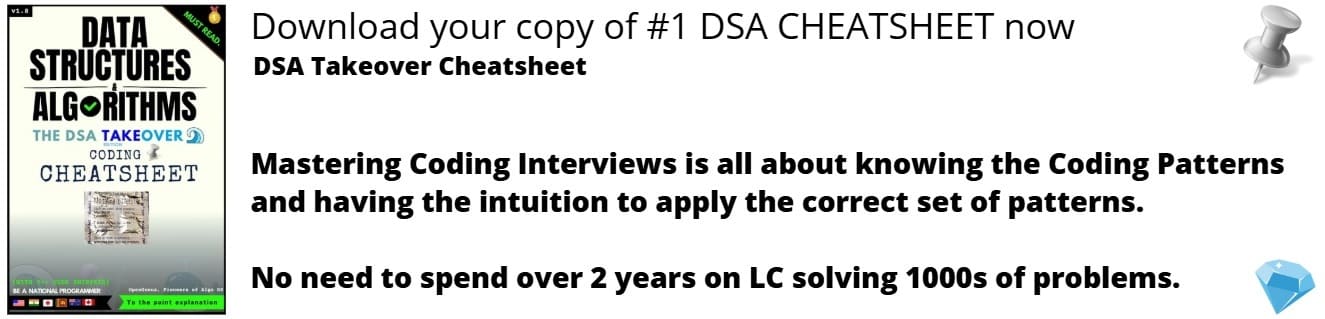
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 5 minutes
Controlling the flow of execution based on certain conditions is the most basic (and equally powerful) logic we can give to our programs. Let's look at the pythonic way of doing this. You may already be familiar with some of this syntax in other languages.
if else statements are the commonly used control statements and are available in most popular languages like Python, Java, C, C++ and others. We will take a deep look into if else statements in Python.
The if
statement
In simple language, an if statement could be read as
If this statement is true execute the indented code.
The Syntax is as follows
if condition:
# statements to be executed
Example:
year = 2101
if year == 2101:
# above condition evaluates to True
print("Welcome to the future!")
print("Make the most out of it.")
Output:
Welcome to the future!
Make the most out of it.
First of all, let's talk about the condition part. What can we put in there?
Any condition is just an expression that evaluates to a boolean. To know more let's take a deep dive into the world of comparison operators and boolean values.
Following this, we will look into:
- else part
- else if (elif) part
- nested if else
Mastering the basics of condition
Boolean Values
A boolean is a data type that can have only two values i.e., True
or False
. We can mix and match booleans in different ways and perform many operations on them. Booleans can be stored in a variable like any other data type. Try typing this in a python terminal.
>>> hello = True
>>> print(hello)
True
>>> type(hello)
<class 'bool'>
Note: The T in True and F in False must be capital. The type()
function returns the class of the argument passed to it. Pretty useful if you are not sure what kind of value a variable stores.
Comparing two values
Comparison Operators are used to compare two values. They compare left and right side of the operator and evaluates it to a single boolean.
Some common comparison operators
Operator | Description |
---|---|
== | equal to |
!= | not equal to |
< | less than |
> | greater than |
<= | less than or equal to |
>= | greater than or equal to |
Operations on Boolean values
Operator | Description |
---|---|
and | evaluates to True if both boolean values are True otherwise evaluates to False |
or | evaluates to False if both boolean values are False otherwise evaluates to True |
not | works on only one operand, evaluates to opposite of the boolean value |
Getting our hands dirty
With the knowledge of booleans and comparison operators try the following in a python terminal.
>>> not True
False
>>> (10 == 10) and (10 == 20)
False
>>> (18 < 15) or (15 < 18)
True
Now that you know the prerequisites here is a simple example of if statement in action.
year = 2101
if year == 2101:
# above condition evaluates to True
print("Welcome to the future!")
print("Make the most out of it.")
Output:
Welcome to the future!
Make the most out of it.
The else
part
The if statement can optionally be followed by an else block. It allows us to define what to do if the condition in if part is not true. In simple language else statement can be read as:
If this condition is true, execute this code. Or else, execute that code.
Syntax:
if condition1:
code statements
else:
code statements
Here is an example:
year = 2019
if year >= 2101:
print("Welcome to the future!")
else:
print("Dang! You are still in 21th century.")
print("Make the most out of it.")
Output
Dang! You are still in 21th century.
Make the most out of it.
Take care of the indentation while writing python code else you will get errors and logic may not work as you want. Also, this promotes clean code practices which is easier to understand and debug later.
The elif
(else if) statement
Alternatively, we may want to check against multiple cases sequentially if the previous ones fail. We can stack elif statement to create an if-elif ladder.
Syntax:
if condition1:
code statements
elif condition2:
code statements
else:
code statements
An example will make it more clear.
number = int(input("Enter a number between 1 and 5: "))
if number == 1:
print("You entered one.")
elif number == 2:
print("You entered two.")
elif number == 3:
print("You entered three.")
elif number == 4:
print("You entered four.")
elif number == 5:
print("You entered five.")
else:
print("Out of range. Try again.")
The above code is similar to switch statement in C. Note that the last else acts as a default case. It ensures that atleast one of the block of elif ladder executes. Also the order in which we write elif conditions matter. Note that we need to typecast the input given by user into int as input function returns a string. Comparing a string with integer will result in a false.
Output
Enter a number between 1 and 5: 3
You entered three.
Nested if else
Multiple if statements can be put inside other if else blocks to create more complex logic. Here is a program to find the largest of three numbers using nested if else.
a, b, c = 20, 4, 7
if a >= b:
if a >= c:
print("A is largest number.")
else:
print("C is largest number.")
else:
if b >= c:
print("B is largest number.")
else:
print("C is largest number.")
Output
A is largest number.
That's it folks! With this knowledge under your belt you can do all types of shenanigans.