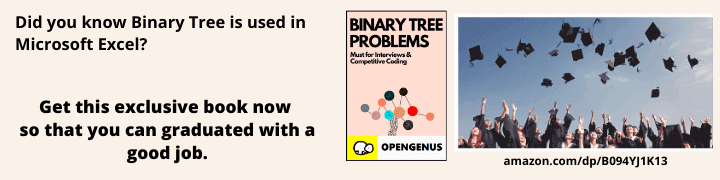
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In C++ language, the assignment operator '=' is used to assign a value to a variable. Some main points regarding assignment operators are:
- Assignment operator is a binary operator.
- Assignment operator has lower precedence than all other operators except comma operator.
- The left hand side operand of the assignment operator is a variable and the right hand side operand is the value.
int opengenus_a =5; // opengenus_a is a variable of integer type
// and 5 is the assigned value
float opengenus_b=6; // opengenus_b is a variable of float type
// and 6 is the assigned value
Different uses of assignment operator
As we know that we can use assignment operator to assign a value to the variable.But we can use an assignment operator in many different ways.
- += : It is a shorthand operator which is a combination of + perator and = operator.This operator first adds the current value of the variable on the left side with the value on the right side and then assigns the value to the variable on the left.
(a=a+b) this can be reduced as (a+=b)
- -= : This operator is a combination of - and = operator.This operator first subtracts the current value of the variable on the left side with the value on the right side and then assigns the value to the variable on the left.
(a=a-b) this can be reduced as (a-=b)
=
: This operator is a combination of * and = operator.This operator first multiplies the current value of the variable on the left side with the value on the right side and then assigns the value to the variable on the left.
(a=a*b) this can be reduced as (a*=b)
- /= :This operator is a combination of / and = operator.This operator first divides the current value of the variable on the left side with the value on the right side and then assigns the value to the variable on the left.
(a=a/b) this can be reduced as (a/=b)
- %= :This operator is a combination of % and = operator.This operator first calculate the remainder of the current value of the variable on the left side with the value on the right side and then assigns the value to the variable on the left.
(a=a%b) this can be reduced as (a%=b)
Let's implement all these shorthand operators in a C++ program:
#include<iostream>
using namespace std;
int main()
{
int a=10,b;
b=a;
cout<<"value asssigned to b is: "<<b<<endl;
b+=5;
cout<<"addition: "<<b<<endl;
b-=5;
cout<<"subtraction: "<<b<<endl;
b*=5;
cout<<"multiplication: "<<b<<endl;
b/=5;
cout<<"division: "<<b<<endl;
b%=5;
cout<<"remainder: "<<b<<endl;
return 0;
}
Output:
value assigned to b is: 10
addition: 15
subtration: 10
multiplication: 50
division: 10
remainder:0
This is the code in which we've implemented all the types of assignment operator.
Comparision operator '=='
- We have an operator known as comparision operator ==.This operator should not be taken as an assignment operator. It has a whole different task to perform in programming.
- Comparision operator also known as equality operator, checks whether the value of two operands are equal or not.
operand1 == operand2
A code to understand the working of comparision operator
#include<iostream>
using namespace std;
int main()
{
int Open=12,Genus=12;
if(Open==Genus) //Comparing the values of two operands
{
cout<<"values are equal";
}
else
{
cout<<"values are not equal";
}
return 0;
}
Output:
Values are equal
- This shows that the working of assignment operator '=' is different from comparision operator '=='.
- Assignment operator is used to assign a value to a variable and comparision operator is used to compare the values of two different variables.
Learn more:
- Fundamental Data Types in C++ by Siddharth Gusain
- Variadic function in C/ C++ by Khitish Panigrahi
- Understand use of Pointers in C and C++ by Ishneet Kaur Ahuja
- Different ways to take input in C++ by Vincent Iroleh
- Auto keyword in C++ by Harshita Sahai
- List of C++ topics at OpenGenus
- List of C topics at OpenGenus