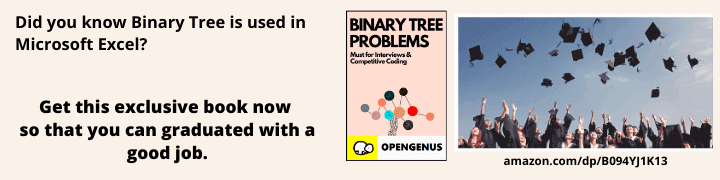
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have designed and implemented a C Program to convert number to binary using bitwise operators.
Table of contents:
- Problem Statement
- Approach 1
- Alternative Approach
- C Program to convert number to binary using bitwise operators
- C Program with saving converted Binary format
Learn:
Problem Statement
In this problem, we have to design an approach to convert any number in decimal format to binary format using bitwise operations only.
The usual approach is to use division operations with 10 or 2. The challenge is to use bitwise operations only.
Implement the solution in C programming language.
Approach 1
Let us assume we are dealing with 16 bit numbers.
To check if the most significant bit is set to 1, we can do AND bitwise operation with the most significant bit set and all other bits set to 0 (that is )
Also, 0b1000000000000000
is equal to 0x8000 in hexadecimal format.
if(N & 0x8000)
// Most significant bit is 1
else
// Most significant bit is 0
The key idea is to is do AND with the original number and another number with i-th bit set to 1 and based on the result (zero or non-zero), we determine if the resultant number in binary format.
- 0x8000 = 16-th bit set to 1 only
- 0x8000 >> 1 = 15-th bit set to 1 only
- 0x8000 >> 1 >> 1 OR 0x8000 >> 2 = 14-th bit set to 1 only
- and so on.
Alternative Approach
An alternative approach is to start checking from the lowest significant bit to most significant bit.
We will start by checking the lowest significant bit:
if(N & 1)
// Most significant bit is 1
else
// Most significant bit is 0
Incrementally, we need to check bit with higher significance:
- 1 = 1st bit set to 1 only
- 1 >> 1 = 2-nd bit set to 1 only
- 1 >> 1 >> 1 OR 1 >> 2 = 3-rd bit set to 1 only
- and so on.
C Program to convert number to binary using bitwise operators
Following is the complete C Program to convert number to binary using bitwise operators:
// Part of iq.opengenus.org
#include<stdio.h>
void convertToBinary(int num)
{
int mask = 0x8000;
while (mask != 0) {
if ((num & mask) == 0)
printf("0");
else
printf("1");
mask = mask >> 1;
}
}
int main() {
int integer_number = 103;
printf("%d in binary format is: ", integer_number);
convertToBinary(integer_number);
return 0;
}
Output:
103 in binary format is: 0000000001100111
C Program with saving converted Binary format
To save the output, we define an array bin of size INT_SIZE (same as size of integer in C). The datatype of the array elements will be integer but we can use boolean as we need to store 0 or 1 only.
Following is the complete C Program to convert number to binary using bitwise operators along with saving the converted Binary number:
// Part of iq.opengenus.org
#include <stdio.h>
#define INT_SIZE sizeof(int) * 8
void convertToBinary(int *bin, int num) {
int index = INT_SIZE - 1;
while(index >= 0)
{
bin[index] = num & 1;
index--;
num >>= 1;
}
}
void printBinary(int * bin) {
printf("Converted number: ");
for(int i=0; i<INT_SIZE; i++)
{
printf("%d", bin[i]);
}
}
int main()
{
int num = 103;
int bin[INT_SIZE];
convertToBinary(bin, num);
printBinary(bin);
return 0;
}
Output:
Converted number: 00000000000000000000000001100111
With this article at OpenGenus, you must have the complete idea of how to implement a C Program to convert number to binary using bitwise operators.