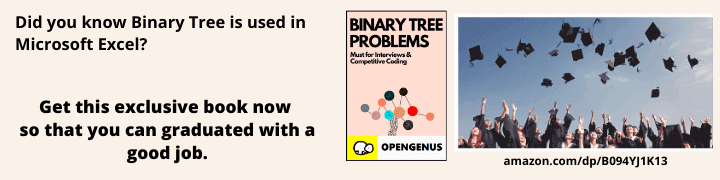
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn about different ways to convert an array to vector in C++.
An Array is the collection of elements of the same data type and are stored in contiguous memory location. We can not change it's size once we have initialised it.
A Vector is also a collection of elements of the same data type but they are dynamic array i.e. we can change it's size as and when required.
There are different ways to convert array to vector in C++. Some of them are mentioned below:
- Using a loop and push_back() function
- Using Range-based Constructor
- Using C++ STL (Standard Template Library):
- copy() function
- insert() function
- assign() function
1. Using a loop and push_back() function:
We will create an array of size n and an empty vector. We will run a for loop till n and use push_back() function to insert element into the vector.
#include <iostream>
#include <vector>
using namespace std;
int main()
{
int arr[] = { 1, 3, 5, 7, 9, 11 };
int n = sizeof(arr) / sizeof(arr[0]);
vector <int> vec;
for (int i = 0; i < n; i++) {
vec.push_back(arr[i]);
}
for (int i = 0; i < n; i++) {
cout << vec[i] << " ";
}
return 0;
}
Output:
1 3 5 7 9 11
2. Using Range-based Constructor:
We will pass two iterators to the vector constructor to convert an array to a vector, the first one pointing to the beginning of the array, and the second pointing to the end of the array.
#include <iostream>
#include <vector>
using namespace std;
int main()
{
int arr[] = { 1, 3, 5, 7, 9, 11 };
int n = sizeof(arr) / sizeof(arr[0]);
vector<int> vec(arr, arr + n);
for (int i = 0; i < n; i++) {
cout << vec[i] << " ";
}
return 0;
}
Output:
1 3 5 7 9 11
3. Using C++ STL(Standard Template Library):
-
copy(itr1_start, itr1_end, itr2_start) function:
Another way to convert an array to a vector is to use the inbuilt copy() function. This function is part of the C++ Standard Template Library (STL) and allows us to copy a range of elements from one container to another. It takes three arguments:
- an iterator to the first element of the array
- an iterator to the last element of the array
- an iterator to insert the values in the vector
#include <iostream>
#include <vector>
using namespace std;
int main()
{
int arr[] = { 1, 3, 5, 7, 9, 11 };
int n = sizeof(arr) / sizeof(arr[0]);
vector<int> vec;
copy(begin(arr), end(arr), vec.begin());
for (int i = 0; i < n; i++) {
cout << vec[i] << " ";
}
return 0;
}
Output:
1 3 5 7 9 11
-
insert(position, itr1_start, itr1_end) function:
We can use an inbuilt insert() function of C++ Standard Template Library (STL) to convert an array to a vector. It allows us to add new elements to the container at a specific position. It takes three arguments:
- an iterator pointing to the position at which the elements are to be inserted.
- an iterator to the first element of the array
- an iterator to the last element of the array
#include <iostream>
#include <vector>
using namespace std;
int main()
{
int arr[] = { 1, 3, 5, 7, 9, 11 };
int n = sizeof(arr) / sizeof(arr[0]);
vector<int> vec;
vec.insert(vec.begin(), arr, arr + n);
for (int i = 0; i < n; i++) {
cout << vec[i] << " ";
}
return 0;
}
Output:
1 3 5 7 9 11
-
assign(itr_start, itr_end) function:
We can also use assign() function to assign values from an array to an empty or already existing vector. It takes the beginning and end iterators of the array as arguments.
#include <iostream>
#include <vector>
using namespace std;
int main()
{
int arr[] = { 1, 3, 5, 7, 9, 11 };
int n = sizeof(arr) / sizeof(arr[0]);
vector<int> vec;
vec.assign(arr, arr + n);
for (int i = 0; i < n; i++) {
cout << vec[i] << " ";
}
return 0;
}
Output:
1 3 5 7 9 11
With this article at OpenGenus, you must have the complete idea of how to convert an array to vector in C++.