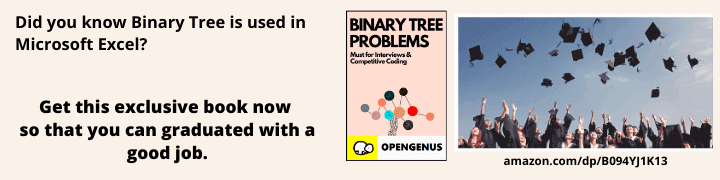
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have covered the essential components of Ruby such as OOP concepts like class and object, Data Types, Methods, Variables and much more.
Table of contents:
- Classes and Objects in Ruby
- Variables, Constants, Literals, Booleans and nil
- Numbers, Strings, Symbols, Arrays, Hashes, Ranges, Regular expressions, Procs, Percent Strings
- Data Types: Numbers, Strings, Symbols, Hashes, Arrays, Boolean
- Methods
Classes and Objects
A class is a blueprint, or a prototype, from which objects are created.
Objects are instances of classes. With respect to real-world, a car is a class, and a sedan is it's object.
A class in Ruby can be created in the following way-
class Car
end
Objects can be created in Ruby using the new method
class Hello
@@msg = "Hi there"
end
x = Hello.new
p x
---OUTPUT---
#<Hello:0x000055f13aacbe18>
Variables
Variables are the building blocks of any software. They are the labelled memory locations, that can be used to store a value type.
Creating a variable is really simple.
limit = 10
To use a variable, just type the variable name.
limit * 5
# which is 50
A variable can also interact with multiple other variables.
limit = 10
factor = 5
expected_value = 2.6
limit * factor
# which is equal to 50
total = expected_value + factor
#this works too
You are not just limited to integer type data.
The five types of variables in Ruby are:
-
Global variables:
Global variables starts with a $ sign. As the name suggests, it's scope is global, which means that it can be accessed from anywhere in the program. Global variables have nil as their default value.
Example: $name_of_variable -
Instance variables:
Instance variables start with a @ sign. They belongs to a single instance of the class, and can be accessed from any instance of the class within a method. They have private access specifier, but can be accessed. Just like global variables, an uninitialized instance variable will have a nil value. They have limited access to a given instance of that particular class, but can be accessed by using these methods:
instance_variables
instance_variable_defined?
instance_variable_set
instance_variable_get
remove_instance_variable
We can also use attr_accessor, attr_reader and attr_writer to allow access to the instance variables (of course, with the @ sign).
Another means of accessing is by using self.
self.x = 10
@x = 10
# Both of these statements are equivalent to each other
Example: @name_of_variable
- Class variables:
These variables start with the @@ sign. As the name suggests, they belong to the class, and can be accessed from anywhere inside the class. If the value is changed for a particular instance, it's value changes for all of it's instance. Class variables are shared by the child of that given class or module in which the particular variables were defined.
Example: @@name_of_variable
- Local variables:
Local variables in Ruby begin with a lowercase letter or _. Their scope ranges from either within their class, module, def, or a block (either do ... end or {})
Example: name_of_variable or _name_of_variable
Constants
Constants are either in complete uppercase, or start with one uppercase letter.Constants can be accessed within a class/model, and those outside can be accessed globally.
Example: NAME_OF_CONSTANT
Literals
Note!!: Literals may appear the same as data types, except that they're not!! Literals are the data representation of fixed values. For example, 10, 'e', 7.6, etc.
x = 10 is a variable, and so is name = "john doe"
Types of literals in Ruby are:
Types |
---|
Booleans and nil |
Numbers |
Strings |
Symbols |
Arrays |
Hashes |
Ranges |
Regular Expressions |
Procs |
Booleans and nil
nil and false both denote the false value. nil is used to denote "no value", but conditionally evaluate to false.
true is a true value. All objects, except nil and false evaluate to a true value.
true, nil and false can also be written as TRUE, NIL and FALSE - although the lowercase forms have better readibility.
Numbers
Numbers may be of any size. You can also add in an underscore in between for better readibility (as seperators).
