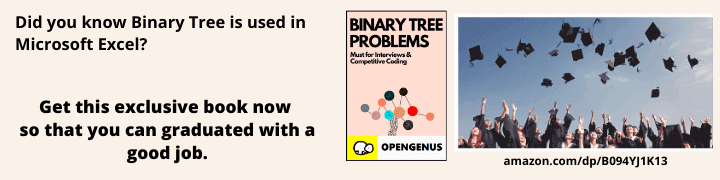
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes
Yes you read it right it's not function but functors it stands for function objects.
In other words, a functor is any object that can be used with () (opertor overloading) in the manner of a function.
Function objects are another example of the power of generic programming and the concept of pure abstraction. We could say that anything that behaves like a function is a function. So, if we define an object that behaves as a function, it can be used as a function as well.
It allows an object to behave as a function. Objects have state that is they can store data but functions cannot store anything as it is a property. Functions are do the pre-defined computations provided the input data but cannot get any data from its previous use.
To bring the best of both objects and functions, we can functors which are function objects or functions that have a state that is it can store a value.
For example:
function f1(d1): uses data d1 and output of its previous use (state)
This is possible in functors.
Syntax
Functors are defined by defining any particular function in a particular format such that there are two cases:
- one to handle if there are no previous state
- other to handle input data and previous state
class obj
{
<data type> data;
public:
// handle to previous state
obj(){
}
// new_data is the current input and data is the initial state
obj(<data type> new_data): data {new_data}
{
// do something with new_data and data
}
};
Example
#include<iostream>
using namespace std;
class mul
{
int _val;
public:
mul(){
}
mul(int val):_val{val}{
return val*_val;
}
};
int main()
{
mul mul12(12);
cout<<mul12(3)<<"\n";
cout<<mul12(2);
return 0;
}
Output:
36
24
In the above example we do the following steps :
- We created an object of name mul12 and pass 12 as a paramter.
- After creating the object we use mul12 as a function and pass 3 and 2 as parameter respectively.
mul(int val):_val{val}
{
return val*_val;
}
- We print the result.
Advantages of functors :
- Function object are "smart functions." :
Objects that behave like pointers are smart pointers. This is similarly true for objects that behave like functions: They can be "smart functions" because they may have abilities beyond operator (). Function objects may have other member functions and attributes. This means that function objects have a state.
- Each function object has its own type :
Ordinary functions have different types only when their signatures differ. However, function objects can have different types when their signatures are the same. In fact, each functional behavior defined by a function object has its own type. This is a significant improvement for generic programming using templates because you can pass functional behavior as a template parameter.
- Function objects are usually faster than ordinary functions :
The concept of templates usually allows better optimization because more details are defined at compile time. Thus, passing function objects instead of ordinary functions often results in better performance.
Use of functors
Functors are used in systems where it is necessary to store previous information like:
-
database systems: it is useful to store number of active connections at the time
-
load balancing in a system through operating system: it is useful to know number of active processes at the time