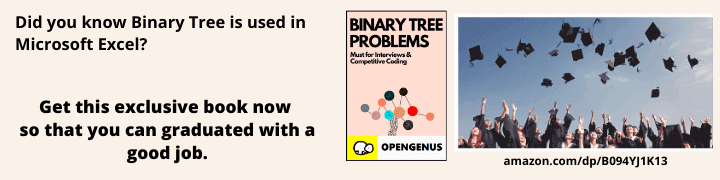
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In simple words, Control Flow is the order in which the statements written in a program are executed when a program is compiled. They are also referred to as Flow Control Statements.
In DART Programming Language the code statements are executed from the Top to Bottom.
The Control Flow Statemts in DART are of mainly three types. They are,
1. Iteration Statements
2. Selection Statements or Condtional Statements
3. Jump Statements
1. Iteration Statements
teration Statements are those which are used to execute set of statements repeatedly until a particular conditon is fulfilled or satisfied. They are also commonly referred to as looping statements.
The following are the Iteration Statements in DART
- For Loop (Definite Loop)
- While Loop ( Indefinite Loop)
- Do While Loop (Indefinte Loop)
For Loop
For Loop is a Definite Loop (One where the number of iterations are constant) it checks for conditions specified and used to execute the statements for a specified number of times.
The syntax of for loop in Dart is :
for (Initialization; Condition; Increment/Decrement)
{
// statement block
}
Example for For Loop :
void main()
{
for (var i = 1; i <= 10; i++)
{
if (i%2 == 0) print(i);
}
}
The code will output all the even numbers(divisible by 2) from 1-10.
The output will be of the code will be
3
4
5
2
4
6
8
10
Here , the loop generates numbers from 6 to 1 and calulates the product in every iteartion and outputs the factorial of the number entered.
While Loop
While Loop is a type of indefinite(Loops in which number of iterations are not constant) Loop which checks the test expression or based on a boolean condition(True or False) . That means if the test expression is true it will execute the statement block and it will evaluate again and again as long as the test expression is true. When the test expression is false it will exit from the loop.
Syntax for While Loop
while(condition)
{
// Statement block
}
Example for While Loop
void main()
{
var i = 1;
while (i<=5)
{
print(i);
i++;
}
}
The Code will output numbers from 1 to 5 because the condition (i<=5) is true.
Output of the code is
1
2
3
4
5
Do-While Loop
Do-While like While Loop is a type of Indefinite Loop(Number of iterations are not constant).
In Do-While Loop is similar to While Loop the difference is Do-While loop executes the statement block once even if the test expression or condition is false unlike While Loop which does not execute statement block if the test expression is false
Syntax for Do-While Loop
do
{
// statement block
}
while (condition);
Example for Do-While Loop
void main()
{
var i = 1;
do
{
print(i);
i++;
}
while (i<=5);
}
Output of the Code is
1
2
3
4
5
2. Selection Statements/Condtional Statements
Conditional Statements are Decision making Statements which evaluate a single or multiple test conditions which result in Boolean Value(True or False). The result of the test condition determines which statement block to be executed.
There are mainly four Conditional Statements in Dart
- If Statement
- If else Statement
- If else if Statement
- Switch Case Statement
If Statement
If Statement executes the statement block if and only if the particular test condition is true. If the test condition is False then the statement block is skipped.
Syntax for If Statement in Dart
if(test condition)
{
// Statement Block
}
Example for If Statement
void main()
{
int number = 15 ;
if(number>10)
{
print("15 is greater than 10");
}
}
The Output of the program will be "15 is greater than 10" , Because 15 is greater than 10
If the condition was (number>20) then the result would be False and the statement block would be skipped.
If else Statement
If Else statement is similar to If Statement only additional part is when the Test Condition is evaluated as False then it executes another statement block unlike If Statement where the statement block will be skipped if the test condition is evaluated as False.
Syntax for If else Statement in Dart
if(test condition)
{
//Statement Block 1
}
else
{
// Statement Block 2
}
Example for If else Statement in Dart
void main()
{
int x = 15 ;
int y = 30 ;
if(x>y)
{
print("x is greater than y");
}
else
{
print("y is greater than x");
}
}
The output of the program will be:
"y is greater than x" because y(30) is greater than x(15). Here the test condition was exaluated as False but as we have used If else statement it moved to Statement block 2 and executed that Statement.
If else if Statement
- If else if statement includes alternative test conditions such that if the first test condition is True then it will execute the if statement and skip all the rest test conditions.
- If it is evaluated as False then it will skip the if condition and move to alternative if else test condition and if it is evaluated as True it will execute that particular statement block.
- And if the test condition is evaluated as False then it will move to next alternate if else condtion and this cycle goes on. If none of the test condition is true then It will skip all the if else statements and move to else statement and execute that statement block.
- An If else if condition can have 'n' number of if else statements
Syntax for If else if Statement in DART
if(test condition1)
{
// statement block 1
}
else if(test condition2)
{
// statement block 2
}
.
.
else if(test condition N)
{
// statement block N
}
else
{
statement block x
}
Example for If else if Statement
void main()
{
var x = -4;
if(x > 0)
{
print("x is a positive integer ");
}
else if(x==0)
{
print("x is equal to zero ");
}
else
{
print("x is a negative integer");
}
}
The output of the code will be:
"x is a negative integer" because the if condition and if else condition were evaluated as false So the program executed the else statement.
Switch Case Statement
Switch Case Statement is used when we have to evaluate a test condition against multiple cases. The statement block of the particular case which is evaluated as true is executed. If all the case statements are evaluated as False then the statement block of the default case will be executed.
Syntax of Switch Case Statement
switch(test condition)
{
case value1:
{
// statement block
}
break;
case value2:
{
// statement block
}
break;
default:
{
// statement block
}
break;
}
Example for Switch Case Statement
void main()
{
var number = 3;
switch(number){
case 1:
{
print("the number is 1.");
}
break;
case 2:
{
print("the number is 2.");
}
break;
case 3:
{
print("the number is 3.");
}
default :
{
print("The number is other than 3");
}
}
The output of the program will be -
"The number is 3" because the case value of the number is equal to 3 , which results in True and statement block of that particular case statement was executed.
3. Jump Statements
Jump Statements as the name suggests are used to transfer the control to other statements in the program when used.
Jump Statements in Dart are of mainly two types:
- Break
- Continue
Break Statement
Break statement is mainly used to termimate the execution of that particular statement and transfers the control to the following statement. It is mainly used in Switch case , Looping Statements and If else statement.
Synatx for Break Statement in Dart:
break ;
Continue Statement
Continue statement is used mainly in Looping Statements and Conditional Statements. It used when a particular iteration has to be skipped in a loop. When continue statement is used the particular iteration is skipped and the control is transferred to the following statement. It does not terminate or exit from the loop unlike break statement which does when used.
Syntax for Continue Statement:
continue ;
With this article at OpenGenus, you will have a complete idea of using different control flow statements in Dart. Enjoy.