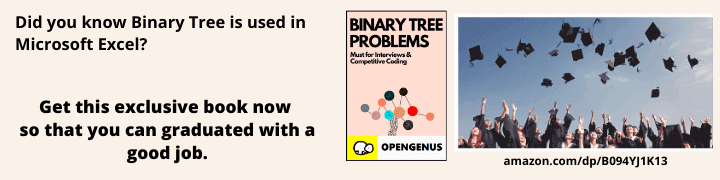
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will talk about what error handling by exception is in Java, how to do it, and what it is used for.
Table of contents:
- Exceptions review
- What is error handling by exception
- How to handle errors by exception in Java
- What handling errors by exceptions is used for
Exceptions Review
Exceptions in Java are an event that occur while a program is happening. This event disrupts the Java program, and when occurs, is handed to the runtime system.
What is error handling by exception
The Java language provides a way to handle exceptions: throwing and catching exceptions. This can be done with try-catch blocks. Code that might throw an exception is put into the try block, and the catch block is able to handle the error by exception. Essentially, we want to be able to have control over exceptions in our Java code and possibly do something during our program depending on what exception is thrown.
How to handle errors by exception in Java
By default, the java.lang.Exception import is included in Java programs. However, more specific libraries such as java.io.IOException and java.land.NullPointerException need to be imported.
Here is the basic syntax to throw and catch exceptions:
try {
// code that might throw an exception
} catch (Exception e) {
// code to handle the exception
}
Here is an example of a more complex try/catch scenario:
try {
// code that might throw an exception
} catch (NullPointerException e) {
// this code will be run if there is a
// NullPointerException thrown in the try block
} catch (IOException e) {
// this code will be run if there is a
// IOException thrown in the try block
} catch (ArrayIndexOutOfBoundsException e) {
// this code will be run if there is a
// ArrayIndexOutOfBoundsException thrown in the try
// block
} catch (Exception e) {
// this code will be run if an exception that is not
// already attempted to be caught by the above catch
// blocks is thrown
} finally {
// code in the finally statement will be executed
// regardless of if an exception is thrown - it will
// always execute if try is entered
}
Basically, through this structure, if a try block is entered and an exception is thrown by the code or by a throw statement, the try block will stop there and immediately jump to the catch statements, seeing if any of the catch statements are of the exception type that was thrown. Most of the time, it is useful to have multiple catch blocks to handle different exceptions differently. Although, since all exceptions are a subclass of Exception, having just one catch with "Exception e" as a target is able to catch all Exceptions.
It is useful and good style to have code within these catch blocks. For example, you could put a println statement in each of these blocks which outputs which specific exception was thrown. This way the programmer/user is able to see what specifically went wrong. Another idea would be to put code that helps the program as it carries on after exiting this structure, dependent on which exception was thrown.
Also, the "finally" statement is always executed. In essence, a try is run, 0-1 catch statements are executed, and a finally statement is executed.
Below is an implementation of try, catch, and finally in some code that reads data from a file:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileHandler {
public static void main(String[] args) {
BufferedReader reader = null;
try {
reader = new BufferedReader(new
FileReader("file.txt"));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.out.println("Error reading file: "
+ e.getMessage());
} finally {
try {
if (reader != null) {
reader.close();
}
} catch (IOException e) {
System.out.println("Error closing file: "
+ e.getMessage());
}
}
}
}
In this example, the code in the try block reads data from a file using a 'BufferedReader'. If an IOException is thrown, the catch block will handle it by printing an error message. The finally block is used to close the 'BufferedReader' and release any system resources that is was using. If an error occurs while closing the 'BufferedReader' the nested catch block inside the finally block will handle it by printing an error message.
What handling errors by exceptions is used for
Now that we know what exceptions are and how we can handle errors by exception, what is the point of doing this? Well, handling errors by exceptions is a fundamental feature of Java programming. It is used to detect and handle errors that may occur during the execution of a Java program. When an error occurs, Java creates an exception object that contains information about the error, including the type of error and the location of the code where it occurred.
Using exceptions allows you as a developer to write code that is more robust, reliable, and maintainable. By handling errors consistently, Java programs can provide better error reporting and handling. Further, although you should not use exceptions overly, when exceptions are used it is able to recover programs from errors in a graceful manner, as opposed to just crashing or producing incorrect results.
As you are writing Java code, here are some common scenarios where you might want to consider using error handling by exceptions:
- Input/output errors: Java programs often interact with files, network connections, and other external resources that may fail or become unavailable. Using exceptions allows programs to handle these errors in a consistent way, such as notifying the user or retrying the operation.
- Data validation: Java programs often need to validate user input or data from external sources. Using exceptions allows Java programs to detect and handle validation errors, such as invalid format or range errors.
- Database errors: Java programs often interact with databases, which can generate errors such as connection failures, syntax errors, or data integrity violations. Using exceptions allows Java programs to handle these errors in a consistent way, such as by rolling back transactions or retrying queries.
Overall, handling errors by exceptions is an important aspects of Java programming. It ensures that Java programs are reliable, robust, and maintainable.
With this article at OpenGenus, you must have the complete idea of error handling by exception in Java.