1234
# is the same as
1_234
Floating points may be written as
12.34
1234e-2
1.234E1
Prefixes may be added to write numbers in decimal, hexadecimal, octal as well as binary formats.
The prefixes are-
Type | Prefix |
---|---|
Decimal | 0d or 0D |
Hexadecimal | 0x or 0X |
Octal | 0o or 0 |
Binary | 0b or 0B |
Examples
0d234
0xaB
0255
0b011010101
Like integers and floats, underscores can be used for readibility.
Strings
The most common way to write a string is:
"A string"
The main difference between a single and a double quotes string is that double quotes support interpolation.
"one plus one = #{1+1}"
# gives: one plus one = 2
'one plus one = #{1+1}'
# gives: one plus one = #{1+1}
Interpolation in double quotes can be disabled by adding the escape character "" in front of the hash(#) symbol.
Strings can also be created with %. %q(...) represents single quotes string, whereas %Q(...) represents double quotes string.
In order to add a large block of text, we should use a here document or "heredoc".
x = <<HEREDOC
First line
Second line
Ending...
HEREDOC
# "First line\nSecond line\nEnding...\n"
Note that anything can be written instead of the HEREDOC - but an uppercase identifier must be used.
If suppose you want to indent the HEREDOC, you may also add a - before the literal
f = <<-WHAT
Oh, I see...
Let me end this
WHAT
# "Oh, I see...\nLet me end this\n"
If you want to avoid the string interpolation, just add a single quotes to the opening identifier
expect = <<-'THIS'
Two plus two is #{2+2}
THIS
# "Two plus two is \#{2+2}\n"
Symbols
A symbol represents a name inside the interpreter. Symbols can be referenced by using a colon :my_symbol. Symbols can also be created by interpoltion - and we can also use single quotes to remove interpolation.
:my_symbol
# :my_symbol
:"my_symbol"
# :my_symbol
:"my_symbol#{1+1}"
# :my_symbol2
:'my_symbol#{1+1}'
# :"my_symbol\#{1+1}"
Arrays
An array is created using the objects between [ and ].
[1, 2, 3]
Hashes
A hash is created using key-value pairs between { and }.
{ "a" => 1, "b" => 2 }
We can also use a sybmol inside a hash.
{ a: 1, b: 2}
Ranges
A range represents an interval of values. They may include or exclude it's ending values.
(1..5) # will include 5
(1...5) # will exclude 5
Regular expressions
A regular expression is created using "/"
/a regular expression/
These regex may be followed by a case.
/a regular expression/i
Procs
A proc is created with ->
-> { 1 + 1 }
We can also add arguments for the proc.
->(x) { 1 + x }
Percent Strings
%(...) is useed to create a string. Upper case letters allow interpolation, whereas lower case letters disable them.
The types of percent strings in Ruby are:
- %i or %I - Arrays or symbols
- %q or %Q - String
- %r or %R - Regular Expression
- %s or %S - Symbol
- %w or %W - Array of strings
- %x or %X - Backtick (capture shell result)
For the two array forms of percent string, an escape character must be used if you wish to use space
%w[one one-hundred\ one]
# ["one", "one-hundred one"]
If any brackets are being used, it must be closed as well.
Data Types
Numbers
Numbers, as the name suggests, talks about integers and floating points.
Class | Description | Example |
---|---|---|
Integer | Holds the integer value | 10 |
Fixnum | Integers that can fit into the OS assigned integer types of 32-64 bit (deprecated into Integer in 2.4) | 45 |
Bignum | For large-sized Integers (deprecated into Integer in 2.4) | 134534563463 |
Float | Non-precise decimal values | 7.0 |
Complex | Used to express complex numbers expressed in the form a+bi where a and b are real numbers, and i is an imaginary unit | 7+5i |
Rational | For representing fractions | 7/2 |
BigDecimal | Decimals with perfect precisions.Although similar to float, precision can lead to slower performance | 9.86 |
Strings
Strings are a sequence of characters, enclosed inside quotes (either single or double quotes). Characters may include numbers, symbols or alphabets.
