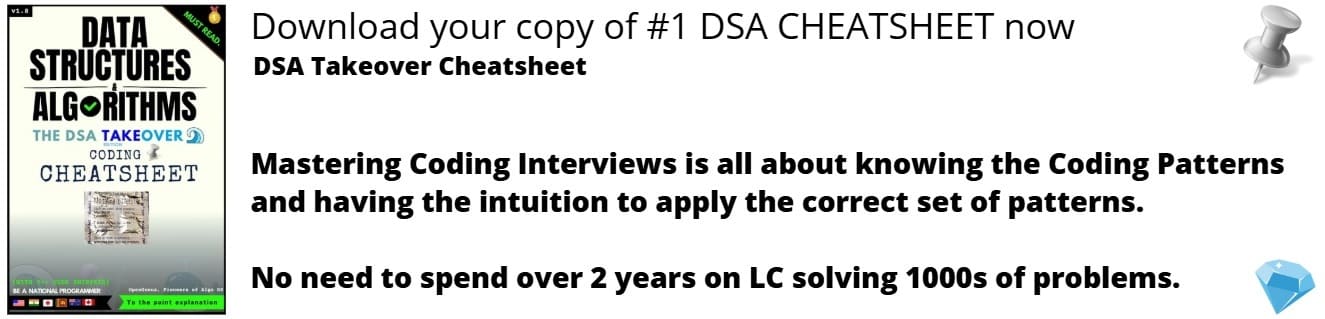
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored 4 different methods to convert vector to array in C++ Programming Language.
Table of content
- Introduction about vectors and arrays
- Different methods of converting vector to arrays
- Their implementation.
Introduction about vectors and arrays -
Arrays and Vectors both store elements at contiguous memory locations.
In c++ arrays are static and are of fixed size, i.e once we declared the size of an array in a program it is fixed and can't be changed during execution of program even if the program requires more storage and hence reduces program capability.
On the other hand vectors are dynamic arrays and are more flexible to program needs i.e. vectors can resize itself automatically according to program needs i.e they resize when insertion of element or deletion of element from vector takes place.
In both arrays and vectors elements are stored at adjacent memory locations so that they can be acccessed using iterator or pointer.
Need for conversion-
1.arrays are more efficient than vectors.
2.arrays are faster than vectors i.e vectors take more time in accessing elements as compared to array.
3.vectors occupies more memory
Different methods of converting vector to arrays(4 ways) -
- using for loop
- Using copy() function
- Using transform() function
- Using data() function
1. using for loop -
In this we using for loop traverse the whole array and copy each vector element to array.
Its implementation is given below-
#include<bits/stdc++.h>
using namespace std;
int main()
{
vector<int> v{1,4,6,7,8,9,2,3};
int n,i;
n=v.size();
int a[n];
for( i = 0; i < n; i++)
{
a[i] = v[i];
}
for(auto i: a) {
cout << i << endl ;
}
return 0;
}
Output :
1
4
6
7
8
9
2
3
Using copy() function -
copy() function of c++ can copy the elements from one object to another based on range.e.g pass the vector element to copy() function in range [start,end] and iterator to first element of array ,it will copy all elements of vector to array.
Its implementation is given below-
#include<bits/stdc++.h>
using namespace std;
int main()
{
vector<int> v{1, 3, 4, 5, 6, 7, 8, 9};
int arr[v.size()];
copy( v.begin(), v.end(), arr);
for(auto i: arr) {
cout<<i<<", ";
}
return 0;
}
Output :
1, 3, 4, 5, 6, 7, 8, 9,
Using transform() function -
transform() function belongs to c++ STL library.This function accepts an input range , an output iterator and a unary function as arguments. It basically iterates over all element applies unary function and returns output iterator.
The transform function is used in two forms -
1. Unary operation -
In this method unary operations unary_op are performed in elements in range [start,end] and stores the result in output array.It takes the pointer to start and end position of first array and start position of output array .
e.g.
int unary_op (int x) {
x = x * 2;
return x;
}
transform (arr1.start, arr1.end , output.start, unary_op);
2.Binary operation
In this method binary operation binary_op performed on elements in range [start,end] and stores the result in output array.It takes the pointer to start and end position of first array and start position of second array and output array along with a binary operation we want to apply .
e.g.
int binary_op (int i, int j) {
return i*j;
}
transform (arr1.start, arr1.end, arr2.start, output.start,binary_op );
Its implementation is given below-
#include<bits/stdc++.h>
using namespace std;
int main()
{
vector<int> v{1, 2, 3, 4, 5, 7, 8, 9};
int arr[v.size()];
transform( v.begin(), v.end(), arr, [](const auto & ele){return ele; });
for(auto i: arr) {
cout<<i<<", ";
}
return 0;
}
Output :
1, 2, 3, 4, 5, 7, 8, 9,
Using data() function -
data() function of vector in c++ returns a pointer to the internal array of vector.In this case as it returns internal array of vector so any changes in this brings changes to our original vector too.
Its implementation is given below-
#include <bits/stdc++.h>
using namespace std;
int main()
{
vector<int> v {2, 5, 7, 8, 12, 34, 56, 78};
int *arr = v.data();
int size=v.size();
for(int i = 0; i < size; i++)
{
arr[i] = arr[i]*2;
cout<<arr[i]<<" ,";
}
cout<<endl;
cout<<"modified vectors elements - \n";
for(auto i : v)
cout<<i<<" ,";
return 0;
}
Output :
4 ,10 ,14 ,16 ,24 ,68 ,112 ,156 ,
modified vectors elements -
4 ,10 ,14 ,16 ,24 ,68 ,112 ,156 ,
Above are the different methods for converting vectors to arrays in C++.