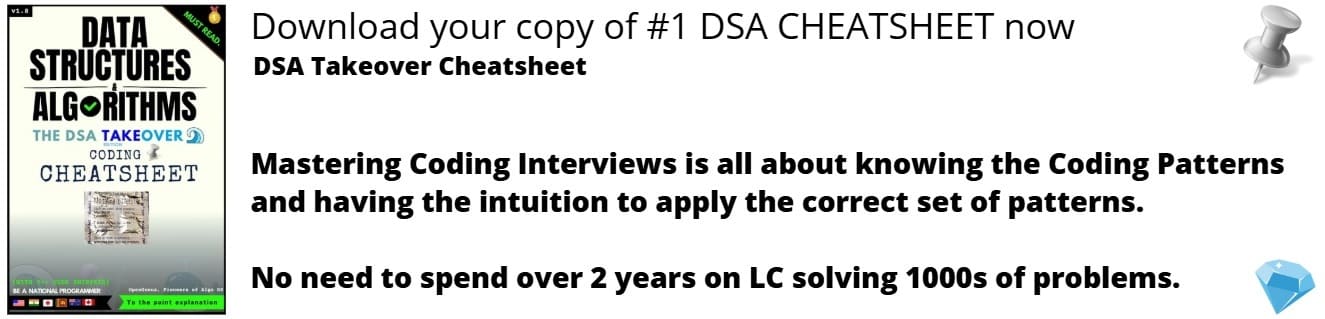
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 10 minutes
The most commonly used features of STL are :
1. Iterator
As described earlier, they are the tools used to access and iterate the values in the container class. To be specific, they are objects of the container class which are used to iterate through the container. Pointers are infact a type of iterators.
*Syntax* : < container class > < data-type > :: iterator < iterator name >
Example : stack < int > :: iterator itr;
2. Vector
It falls under the category of containers and is similar to array except the fact that its size can be changed or resized. It is often called dynamic array.
// Code Snippet
vector < int > v;
vector < int >::iterator it;
v.push_back(1); // inserting 1 to vector v
v.push_back(2); // inserting 2 to vector v
// printing the vector contents
for(it = v.begin(); it != v.end();++it)
cout << *it << ' ';
// Output: 1 2
3. Stack
STL contains the implementation of Stack the LIFO data structure.It consists of push, pop, top, empty and size functions.
// Code Snippet
// declaration of stack
stack < int > s;
// inserting elements in stack.
for(int i = 0;i < 5; i++)
s.push(i); // 0 1 2 3 4
//size of stack s
cout<< ”Size of stack is: ” < < s.size( ) << endl; // 5
// top element from stack, it will be the last inserted element.
cout<<”Top element of stack is: ” << s.top( ) << endl ;
// deleting all elements from stack
for(int i = 0;i < 5;i++)
s.pop( ); // 4 3 2 1 0
4. Queue
STL also contains the FIFO data structure called as Queue.It consist of push, pop, front, empty and size functions.
// Code Snippet
queue < int > q;
for(int i = 0;i < 4;++i)
q.push(i); // inserting elements to queue q
while(!q.empty())
{
cout << q.front(); // 0 1 2 3
q.pop();
}
5. Priority Queue
Heaps are implemented in STL in the forms of Min-Heap and Max-Heap priority queueus which are used to extract the lowest and highest element in constant time O(1)
// Code Snippet
priority_queue < int > pq; // Max-heap priority queue
// inserting elements in priority queue
pq.push(10);
pq.push(22);
pq.push(6);
while(!pq.empty())
{
cout << pq.top() << " ";
pq.pop();
}
Output : 22 10 6
6. Map
It is a container which is used to store data of the form of key value pairs. Thus there is a one to one mapping and the key is used to uniquely identify the particular value.
// Code Snippet
// Mapping a,b,c.. with 1,2,3 etc.
map < char,int > mp;
mp['a'] = 1;
mp['b'] = 2;
mp['c'] = 3;
7. Set
Set is a container which is used to store only unique elements in it unlike vectors or arrays which store duplicates as well.
// Code Snippet
set < int > s;
set < int > ::iterator itr;
int A[] = {1, 2, 2, 1, 2, 3};
for(int i = 0;i < 6;++i)
s.insert(A[i]);
for(it = s.begin();it != s.end();++it)
cout << *it << ' '; // Output : 1 2 3
8. Pair
Pair is a container that can be used to combine together two values which may be of different types. Pair thus provides a way to store two heterogeneous objects as a single unit.
// Code Snippet
pair < string, int > p;
p = make_pair("Vimal", 97745');
cout << p.first << ' ' << p.second << endl;