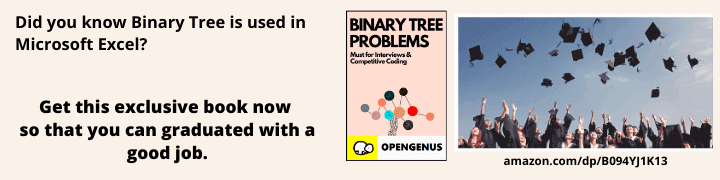
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 45 minutes | Coding time: 5 minutes
The main purpose of C++ programming is to add object orientation to the C programming language and classes are the central feature of C++ that supports object-oriented programming and are often called user-defined types
.
A class is a mechanism for creating user-defined data types. It is similar to the C language structure data type. In C, a structure is composed of a set of data members. In C++, a class type is like a C structure, except that a class is composed of a set of data members and a set of operations that can be performed on the class.
In other words, these are the building block of C++ that leads to Object Oriented programming.It is a user defined data type, which holds its own data members and member functions, which can be accessed and used by creating an instance of that class. A class is like a blueprint
for an object.
A class is used to specify the form of an object and it combines data representation and methods for manipulating that data into one neat package. The data and functions within a class are called members of the class.That means, the variables inside class definition are called as data members and the functions are called member functions.
All in all, it can also be said that a class is a way to bind the data and its associated functions together in a single unit. This process is called Encapsulation.
Example-1:
Class of birds, all birds can fly and they all have wings and beaks. So here flying is a behavior and wings and beaks are part of their characteristics. And there are many different birds in this class with different names but they all posses this behavior and characteristics.
That means, a Class is just a blue print, which declares and defines characteristics and behavior, namely data members and member functions respectively. And all objects of this class will share these characteristics and behavior.
Example-2:
Consider the Class of Cars. There may be many cars with different names and brand but all of them will share some common properties like all of them will have 4 wheels, Speed Limit, Mileage range etc. So here, Car is the class and wheels, speed limits, mileage are their properties.
Here, the data member will be speed limit, mileage etc and member functions can be apply brakes, increase speed etc.
C++ Class
When we define a class, we define a blueprint for a data type. This doesn't actually define any data, but it does define what the class name means, that is, what an object of the class will consist of and what operations can be performed on such an object.
A class definition starts with the keyword class followed by the class name; and the class body, enclosed by a pair of curly braces. A class definition must be followed either by a semicolon or a list of declarations. For example, we defined the Box data type using the keyword class as follows −
class Student
{
public:
int id; //field or data member
float salary; //field or data member
String name; //field or data member
};
In the above example, the keyword public determines the access attributes of the members of the class that follows it. A public member can be accessed from outside the class anywhere within the scope of the class object. We can also specify the members of a class as private or protected.
C++ Objects
An Object is an instance
of a Class. When a class is defined, no memory is allocated but when it is instantiated (i.e. an object is created) memory is allocated.
Objects are instances of class, which holds the data variables declared in class and the member functions work on these class objects.
Each object has different data variables. Objects are initialised using special class functions called Constructors
.
And whenever the object is out of its scope, another special class member function called Destructor
is called, to release the memory reserved by the object. C++ doesn't have Automatic Garbage Collector like in JAVA, in C++ Destructor performs this task.
In C++, object is a group of similar objects. It is a template from which objects are created. It can have fields, methods, constructors etc.
We declare objects of a class with exactly the same sort of declaration that we declare variables of basic types. Following statements declare two objects of any class Box −
Box Box1; // Declare Box1 of type Box
Box Box2; // Declare Box2 of type Box
Both of the objects Box1 and Box2 will have their own copy of data members.
Syntax :
ClassName ObjectName;
Accessing data members and member functions :
The data members and member functions of class can be accessed using the dot(‘.’)
operator with the object.
1. Accessing data members :-
Example-1 : if the name of object is obj and you want to access the member function with the name printName() then you will have to write obj.printName().
Lets now, understand through code :-
Example-2:
# include<iostream>
using namespace std;
class Box
{
public:
double length; // Length of a box
double breadth; // Breadth of a box
double height; // Height of a box
};
int main()
{
Box Box1; // Declare Box1 of type Box
Box Box2; // Declare Box2 of type Box
double volume = 0.0; // Store the volume of a box here
// box 1 specification
Box1.height = 5.0;
Box1.length = 6.0;
Box1.breadth = 7.0;
// box 2 specification
Box2.height = 10.0;
Box2.length = 12.0;
Box2.breadth = 13.0;
// volume of box 1
volume = Box1.height * Box1.length * Box1.breadth;
cout << "Volume of Box1 : " << volume << endl;
// volume of box 2
volume = Box2.height * Box2.length * Box2.breadth;
cout << "Volume of Box2 : " << volume << endl;
return 0;
}
Output :
The output of above example-2 is as follows :
Volume of Box1 : 210
Volume of Box2 : 1560
NOTE :- Private and Protected members can not be accessed directly using direct member access operator (.).
