached to the standard error device,i.e Display device.
As cerr is un-buffered so it is used when we need to display the error message immediately. It does not have any buffer to store the error message and display later.
The use of cerr is similar to the use of cout. The difference consists in the fact you use your error stream for output and not standard output stream:
Example
cerr << "There is some error in the program";
Output
There is some error in the program
buffered standard error stream (clog):
clog is the object of ostream class represents buffered standard error stream. The difference between buffered and un-buffered error stream consists in the fact that each insertion to clog object causes the output to be held in a buffer until the buffer is filled or flushed.
clog << "There is some error in the program";
Output
There is some error in the program
Note : The diffrence between cerr and clog may not be evident here but can be seen in larger programs.So it is a good practice to display error messages using cerr stream and while displaying other log messages then clog should be used.
Output in a file/Writing in a file
fstream is another C++ standard library like iostream and is used to read(take input) and write(give output) on files.
These are the data types used for file handling from the fstream library:
ofstream: It is used to create files and write on files.
ifstream: It is used to read from files.
fstream : It can perform the function of both ofstream and ifstream which means it can create files, write on files, and read from files.
Note: Make sure you always include header when you use files.
For files you want to write in, you need a file stream object.
Synatx
ofstream output_file_object;
or
fstream output_file_object;
Note : 1.Before doing any operation a file needs to be opened first (described in next syntax).
2.While using fstream object we need to open file in required mode for operation,i.e in output mode for writing into the file
You can write information to a file from your program using the stream insertion operator (<<) like we use that operator to output information to the screen. The only difference here is that you use an ofstream or fstream object instead of the cout object.
Syntax
ofstream output_file_object;
output_file_object.open("file_name.txt/dat");
char word[]="some string";
output_file_object<<word;
or
fstream output_file_object;
output_file_object.open("file_name.txt/dat", ios::out);
char variable_name[]="some string";
output_file_object<<variable_name;
Example
ofstream outFile;
outFile.open("file1.txt");
char str[]="I m a GSSOC19 participant";
outFile<<str;
cout<<"Output in file was successful!";
Output
Output in file was successful!
Formatted Output
C++ provides various formatted console I/O functions for formatting the output.
They can of three types-
1.Ios class functions and flags
2.Manipulators
3.User-defined output functions
Some Ios class functions are-
- width() : to set required field width.The output would be displayed in given width
- precision() : to set no. of decimal point to float value.
- fill() : to set a character to fill in blank spaces of width
- setf() : to set various flags for formatting outputs
- unsetf() : to remove flags
Example
cout.width(5);
cout<<"A";
Output
_ _ _ _A
Note : The output A is displayed on the 5th column as width is set to 5 and('_' represents blank space).
Manipulators
Outputs can be formatted using another C++ standard library, known as iomanip.This library offers the programmer several input/output manipulators,Such as -setw(),setprecision(),setf(),etc.
Some manipulators-
- setw() : manipulators sets the width of the field assigned for the output. It takes the size of the field (in number of characters) as parameter.
- setprecision(): manipulator sets the total number of digits to be displayed when floating-point numbers are printed
- setf() : to set flags
Example
cout<<setw(6)<<"X"<<endl;
cout<<setprecision(5)<<123.456<<endl;
cout.setf(ios::fixed);
cout<<setprecision(5)<<12.345678;
Output
_ _ _ _ _X
123.45
12.34567
Note: In first output,X is displayed after 5 blankspaces on 6th position and ('_' represents blank space).
In Second output, precision is 5 total no of didgits displayed is 5,(notice the rounding off).
In third output ,once the flag has been set, the number you pass to setprecision() is the number of decimal places you want displayed.