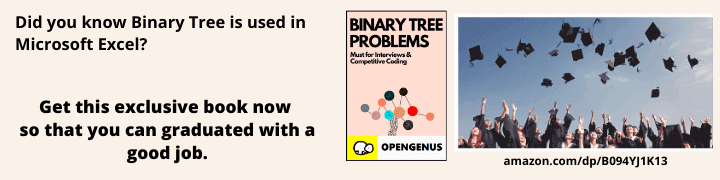
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes
Up till C++11 the programmer needed to mention the data type of the variable being used in the code but now we can use the keyword decltype and auto the type will be idetified by the compiler itself.
This property is called type inference and helps in saving a lot of time, doing this will only lead to an increase in compilation time but there will be no reduction in the rum time.
NOTE : decltype type specifier, together with the auto keyword, is useful primarily to developers who write template libraries.
Decltype stands for declared type of an entity or the type of an expression. It lets you extract the type from the variable so decltype is sort of an operator that evaluates the type of passed expression.
SYNTAX :
decltype( expression )
Example 1: demonstrate use of decltype
// C++ program to demonstrate use of decltype
#include <bits/stdc++.h>
using namespace std;
int function1() { return 24; }
char function2() { return 'o'; }
int main()
{
// Data type of x is same as return type of fun1()
// and type of y is same as return type of fun2()
decltype(function1()) x;
decltype(function2()) y;
cout << typeid(x).name() << endl;
cout << typeid(y).name() << endl;
return 0;
}
Output:
i
c
NOTE : The typeid operator allows the type of an object to be determined at run time.
The above code is the most simplest way of expressing decltype where with the help of keyword decltype and typeid we our able to identify the type of our expression and display it's type respectively.
Example 2: Another way of using decltype
// Another C++ program to demonstrate use of decltype
#include <bits/stdc++.h>
using namespace std;
int main()
{
int x = 5;
// j will be of type int : data type of x
decltype(x) j = x + 20;
cout << typeid(j).name();
return 0;
}
Output:
i
The above code helps us expressing decltype where with the help of keyword decltype and typeid we our able to identify the type of our expression and display it's type respectively.
Example 3
//auto and decltype
#include<iostream>
#include<vector>
using namespace std;
int main()
{
// Using auto for type deduction
vector<int> arr(5);
for(auto it = arr.begin(); it != arr.end(); it ++)
{
cin >> *it;
}
// Using decltype for type deduction
vector<int> arr(10);
for (decltype(arr.begin()) it = arr.begin(); it != arr.end(); it++)
{
cin >> *it;
}
return 0;
}
In the above example we tend to explain both keywords auto and decltype as when use together can do wonders for your code. In this code we make our user needs in mind and make an array of space 5 but didn't specified the data type to be used while assiging the value and gives the used freedom to know the data type of values in array as well type of expression.
Difference between decltype and auto
-
decltype gives the declared type of the expression that is passed to it while auto does the same thing as template type deduction. So, for example, if you have a function that returns a reference, auto will still be a value (you need auto& to get a reference), but decltype will be exactly the type of the return value.
-
autois limited to defining the type of a variable for which there is an initializer while decltype is a broader construct that, at the cost of extra information, will infer the type of an expression.