Example-3: Initialize and Display data through method
# include <iostream>
using namespace std;
class Employee
{
public:
int id; //data member (also instance variable)
string name; //data member(also instance variable)
float salary;
void insert(int i, string n, float s)
{
id = i;
name = n;
salary = s;
}
void display()
{
cout << id << " " << name << " " << salary << endl;
}
};
int main(void)
{
Employee e1; //creating an object of Employee
Employee e2; //creating an object of Employee
e1.insert(201, "Sonoo",990000);
e2.insert(202, "Nakul", 29000);
e1.display();
e2.display();
return 0;
}
Output :
201 Sonoo 990000
202 Nakul 29000
2. Member Functions :
A member function of a class is a function that has its definition or its prototype within the class definition like any other variable. It operates on any object of the class of which it is a member, and has access to all the members of a class for that object.
There are 2 ways to define a member function:
- Inside class definition
- Outside class definition
Defining a member function within the class definition declares the function inline, even if we do not use the inline specifier.
If the member function is defined inside the class definition it can be defined directly, but if its defined outside the class, then we have to use the scope resolution ::
operator along with class name alng with function name.
1. Inside class definition :
If we define the function inside class then we don't not need to declare it first, we can directly define the function.
Example -
#include<iostream>
using namespace std;
class Cube
{
public:
int side=10;
void getVolume()
{
cout<< side*side*side; //prints volume of cube
}
};
int main()
{
Cube obj;
obj.getVolume()
return 0;
}
Output :-
1000
2. Outside class definition :
But if we plan to define the member function outside the class definition then we must declare the function inside class definition and then define it outside.
Example -
#include<iostream>
using namespace std;
class Cube
{
public:
int side=10;
int getVolume();
}
// member function defined outside class definition
void Cube :: getVolume()
{
cout<< side*side*side;
}
int main()
{
Cube obj;
obj.getVolume()
return 0;
}
Output :-
1000
NOTE :-The main function for both the function definition will be same. Inside main() we will create object of class, and will call the member function using dot . operator.
Calling Class Member Function in C++
# include <iostream>
using namespace std;
class Box {
public:
double length; // Length of a box
double breadth; // Breadth of a box
double height; // Height of a box
// Member functions declaration
double getVolume(void);
void setLength( double len );
void setBreadth( double bre );
void setHeight( double hei );
};
// Member functions definitions
double Box::getVolume(void) {
return length * breadth * height;
}
void Box::setLength( double len ) {
length = len;
}
void Box::setBreadth( double bre ) {
breadth = bre;
}
void Box::setHeight( double hei ) {
height = hei;
}
// Main function for the program
int main() {
Box Box1; // Declare Box1 of type Box
Box Box2; // Declare Box2 of type Box
double volume = 0.0; // Store the volume of a box here
// box 1 specification
Box1.setLength(6.0);
Box1.setBreadth(7.0);
Box1.setHeight(5.0);
// box 2 specification
Box2.setLength(12.0);
Box2.setBreadth(13.0);
Box2.setHeight(10.0);
// volume of box 1
volume = Box1.getVolume();
cout << "Volume of Box1 : " << volume << endl;
// volume of box 2
volume = Box2.getVolume();
cout << "Volume of Box2 : " << volume << endl;
return 0;
}
Output :-
Volume of Box1 : 210
Volume of Box2 : 1560
Note:- note that all the member functions defined inside the class definition are by default inline, but you can also make any non-class function inline by using keyword inline with them. Inline functions are actual functions, which are copied everywhere during compilation, like pre-processor macro, so the overhead of function calling is reduced. Declaring a
friend function
is a way to give private access to a non-member function.
Types of Member Functions :-
- Simple functions
- Static functions
- Const functions
- Inline functions
- Friend functions
1. Simple Member functions :-
These are the basic member function, which dont have any special keyword like static etc as prefix. All the general member functions, which are of below given form, are termed as simple and basic member functions.
