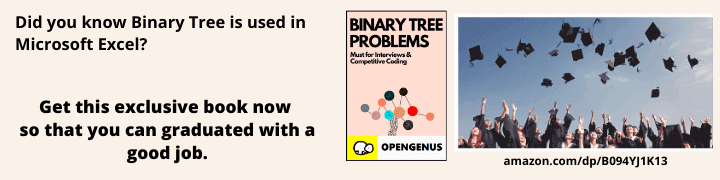
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Arrays: A simple way is to represent the linear relationship between the elements represented using sequential memory locations. We have covered two types of arrays:
- standard Array declaraction
- Array container in Standard Template Library (STL) in C++
Different ways to initialize an array in C++ are as follows:
- Method 1: Garbage value
- Method 2: Specify values
- Method 3: Specify value and size
- Method 4: Only specify size
- Method 5: memset
- Method 6: memcpy
- Method 7: wmemset
- Method 8: memmove
- Method 9: fill
- Method 10: Techniques for Array container
type name[size]={};
A linear array is a list of a finite number of homogeneous data elements such that:
a. All elements are indexed.
b. Elements are stored in successive memory locations.
To obtain the length of data elements of the array we can subtract the largest index by the smallest called as upper bound UB and lower bound LB respectively and adding 1.
Length = UB - LB + 1
To understand it more clearly let's take this question:
Understanding Array Sizes
Let's say an Array A has the following elements A1, A2, A3, . . . . An. Now calculate the Length of the array by the above given formula.
UB is = n
LB is = 1
so as Length = UB - LB +1
we obtain,
Length = n -1 +1 = n
Hence The option 'n'
Notation of arrays
We usually use bracket notation i.e. A[1] ,A[2] , . . . ,A[n]
another way is using parenthesis notation like in FORTRAN, PL/1, and BASIC
Rules for declaration
Irrespective of the programming language we must give three basic items of information while building an array:
- Name of array
- Data type to be stored in it.
- The index set of array i.e. size
Representation in Memory
Let's consider an array OpenGenus in the memory of the computer. So as we know memory locations allocated would be successive to the elements of the array as shown here.
If we know the base address of the array we can easily figure out the address of any location of the index of the array by simply using this method.
Location OpenGenus[k] = BaseLocation(OpenGenus[0]) + w x(k - LowerBound)
w = Number of words per memory cell
Initializing an array:
A simple way to initialize an array is by following the basic rules:
Method 1: Garbage allocation
int OpenGenusArray[10];
Here we gave type as int, name as OpenGenusArray, and size as 10. In this case, the values within the array are garbage value. In other Programming Languages like Java, garbage value is replaced by a default value like 0.
Method 2: Specify value
double OpenGenusArray[] ={1.7,2.0,3.5};
Here we gave type as double, name as OpenGenusArray, and size as 3
Method 3: Specify value and size
int OpenGenusArray[5] ={5,4,3,2,1};
Here we gave type as int, name as OpenGenusArray, and size as 5
Method 4: Specify size and NULL
bool OpenGenusArray[5] ={};
Here we gave type as bool, name as OpenGenusArray, and size as 5
Some other Advance topics with Arrays:
Method 5: memset
Takes in element, the value and size n to fill and consequently fills the first n bytes of the element given by the value.
char str[] = " .opengenus.org";
memset (str,'w',3);
This gives output as
www.opengenus.org
Method 6: memcpy
It copies the value given n bytes from source to destination memory block.
char src[50] = "Welcome to OpenGenus";
char dest[50]="Welcome";
cout<<"Initially destination = "<<dest<<"\n";
memcpy(dest, src, strlen(src)+strlen(dest)+1);
cout<<"After memcpy destination = "<<dest<<"\n";
It's Output is:
Initially destination = Welcome
After memcpy destination = Welcome to OpenGenus
Method 7: wmemset
In simpler terms it's the wide character equivalent of memset.
wchar_t wideChars[] = L" Wide character is character datatype that has a size greater than the 8-bit character!";
wmemset (wideChars,L'-',6);
wprintf (L"%ls\n",wideChars);
It's Output:
------Wide character is character datatype that has a size greater than the 8-bit character!
Method 8: memmove
It copies in a fashion such that it depicts the usage of an intermediate buffer allowing source and destination to overlap using given n bytes from target to souce memory block.
char dest[] = "Computer Science is fun ";
char src[] = "Computer Science is super fun";
cout<<"Initially target = "<<dest<<"\nAnd source = "<<src<<"\n";
memmove(dest, src, 29);
cout<<"After memmove target = "<<dest<<"\nAnd source = "<<src<<"\n";
It's Output
Initially target = Computer Science is fun
And source = Computer Science is super fun
After memmove target = Computer Science is super fun
And source = Computer Science is super fun
Method 9: fill
It fills the array with the given element. It must be of same type else it would interprete it's integer value only and it will accept only a single digit.
array<int, 5> filledArray;
filledArray.fill(007);
for(int x:filledArray){
cout<<x<<" ";
}
It's output:
7 7 7 7 7
Method 10: Array container in C++
Now we will look into Array container in C++: These are better then standart builtin arrays we are provided both in management and memory and efficiency.
For this we must include the header file
#include <array>
Here are the basics for using std:array<type,size>
#include <array>
#include <iostream>
using namespace std;
int main(){
// Creating
std::array<int, 10> containerArray;
std::array<int, 3> anotherContainerArray= {2, 4, 1};
// Traversal
for(int x:anotherContainerArray){
cout<<x<<" ";
}
cout<<"\n";
// Sorting
sort(anotherContainerArray.begin(),anotherContainerArray.end());
// .size() tells the number of elements
cout<<anotherContainerArray.size()<<"\n";
// Accessing with index
cout<<"Element at anotherContainerArray[0]: "<<anotherContainerArray[0]<<"\n";
cout<<"Element at anotherContainerArray[1]: "<anotherContainerArray.at(1)<<"\n";
for(int x:anotherContainerArray){
cout<<x<<" ";
}
return 0;
}
Output of the code
2 4 1
3
Element at anotherContainerArray[0]: 1
Element at anotherContainerArray[1]: 2
1 2 4
Let's see a program for the above
#include <iostream>
#include <array>
#include <bits/stdc++.h>
using namespace std;
int main(){
int openGenusArray[10];
char dest[] = "Topic you are reading ";
char src[] = "Different ways to initialize an array in C++ ";
memmove(dest, src, 50);
cout<<dest<<"\n";
// Filling up array
for (int i = 0; i < 10; i++) {
openGenusArray[i]=i;
}
cout<<"Initial Array:"<<"\n";
for(int x:openGenusArray){
cout<<x<<" ";
}
cout<<"\n";
int i=0,j=9;
while(true){
swap(openGenusArray[i],openGenusArray[j]);
i++;
j--;
if(i>=j)break;
}
cout<<"Final Array:"<<"\n";
for(int x:openGenusArray){
cout<<x<<" ";
}
cout<<"\n"<<"Do Visit:";
char str[] = " .opengenus.org";
memset (str,'w',3);
puts(str);
return 0;
}
Output here is
Different ways to initialize an array in C++
Initial Array:
0 1 2 3 4 5 6 7 8 9
Final Array:
9 8 7 6 5 4 3 2 1 0
Do Visit:www.opengenus.org