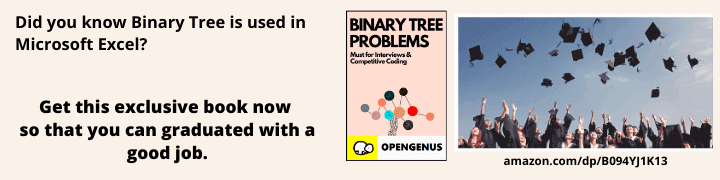
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored how to create array of user-defined objects in C++ Programming Language. We have covered 3 different approaches with complete C++ code.
Table of contents:
- Array of objects in C++
- C++ example
- C++ example with new operator
- C++ example with malloc
Pre-requisites:
Array of objects in C++
In C++, an array of objects is a collection of objects of the same class type that are stored in contiguous memory locations. Since each item in the array is an instance of the class, each one's member variables can have a unique value. This makes it possible to manage and handle numerous objects by storing them in a single data structure and giving them similar properties and behaviours.
No memory or storage is allocated when a class is defined; only the object's specification is defined. We must create objects in order to use the data and access the class's defined functions.
We can think of array of objects as a single variable that can hold multiple values. Each value is stored in a separate element of the array, and each element can be accessed by its index.
Arrays in C++ are typically defined using square brackets [] after the type. The index of the array, which ranges from 0 to n - 1, can be used to access each element.
Syntax for declaring an array of objects in C++:
class className {
//variables and functions
};
className arrayName[arraySize];
where,
className is the name of the class that the objects belong to.
arrayName is the name of the array of objects.
arraySize is the number of objects in the array or the size of array, specified as a constant expression.
C++ example
In this example, more than one Book's details with a Book name and cost can be stored.
Book bk[100] – This is an array of objects having a maximum limit of 100 Books.
First for loop is used to take the input from user by calling bk[i].getdetails() function and second one to print the details of Books by calling the function bk[i].putdetails() function.
#include<bits/stdc++.h>
using namespace std;
class Book
{
public:
char name[50];
int cost;
void getdetails();
void putdetails();
};
void Book::getdetails()
{
cout << "Enter Name : ";
cin >> name;
cout << "Enter Cost : ";
cin >> cost;
}
void Book::putdetails()
{
cout << name << " ";
cout << cost << " ";
cout << endl;
}
int main()
{
Book bk[100];
int n, i;
cout << "Enter Number of Books - ";
cin >> n;
for(i = 0; i < n; i++){
bk[i].getdetails();
}
cout << "Details of Books - " << endl;
for(i = 0; i < n; i++){
bk[i].putdetails();
}
return 0;
}
Output:
Enter Number of Books - 4
Enter Name : Atomic Habits
Enter Cost : 200
Enter Name : Ikigai
Enter Cost : 150
Enter Name : Deep Work
Enter Cost : 160
Enter Name : Mindset
Enter Cost : 165
Details of Books -
Atomic Habits 200
Ikigai 150
Deep Work 160
Mindset 165
Note: The array's size must be a constant expression, which means it must be predetermined at compile time and cannot be changed dynamically at runtime.
C++ example with new operator
We can also use the new operator to dynamically allocate memory for an array of objects. Here is an example:
An array of Number objects are dynamically allocated memory in this example using the new operator. The array's size can be adjusted at runtime, giving it greater flexibility than using a fixed-size array. When dynamically allocated memory is no longer required, it can be deallocated using the delete[] operator.
#include <iostream>
using namespace std;
class Number {
public:
int x;
int y;
};
int main() {
Number *arr = new Number[4];
for (int i = 0; i < 4; i++) {
arr[i].x = i + 1;
arr[i].y = i + 2;
}
for (int i = 0; i < 4; i++) {
cout << "x: " << arr[i].x << " y: " << arr[i].y << endl;
}
delete[] arr;
return 0;
}
C++ example with malloc
We can also allocate memory for an array of objects using malloc. When we use the malloc function to allocate memory for an array of objects, the function returns a pointer to the first byte of the memory that was created. The number of bytes allocated is equal to the size of each object multiplied by the number of objects in the array.
Here's an example implementation of an array of objects using malloc and freeing the memory afterwards:
In this example, we begin by creating a class called objt with just one member value. Then, we use malloc to allocate memory for n objects of type objt, and if the resulting pointer array is not NULL, we know the allocation was successful. If the allocation was successful, the array of objects is available for use as needed. Finally, we release the memory that malloc allocated using the free function.
#include <bits/stdc++.h>
using namespace std;
class objt{
int value;
};
int main() {
int n = 10;
objt *arr = (objt *)malloc(n * sizeof(objt));
if (arr == NULL) {
cout<<"Error in memory allocation"<<endl;
exit(1);
}
free(arr);
return 0;
}
With this article at OpenGenus, you must have the complete idea of Array of objects in C++.