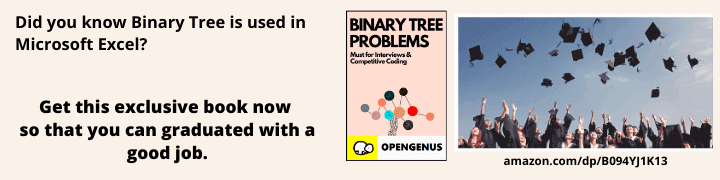
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the approach to develop a User Login and Registration System in C++ using file as a database. We have presented the approach with complete C++ code.
The code is portable to C Programming Language so we have used concepts like struct instead of class and so on.
Table of contents:
- Approach for User Registration
- C++ code for Registration
- Approach for User Login
- C++ code for User Registration and Login using file
Approach for User Registration
We create a structure named user_record to store the username and password and provide critical function like registration.
struct user_record
{
char username[50];
char password[50];
void registration(int);
} obj[5];
The idea of implementing registration function is as follows:
- Open a local file named "registration.txt" as a binary file
- Create the user record with the provided username and password
- Store the user record in the file.
- As the file is in binary, simply opening the file does not leak the username and password.
Following is the registration function:
void user_record::registration(int k)
{
int i=k;
cout<<"Enter user name= ";
cin >> username;
cout << "Enter password= ";
cin >> password;
ofstream filout;
filout.open("registration.txt", ios::app|ios::binary);
if(!filout)
{
cout<<"\nCannot open file\n";
}
else
{
cout << "\n";
filout.write((char *)&obj[i],sizeof(user_record));
filout.close();
}
cout<<"\nYou are registered.";
}
C++ code for Registration
Following is the complete C++ code for user registration using file as a database:
// Part of iq.opengenus.org
#include<iostream>
#include<fstream>
using namespace std;
struct user_record
{
char username[50];
char password[50];
void registration(int);
} obj[5];
void user_record::registration(int k)
{
int i=k;
cout<<"Enter user name= ";
cin >> username;
cout << "Enter password= ";
cin >> password;
ofstream filout;
filout.open("registration.txt", ios::app|ios::binary);
if(!filout)
{
cout<<"\nCannot open file\n";
}
else
{
cout << "\n";
filout.write((char *)&obj[i],sizeof(user_record));
filout.close();
}
cout<<"\nYou are registered.";
}
int main()
{
int t;
cout<<"\nEnter Registration Details for User 1 :: \n";
obj[0].registration(0);
cout<<"\nEnter Registration Details for User 2 :: \n";
obj[1].registration(1);
cout<<"\nEnter Registration Details for User 3 :: \n";
obj[2].registration(2);
user_record obj2;
ifstream filein;
filein.open("registration.txt",ios::in|ios::binary);
if(!filein)
{
cout<<"\nUnable to open registration database. Please try again later.\n";
}
else
{
cout<<"\nRegistered Details of All Users :: \n";
filein.read((char *)&obj2,sizeof(obj2));
while(filein)
{
cout<<"\nUsername :: " << obj2.username << "\nPassword :: " << obj2.password << "\n";
filein.read((char *)&obj2,sizeof(obj2));
}
filein.close();
}
return 0;
}
Approach for User Login
The idea of implementing user login function is as follows:
- Open the local file "registration.txt"
- Traverse through the user records in the binary file
- For each record, compare the current username and password provided during login
- If there is a match, login is successful.
Following is the sample implementation of the login function in C++:
void user_record::login(char* current_username, char* current_password)
{
int success = 0;
ifstream filein;
filein.open("registration.txt",ios::in|ios::binary);
if(!filein)
{
cout<<"\nUnable to open registration database. Please try again later.\n";
}
else
{
filein.read((char *)&obj2,sizeof(obj2));
while(filein)
{
if(current_username == obj2.username && current_password == obj2.password) {
success = 1;
break;
}
filein.read((char *)&obj2,sizeof(obj2));
}
filein.close();
}
if (success == 1)
cout << "\nLogin successful.";
else
cout << "\nLogin failed.";
}
C++ code for User Registration and Login using file
Following is the complete C++ code for login and registration using file as a database:
// Part of iq.opengenus.org
#include<iostream>
#include<fstream>
using namespace std;
struct user_record
{
char username[50];
char password[50];
void registration(int);
void login(char*, char*);
} obj[5];
void user_record::registration(int k)
{
int i=k;
cout<<"Enter user name= ";
cin >> username;
cout << "Enter password= ";
cin >> password;
ofstream filout;
filout.open("registration.txt", ios::app|ios::binary);
if(!filout)
{
cout<<"\nCannot open file\n";
}
else
{
cout << "\n";
filout.write((char *)&obj[i],sizeof(user_record));
filout.close();
}
cout<<"\nYou are registered.";
}
void user_record::login(char* current_username, char* current_password)
{
int success = 0;
ifstream filein;
filein.open("registration.txt",ios::in|ios::binary);
if(!filein)
{
cout<<"\nUnable to open registration database. Please try again later.\n";
}
else
{
filein.read((char *)&obj2,sizeof(obj2));
while(filein)
{
if(current_username == obj2.username && current_password == obj2.password) {
success = 1;
break;
}
filein.read((char *)&obj2,sizeof(obj2));
}
filein.close();
}
if (success == 1)
cout << "\nLogin successful.";
else
cout << "\nLogin failed.";
}
int main()
{
int t;
cout<<"\nEnter Registration Details for User 1 :: \n";
obj[0].registration(0);
cout<<"\nEnter Registration Details for User 2 :: \n";
obj[1].registration(1);
cout<<"\nEnter Registration Details for User 3 :: \n";
obj[2].registration(2);
user_record obj2;
ifstream filein;
filein.open("registration.txt",ios::in|ios::binary);
if(!filein)
{
cout<<"\nUnable to open registration database. Please try again later.\n";
}
else
{
cout<<"\nRegistered Details of All Users :: \n";
filein.read((char *)&obj2,sizeof(obj2));
while(filein)
{
cout<<"\nUsername :: " << obj2.username << "\nPassword :: " << obj2.password << "\n";
filein.read((char *)&obj2,sizeof(obj2));
}
filein.close();
}
return 0;
}
With this article at OpenGenus, you must have the complete idea of how to implement a C++ program to emulate user registration and login using a file as a database.