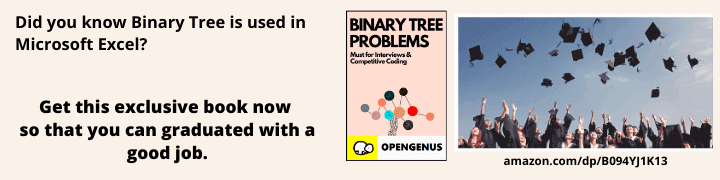
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Hello everyone, in this article at OPENGENUS, we are going to see how to add firebase authentication in iOS apps using firebase Email password authentication.
What is FireBase
Firebase is a cloud service owned by Google which allow the Web,App and also Unity game developers to add some common functionalities like Authentication, Database, Analytics to their application without any need for expensive infrastructure. FireBase provides various kinds of services:
- Authentication - Email,Google,Apple,Github,Yahoo,GameCenter etc.
- Cloud FireStore - Real time NOSQL DataBase
- MLKit - To add ML features to the apps
- Hosting - To deploy Mobile and WebApps
And much more.In this article we are going to cover email,password auth in iOS Apps.
You can download the starter project from here
Adding our app to FireBase
- Login to FireBase Console using your Gmail Id
- Create a new iOS app project
- Give your project a name
- Disable Google Analytics as it is not needed in this project
- Copy and paste the bundle ID of your app from Xcode
- Download th config.json file and add it to the Xcode project
8. Now add this following Cocoa Pod in your App to support FireBase Auth and Install it.
pod 'Firebase/Auth'
Adding new User to FireBase Auth Server
- In the starter project open the SigUpVC.swift and add the following code inside IBOutlet of SignUP button.
@IBAction func SignUpBtnPressed(_ sender: Any) {
if let email = emailtxtbox.text ,let password = passwordtxtbox.text
{
Auth.auth().createUser(withEmail: email, password: password) { (resultdata, error) in
if let err = error
{
self.showAlert(message: err.localizedDescription)
}
else
{
self.showAlert(message: "Account Created")
}
}
}
}
This code creates an new user by getting the email,password text.When the user press the signup buttton the app communicate with FireBase Auth server and register the user. And also FireBase has some constraints like the password must be 6 character long etc.So it will return the error message in the callback which we should present to the user by using an AlertViewController.Which is handled by the local method showAlert().
func showAlert(message: String)
{
let ac = UIAlertController(title: "Info", message: message, preferredStyle: .alert)
ac.addAction(UIAlertAction(title: "Ok", style: .default, handler: nil))
present(ac, animated: true, completion: nil)
}
Now run the app and press the SignUp button to open SignUpVC.
- Enter any email and password and press signup
And also you can see that when you enter a badly formated email id or a password of length below 6 then FireBase Auth server will return the error message which we present to the user
Signing in existing user in FireBase
- Open LoginVC.swift and add the following code
@IBAction func signupbtnclicked(_ sender: Any) {
performSegue(withIdentifier: "segue", sender: self)
}
@IBAction func signinbtnPressed(_ sender: Any) {
if let email = emailtxtbox.text , let password = passwordtxtbox.text
{
Auth.auth().signIn(withEmail: email, password:password) { (authresultdata, error) in
if let err = error
{
self.showAlert(message: err.localizedDescription)
}
else
{
self.showAlert(message: "Success")
}
}
}
}
func showAlert(message: String)
{
let ac = UIAlertController(title: "Info", message: message, preferredStyle: .alert)
ac.addAction(UIAlertAction(title: "Ok", style: .default, handler: nil))
present(ac, animated: true, completion: nil)
}
Here we SignIn existing user using signIn() callback of the firebase.First we check if the email and password textbox is empty if not then send the email and password to auth server for authentication.The callback also returns an error to denote any authentication failure like invalid email or incorrect password which we can present to the user by using an AlertView Controller.
Checking the authenticated users in Server
Now when you login to your FireBase console and select authentication
Advantage of FireBase Auth
Save time on developing Webservices : It saves time by preventin the need for server side methods for different kinds of token verification to add social logins like Facebook and Google.All of them will be handled efficiently by Firebase
Detailed Analytics : FireBase authentication also comes with analytics feature which can be used to analyse users demographic data.
Disadvantage of using FireBase
-
Limited support for iOS features.
-
Unpredictable pricing though for hobby projects it is free.
-
Limited querying capabilities in Real Time NOSQL DataBase
-
Doesn't work in the countries that don't allow Google eg China
Congratulations you have learned how to add Auth feature in your app without need for any self made backend server.
Let's end this article with a quiz