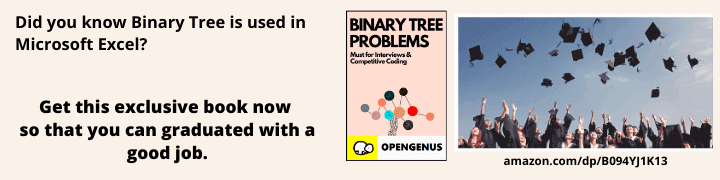
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 40 minutes
The HTML <audio> element is used to embed sound or music element in our HTML page. It may contain one or more audio sources, represented using the src attribute or the <source> element: the browser will choose the most suitable one. We will discuss about in in later part of the article. It can also be the destination for streamed media, using a MediaStream
.
Example
<audio controls src="music.mp3">
Your browser does not support the audio element.
</audio>
Preview:
The above example shows simple usage of the <audio>
tag. Similar to <img>
or <video>
tags, we include a path to the media we want to embed inside the src attribute. We can include other attributes to specify information such as, whether we want it to autoplay and loop, whether we want to show the browser's default audio controls, etc.
The content inside the opening and closing <audio></audio>
tags is shown as a fallback in browsers that don't support the element.
⭐ Important: Browsers don't all support the same audio formats. Wou can provide multiple sources inside nested <source>
tags, and the browser will then use the first one it understands. This can be explained from the example listed below:
<audio controls>
<source src="myAudio.mp3" type="audio/mpeg">
<source src="myAudio.ogg" type="audio/ogg">
Your browser doesn't support HTML5 audio.
</audio>
Some Important Points
- If we don't specify the controls attribute, the audio player won't include the browser's default controls. We can create our own custom controls using JavaScript and the HTMLMediaElement API.
- We can get precise control over our audio using HTMLMediaElement methods.
<audio>
tag can't have subtitles/captions associated with it.- We can also use the Web Audio API to directly generate and manipulate audio streams from JavaScript code.
Some Attributes of <audio> tag
- autoplay - Specifies that the audio will start playing as soon as it is ready. It's value is "autoplay".
- controls - Specifies that audio controls should be displayed (such as a play/pause button etc). It's value is "controls."
- loop - Specifies that the audio will start over again, every time it is finished. It's value is "loop".
- muted - Specifies that the audio output should be muted.
- preload - Specifies if and how the author thinks the audio should be loaded when the page loads. It's value can be auto, metadata or none.
- src - Specifies the URL of the audio file.
HTML5 Audio Methods
The play() Method
The play() method starts playing the current audio or video.
Example:
var music = document.getElementById("myAudio");
function playAud() {
music.play();
}
Syntax | audio|video.play() |
Parameters | None |
Return Value | None |
The pause() Method
The pause() method halts (pauses) the currently playing audio or video.
Example:
var music = document.getElementById("myAudio");
function pauseAud() {
music.pause();
}
Syntax | audio|video.pause() |
Parameters | None |
Return Value | None |
The load() Method
The load() method re-loads the audio/video element. The load() method is used to update the audio/video element after changing the source or other settings.
Example:
document.getElementById("mp3_src").src = "song.mp3";
document.getElementById("mus_src").src = "music.mp3";
document.getElementById("myAudio").load();
Syntax | audio|video.load() |
Parameters | None |
Return Value | None |
The canPlayType() Method
The canPlayType() method checks if the browser can play the specified audio/video type.
Example:
var obj = document.createElement('video');
console.log(obj.canPlayType('video/mp4'));
Syntax | audio|video.canPlayType(type) |
Parameters - type | Specifies the audio/video type (and optional codecs) to test support for. Common values:
Common values, including codecs:
Note: This method can only return "probably" if codecs are included. |
Return Value | A String, representing the level of support. Possible return values:
|