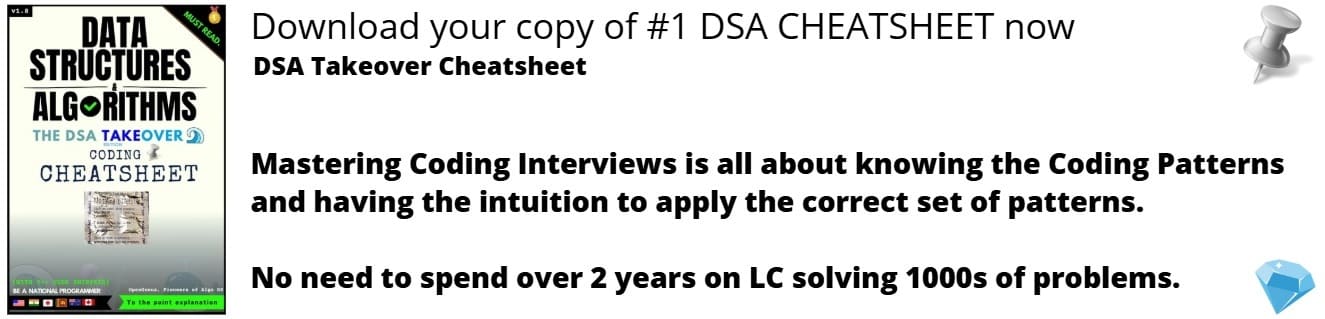
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 35 minutes
One of the most important types of information is the one entered by the user, a lot of times we have to allow the user to enter information to the program and we can make use of that information to perform certain functions.
In this article, we're going to discuss how we can get different types of information from the user.
Whenever we're getting information from the user, the first thing we want to do is store that information somewhere (in a variable). If we don't store the information entered by the user somewhere, it's absolutely useless, because we can't make use of it.
So the first thing we have to do is create a variable to store the user's information. In this article, we're going to write a program that asks the user to enter their age.
#include <iostream>
using namespace std;
int main() {
int age;
return 0;
}
We declared a variable age
of data type integer. Telling C++ that we want the user's information to be of the data type of integer
. We didn't give it a value, because we expect to get the value from the user.
The next thing we are going to do is to prompt the user to enter something. If we don't ask the user to enter something, they're not going to know what type of information we want them to enter. We are just going to use the cout
object here.
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: \n";
return 0;
}
Once we have declared a variable, and prompt the user to enter some information, the next thing is to make use of those values the user entered. The way we get information is actually the opposite of how to give out information.
Instead of object, here we're going to make use of the cout
cin
object and >>
two greater than
sign.
Note: When we use the
cout
object, we make use of the<<
twoless than
sign. very important to remember.
After the greater than sign
, we're going to enter the name of the variable age
where we want to store the value entered by the user. Finally, we're going to print out the information entered by the user.
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: \n";
cin >> age;
cout << "You are " << age << " years old\n";
return 0;
}
Basically, all we did above was to declare a variable age of data type integer
and we prompt the user to enter some information, then we stored the information to the variable age and printed it out.
We can also change the data type of our variable to any other data type we are expecting to get from the user like char
, double
, etc. But if we want to get a data type of string from the user, we have to use something different than just changing variable type to string age
and the cin
. Let's change our program to prompt the user to enter their names instead of age.
Then we're going to use cin
along with the getline
command to get every information entered by the user. The getline
helps us to get everything in the line entered by the user.
#include <iostream>
using namespace std;
int main() {
string name;
cout << "Enter your name: \n";
getline(cin, name);
cout << "Hello " << name;
return 0;
}
We can get as many information as we want from the user, let's make our program smarter by prompting the user of their name and age.
#include <iostream>
using namespace std;
int main() {
string name;
int age;
cout << "Enter your name: \n";
getline(cin, name);
cout << "Enter your age: \n";
cin >> age;
cout << "Hello " << name << ", you are " << age << " years old.\n";
return 0;
}
How to take input from a file?
Before we discuss how to take input from a file, lets take a look at the standard C++ library called fstream, which defines three new data types −
-
ofstream
: This data type represents the output file stream and is used to create files and to write information to files. -
ifstream
: This data type represents the input file stream and is used to read information from files. -
fstream
: This data type represents the file stream generally, and has the capabilities of both ofstream and ifstream which means it can create files, write information to files, and read information from files.
How to take input from a file?
Before we discuss how to take input from a file, let's take a look at the standard C++ library called fstream, which defines three new data types −
-
ofstream
: This data type represents the output file stream and is used to create files and to write information to files. -
ifstream
: This data type represents the input file stream and is used to read information from files. -
fstream
: This data type represents the file stream generally, and has the capabilities of both ofstream and ifstream which means it can create files, write information to files, and read information from files.
To perform file processing, the header files <iostream>
and <fstream>
must be included in our program.
#include <iostream>
#include <fstream>
using namespace std;
int main() {
return 0;
}
Our purpose is to take input from a file. But we can't necessarily do that if there is no file and some information in it.
So, let's learn how we can create, write, and read through a file using our newfound powers.
#include <iostream>
#include <fstream>
using namespace std;
int main() {
string name;
ofstream myfile;
myfile.open ("example.txt");
cout << "Enter your name: \n";
getline(cin, name);
myfile << name;
myfile.close();
return 0;
}
The code above creates a file example.txt
, prompts the user to enter some their name and writes it into the file. Then we closed our file.
Just like when we use our cout
object, but here we're using the file stream myfile
instead.
We can also store multiply data inside our file.
#include <iostream>
#include <fstream>
using namespace std;
int main() {
string name;
int age;
ofstream myfile;
myfile.open ("example.txt");
cout << "Enter your name: \n";
getline(cin, name);
cout << "Enter your age: \n";
cin >> age;
myfile << name << "\n";
myfile << age << "\n";
myfile.close();
return 0;
}
Our example.txt
file should now have our name and age in different lines like this:
Okay, we have been able to create and write to the file, let's learn how we can get information that we have written into our file.
To achieve this we will use the ifstream
#include <iostream>
#include <fstream>
using namespace std;
int main() {
string data; // to hold our files information per line
ifstream myfile ("example.txt");
if (myfile.is_open()) {
while (getline(myfile, data)) {
cout << data << endl;
}
myfile.close();
}
else cout << "Unable to open file";
return 0;
}
In this last example, we read our text file and prints out its content on the screen. We have created a while loop that reads the file line by line, using getline. The value returned by getline is a reference to the stream object itself, which when evaluated as a boolean expression (as in this while-loop) is true if the stream is ready for more operations, and false if either the end of the file has been reached or if some other error occurred.