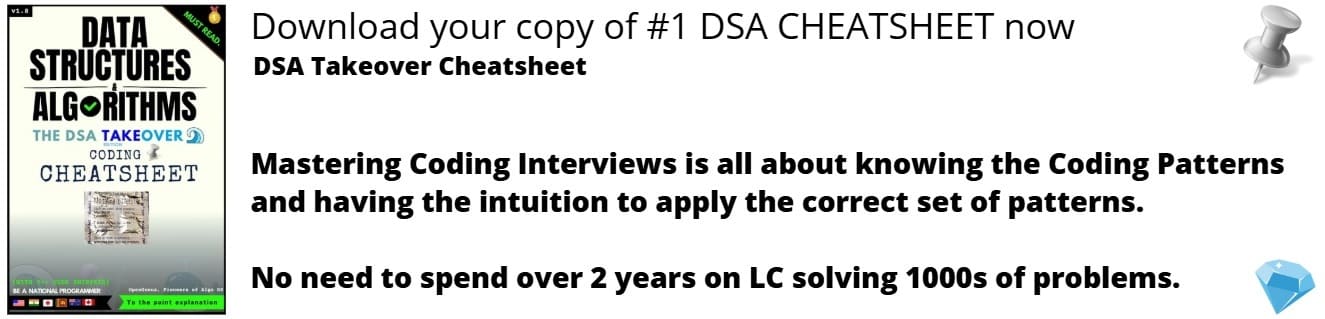
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Copying a file into another is one of the most common file tasks in any Programming Language. In this article, we have explained the approach to copy one file into another using fputc and fgetc in C program and provided a step-by-step explanation with complete C program.
To do this, we have used standard file read and write operations such as fputc and fgetc. The most common error in handling file is that file is not opened and that is handled by our C program. This is an important article that will help you revise this critical operation. This is also one of the most common Interview Questions in the field of C Programming.
Table of contents:
- Approach
- C program to copy one file into another using fputc and fgetc
Approach
The first step is to open a file and make sure it is opened correctly. In this problem, we have to open 2 files: original file and the 2nd file.
FILE *fp1;
fp1 = fopen("original.txt", "r");
if (fp1 == NULL) {
puts("cannot open this file");
exit(1);
}
Read one character from original file using fgetc and add it to the second file using fputc. Repeat this process till end of file (EOF) is reached.
do {
a = fgetc(fp1);
fputc(a, fp2);
} while (a != EOF);
The overall steps are as follows:
- Read first file "original.txt"
- Read second file "copied.txt"
- In a loop, read character from first file using fgetc and add to second file using fputc
- Continue this till end of file is reached.
C program to copy one file into another using fputc and fgetc
Following is the complete C program to copy one file into another using fputc and fgetc:
// Part of iq.opengenus.org
#include<stdio.h>
int main() {
FILE *fp1, *fp2;
char a;
fp1 = fopen("original.txt", "r");
if (fp1 == NULL) {
puts("cannot open this file");
exit(1);
}
fp2 = fopen("copied.txt", "w");
if (fp2 == NULL) {
puts("Not able to open this file");
fclose(fp1);
exit(1);
}
do {
a = fgetc(fp1);
fputc(a, fp2);
} while (a != EOF);
fcloseall();
getch();
return 0;
}
With this article at OpenGenus, you must have the complete idea of how to copy one file into another using fputc and fgetc in C programming language.