Syntax :
return_type functionName(parameter_list)
{
function body;
}
2. Static Member functions :-
Static is something that holds its position. Static is a keyword which can be used with data members as well as the member functions. We will discuss this in details later. As of now we will discuss its usage with member functions only.
A function is made static by using static
keyword with function name. These functions work for the class as whole rather than for a particular object of a class.
These functions cannot access ordinary data members and member functions, but only static
data members and static member functions can be called inside them.
It doesn't have any "this"
keyword which is the reason it cannot access ordinary members
It can be called using the object and the direct member access .
operator. But, its more typical to call a static member function by itself, using class name and scope resolution ::
operator.
example :-
class X
{
public:
static void f()
{
// statement
}
};
int main()
{
X::f(); // calling member function directly with class name
}
3. Const Member functions :-
Const keyword makes variables constant, that means once defined, there values can't be changed.When used with member function, such member functions can never modify the object or its related data members.
Syntax :-
void fun() const
{
// statement
}
Example -
#include <iostream>
class StarWars
{
public:
int i;
StarWars(int x) // constructor
{
i = x;
}
int falcon() const // constant function
{
/*
can do anything but will not
modify any data members
*/
cout << "Falcon has left the Base";
}
int gamma()
{
i++;
}
};
int main()
{
StarWars objOne(10); // non const object
const StarWars objTwo(20); // const object
objOne.falcon(); // No error
objTwo.falcon(); // No error
cout << objOne.i << objTwo.i;
objOne.gamma(); // No error
objTwo.gamma(); // Compile time error
}
4. Inline functions :-
All the member functions defined inside the class definition are by default declared as Inline.
Inline functions are actual functions, which are copied
everywhere during compilation, like preprocessor macro, so the overhead of function calling is reduced
. All the functions defined inside class definition are by default inline, but you can also make any non-class function inline by using keyword inline with them.
For an inline function, declaration and definition must be done together. For example -
inline void fun(int a)
{
return a++;
}
Example -
#include <iostream>
inline int Add(int x,int y)
{
return x+y;
}
void main()
{
cout<<"\n\tThe Sum is : " << Add(10,20);
cout<<"\n\tThe Sum is : " << Add(45,83);
cout<<"\n\tThe Sum is : " << Add(27,48);
}
Output :
The Sum is : 30
The Sum is : 98
The Sum is : 75
Important Points About Inline Functions :-
- We must keep inline functions small, small inline functions have better efficiency.
- Inline functions do increase efficiency, but we should not make all the functions inline. Because if we make large functions inline, it may lead to code bloat, and might affect the speed too.
- Hence, it is adviced to define large functions outside the class definition using scope resolution :: operator, because if we define such functions inside class definition, then they become inline automatically.
- Inline functions are kept in the Symbol Table by the compiler, and all the call for such functions is taken care at compile time.
5. Friend functions :-
Friend functions are actually not a class member function. Friend functions are made to give private access to non-class functions. We can declare a global function as friend, or a member function of other class as friend.
When we make a class as friend, all its member functions automatically become friend functions.
Friend Functions is a reason, why C++ is not called as a pure Object Oriented language. Because it violates the concept of Encapsulation.
Syntax:-
class class_name
{
friend data_type function_name(argument/s);
};
Characteristics of a Friend function:
- The function is not in the scope of the class to which it has been declared as a friend.
- It cannot be called using the object as it is not in the scope of that class.
- It can be invoked like a normal function without using the object.
- It cannot access the member names directly and has to use an object name and dot membership operator with the member name.
- It can be declared either in the private or the public part.
Example-1:
#include<iostream>
using namespace std;
class Box
{
private:
int length;
public:
Box(): length(0) { }
friend int printLength(Box); //friend function
};
int printLength(Box b)
{
b.length += 10;
return b.length;
}
int main()
{
Box b;
cout << "Length of box: " << printLength(b) << endl;
return 0;
}
Output :-
Length of box: 10
Example when the function is friendly to two classes :-
#include <iostream>
class B; // forward declarartion.
class A
{
int x;
public:
void setdata(int i)
{
x=i;
}
friend void min(A,B); // friend function.
};
class B
{
int y;
public:
void setdata(int i)
{
y=i;
}
friend void min(A,B); // friend function
};
void min(A a,B b)
{
if(a.x <= b.y)
std::cout << a.x<< std::endl;
else
std::cout<< b.y<< std::endl;
}
int main()
{
A a;
B b;
a.setdata(10);
b.setdata(20);
min(a,b);
return 0;
}
Output :-
10