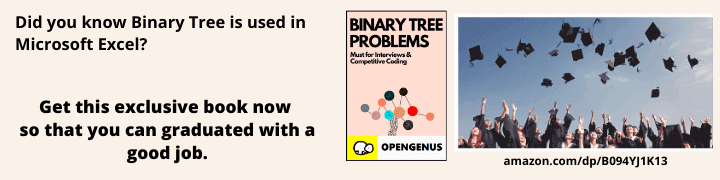
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 40 minutes
The HTML Video tag <video> embeds a media player which supports video playback into the document. We can use <video> for audio content as well, but the <audio> tag may provide a more appropriate user experience.
Example
<video controls width="250" controls>
<source src="video.webm" type="video/webm">
<source src="video.mp4" type="video/mp4">
Sorry, your browser doesn't support embedded videos.
</video>
Preview:
The above example shows simple usage of the <video> element. In a similar manner to the <img> element, we include a path to the media we want to display inside the src attribute. we can include other attributes to specify information such as video width and height, whether we want it to autoplay and loop, whether we want to show the browser's default video controls, etc.
The content inside the opening and closing <video></video> tags is shown as a fallback in browsers that don't support the element.
⭐ Important: In the above example, we have used two <source> tags, because all browsers don't support the same video format. Thus, we can provide multiple sources inside nested <source> elements, and the browser will then use the first one it understands.
Some attributes of <video> tag
autoplay
This attribute requires a boolean attribute (true or false). If specified, the video automatically begins to play back as soon as it can do without stopping to finish loading the data.
buffered
We can use this attribute to determine the time ranges of the buffered media. This attribute contains a TimeRanges object.
controls
If this attribute is present, the browser will offer controls to allow the user to control video playback, including volume, seeking, and pause/resume playback.
height
The height of the video's display area, in pixels.
width
The width of the video's display area, in pixels.
loop
This attribute accepts a boolean attribute; if specified, the browser will automatically seek back to the start upon reaching the end of the video.
muted
If the attribute is set to true, the audio will be initially silenced. Its default value is false, meaning that the audio will be played when the video is played.
preload
This attribute is intended to provide a hint to the browser about what the author thinks leading to the best user experience with regards to what content is loaded before the video is played. The accepted values are none, metadata, auto, empty string.
playinline
This attribute accepts a boolean attribute which indicates that the video is to be played "inline", that is within the element's playback area.
poster
This attribute accepts the URL for an image to be shown while the video is downloading. If this attribute isn't specified, nothing is displayed until the first frame is available, then the first frame is shown as the poster frame.
src
This attribute accepts the URL of the video to embed. This is optional. We may instead use the <source> element within the video block to specify the video to embed.
The <source> tag
The <source> tag specifies multiple media resources for <video> tag. It is an empty tag, meaning that it has no closing tag. It is commonly used to serve the same media content in multiple formats supported by different browsers.
Example:
<video controls width="250" height="200" muted>
<source src="video.webm" type="video/webm">
<source src="video.mp4" type="video/mp4">
This browser does not support the HTML5 video element.
</video>
Preview:
Some attributes of <source> tag:
src
Required for <audio> and <video>, address of the media resource.
type
The MIME-type of the resource, optionally with a codecs parameter.
The <track> tag
The <track> tag is used as a child of the media elements <audio> and <video>. It lets us specify timed text tracks (or time-based data), for example to automatically handle subtitles. The tracks are formatted in WebVTT format (.vtt files).
Example:
<video controls width="250">
<source src="video.mp4" type="video/mp4">
<track default kind="captions" srclang="en" src="video.vtt"/>
Sorry, your browser doesn't support embedded videos.
</video>
Preview:
⭐ Important:It doesn't work locally, we need to run it on a server.
Some attributes of <track> tag:
default
This attribute indicates that the track should be enabled unless the user's preferences indicate that another track is more appropriate. This attribute can only be used once per media element.
kind
This attribute indicates, how the text track is meant to be used. If omitted the default kind is subtitles. The keywords that can be used in this attribute are: subtitles, captions, descriptions, chapters and metadata.
src
This attribute specifies the address of the track (.vtt file). it must be a valid URL.
srclang
This attribute specifies the language of the track text data. If the kind attribute is set to subtitles, then srclang must be defined.
label
This must be a user-readable title of the text track which is used by the browser when listing available text tracks.
HTML5 Audio/Video Methods
The play() Method: to play the video
The play() method starts playing the current audio or video.
Example:
var vid = document.getElementById("myVideo");
function playVid() {
vid.play();
}
Syntax | audio|video.play() |
Parameters | None |
Return Value | None |
The pause() Method: to pause a video
The pause() method halts (pauses) the currently playing audio or video.
Example:
var vid = document.getElementById("myVideo");
function pauseVid() {
vid.pause();
}
Syntax | audio|video.pause() |
Parameters | None |
Return Value | None |
The load() Method: to reload the video
The load() method re-loads the audio/video element. The load() method is used to update the audio/video element after changing the source or other settings.
Example:
document.getElementById("mp4_src").src = "movie.mp4";
document.getElementById("webm_src").src = "movie.webm";
document.getElementById("myVideo").load();
Syntax | audio|video.load() |
Parameters | None |
Return Value | None |
The canPlayType() Method: checks support
The canPlayType() method checks if the browser can play the specified audio/video type.
Example:
var vid = document.createElement('video');
isSupp = vid.canPlayType(vidType+';codecs="'+codType+'"');
Syntax | audio|video.canPlayType(type) |
Parameters - type | Specifies the audio/video type (and optional codecs) to test support for. Common values:
Common values, including codecs:
Note: This method can only return "probably" if codecs are included. |
Return Value | A String, representing the level of support. Possible return values:
|
The addTextTrack() Method
The addTextTrack() method creates and returns a new TextTrack object.The new TextTrack object is added to the list of text tracks for the audio/video element.
Example:
var text1 = myVid.addTextTrack("caption");
text1.addCue(new TextTrackCue("Test text", 01.000, 04.000, "", "", "", true));
Syntax:
audio|video.addTextTrack(kind,label,language)
Parameter Values:
Value | Description |
kind | Specifies the kind of text track. Possible values:
|
label | A string specifying the label for the text track.Is used to identify the text track for the users. |
language | A two-letter language code that specifies the language of the text track. |
Return Value:
A TextTrack Object, which represents the new text track