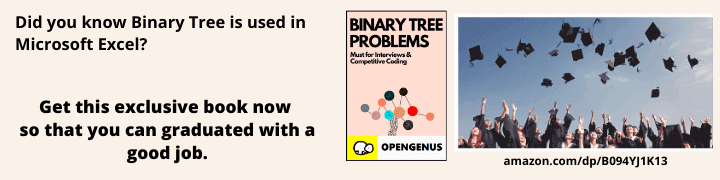
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes
Up till C++11 the programmer needed to mention the data type of the variable being used in the code but now we can use the keyword auto and decltype the type will be idetified by the compiler itself.
This property is called type inference and helps in saving a lot of time, doing this will only lead to an increase in compilation time but there will be no reduction in the rum time.
The auto keyword specifies the type of the variable that is being declared will be automatically identified by the compiler.
NOTE : If a function will return an auto type then it will evaluted during run time.
Example 1: Using auto for type inference
// C++ program to demonstrate working of auto
// and type inference
#include <bits/stdc++.h>
using namespace std;
int main()
{
auto x = 20;
auto y = 98.77;
cout << typeid(x).name() << endl
<< typeid(y).name() << endl;
return 0;
}
Output:
i
d
NOTE : The typeid operator allows the type of an object to be determined at run time.
In this example we try to explain the main idea behind auto being introduced in C++14 i.e not defining the type of variable being used. The output consists of the type of the value assigned to the auto variables.
i : integer
d : decimal
Example 2: Using auto to save time while creating iterators
// C++ program to demonstrate that we can use auto to
// save time when creating iterators
#include <bits/stdc++.h>
using namespace std;
int main()
{
// Create a set of strings
set<string> st;
st.insert({ "Welcom", "to", "OpenGenus", "IQ" });
// 'it' evaluates to iterator to set of string
// type automatically
for (auto it = st.begin(); it != st.end(); it++)
cout << *it << " ";
return 0;
}
Output:
Welcome to OpenGenus IQ
A smart way of using auto is when you have long declerations and can reduce the time as diplayed above.
As explained above we use the auto type intference for long decelarations i.e Welcom to OpenGenus IQ and this our output as well.
We display our result using for loop which can be easily understood from the example.
Example 3: method with return type as auto
#include<iostream>
using namespace std;
auto multiply (int x, int y)
{
auto z = x*y;
return z;
}
int main()
{
auto x = 5;
auto y = 3;
auto a = multiply(x,y);
cout<<a;
return 0 ;
}
Output:
15
In order to expalin our statement mentioned in the introduction (NOTE : If a function will return an auto type then it will evaluted during run time) we try to explain the concept of defining the return type as auto and get the desired output.
Difference between template and auto
Auto keyword different from template only when you need to make a generic class.
Generics is the way to allow type (Integer, String, … etc and user-defined types) to be a parameter to methods, classes and interfaces.
auto
class A { auto a; auto b; }
When you create an object of the above class it will give you an error.
B b; // Give you an error because compiler cannot decide type so it can not be assigned default value to properties
Whereas using template you can make a generic class like this:
template
template <class T>
class A
{
T a;
};
int main()
{
A<int> A1; //No Error
return 0;
}
Difference between auto and decltype
-
auto is limited to defining the type of a variable for which there is an initializer while decltype is a broader construct that, at the cost of extra information, will infer the type of an expression.
-
decltype gives the declared type of the expression that is passed to it while auto does the same thing as template type deduction. So, for example, if you have a function that returns a reference, auto will still be a value (you need auto& to get a reference), but decltype will be exactly the type of the return value.