"apple"
A variable can be used to represent a string-
word='apple'
What makes Ruby truly beautiful than most of the scripting languages are the rich sets of methods that can come in handy for text processing and string manipulation.
Symbols
Symbols are data types that can be used to represent other data types. Althoug it may look similar to a string, symbols are actually immutable - that is, only one copy of a symbol can be created, as opposed to string, where multiple objects can be created
p :demo.object_id
p :demo.object_id
# creating a new symbol does not change the object id
p "demo".object_id
p "demo".object_id
# creating a new string changes it's object id
---OUTPUT---
p :demo.object_id
p :demo.object_id
# creating a new symbol does not change the object id
p "demo".object_id
p "demo".object_id
# creating a new string changes it's object id
Symbols may be used as flags, but we can also use constants.
Symbols are used in hashes, as keys, because of their efficiency.
student = {:name => "Aaron", :age => 25, :job => "Neurosurgeon"}
puts student[:name]
---OUTPUT---
Aaron
Hashes
A hash is a collection of key-value pair.
It is similar to an array, except that values are represented not by indices, but by a particular key.
A hash can be created in two different ways-
p months = {"1"=> "January", "2"=> "February", "3"=> "March"}
day = Hash.new
day["first"] = "Monday"
day["second"] = "Tuesday"
p day
---OUTPUT---
{"1"=>"January", "2"=>"February", "3"=>"March"}
{"first"=>"Monday", "second"=>"Tuesday"}
Arrays
An array stores data in ordered, integer-indexed collection. Data in an array can be of any type. An array starts with a 0, as is seen in other languages. Ruby also features the negative index feature - arrays are taken from the last number. So, an index of -1 indicates the last element, an index of -2 indicates the second last element, and so on.
Arrays can be declared the following way-
p greet = Array.new(10, "Hello")
p num = Array(0..15)
p name = ["Aaron", "Mike", "Arun", "Yuki", "Sophie"]
---OUTPUT---
["Hello", "Hello", "Hello", "Hello", "Hello", "Hello", "Hello", "Hello", "Hello", "Hello"]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
["Aaron", "Mike", "Arun", "Yuki", "Sophie"]
Booleans
Boolean type represents whether the given value is true or false.
It can be simply declared in the following manner -
bool1 = true
bool2 = false
if bool1|bool2
puts "Okay"
else
puts "Not okay"
end
---OUTPUT---
Okay
Methods
Methods in Ruby start with def followed by the name of the method. Methods should always begin with a lowercase.
def method_name
# Put something here
end
To add parameters:
def method_name (var1, var2)
# Put something here
end
To set a default value:
def method_name (var1 = value1, var2 = value2)
# Put something here
end
To call a method, we write:
method_name
To call a method with parameters:
method_name(25, 30)
# OR
method_name 25, 30
Every method in Ruby returns a value - which is the last statement. For example:
def check
i=100
j=34
k=60
end
# returned variable is k
We can also use the return statement to make the methods readable, or to send multiple parameters.
To create a method with variable parameters, we need to add a parameter with an asterisk as the prefix:
def check(*val)
puts "The number of parameters is #{val.length}"
for i in 0...val.length
puts "The parameters are #{val[i]}"
end
end
check "Tom", "16", "M"
---OUTPUT---
The number of parameters is 3
The parameters are Tom
The parameters are 16
The parameters are M
A private method is when a method is defined outside the class - they are private by default. A method defined inside the class is called a class method - and they are public by default.
To define a class method:
class Car
def type
end
def Car.wheel # to use a method without instantiating the class
end
end
The alias keyword in RUby is used to deal with methods and their attribute aliases
class Car
def carname
'Ford Mustang'
end
alias modelname carname
alias brandname modelname
end
c = Car.new
p c.carname # => 'Ford Mustang'
p c.modelname # => 'Ford Mustang'
p c.brandname # => 'Ford Mustang'
There is also a keyword to cancel the method definition - called the undef statement, and the syntax is undef method-name.
def hello
"Hello World"
end
hello # => "Hello World"
undef hello
hello # => NameError (undefined local variable or method `hello' for main:Object)
With this article at OpenGenus, you must have strong hold on Essential components of Ruby.