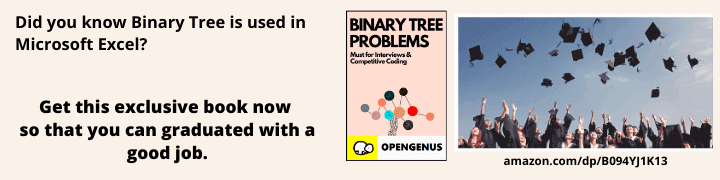
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Write a program to enter a three digit number and separately print the the digits of the number and add it and provide the implementation in C programming language.
Problem Statement
In this problem we will take an input of a three digit number number and separately print the digits and add them.
for example: 274
output
2
7
4
2+7+4=13
Solution
-
First we take an input of a three digit number from the user for example a and store it in int data type.
-
Then we will extract the digit in the hundred's place. For that we have to create a variable b and store it in int data type. Then we divide a by 100 and store it in b. Since b is a int data type only the quotient will be stored that is the third digit will be stored in b.
-
For the digit in the ten's place we will create a variable c of data type int.Then we divide a by 10. Now the digit in hundred's and ten's place in stored in c. Then we multiply b with 10 and subtract from c. We will get the ten's digit this way.
for example:
a=273
b=a/100=2
c=a/10
c=273/10=27
c=c-(bx10)=27-20=7 -
For the digit in the one's place we create another variable e and store it in int data type and then we will multiply b with 100 and c with 10 and subtract from a
For example
e=a-(bx100)-(cx10)= 273-200-70=3 -
Now we print all the digits separately and add them.
Implementation
Following is the implementation in C programming language
#include<stdio.h>
int main()
{
int a,b,c,d,e;
printf("Enter a 3 digit number=");
scanf("%d",&a);
b=a/100;
c=(a/10)-(b*10);
d=a-(b*100)-(c*10);
printf("%d\n",b);
printf("%d\n",c);
printf("%d\n",d);
e=b+c+d;
printf("%d+%d+%d=%d\n",b,c,d,e);
return 0;
}