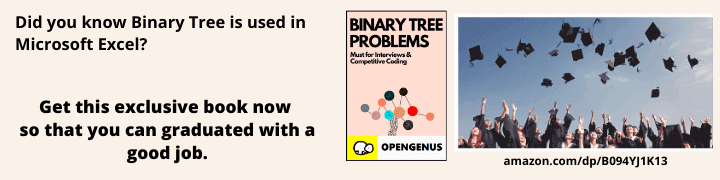
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Hibernate is an open-source Java ORM tool that is used between the Java application and the JDBC that connects to the database. To put the hibernate into work we need to provide three things:
- Hibernate Configuration - to configure caching strategies, SQL statement logging in the console, etc.
- Database connection details - database credentials like driver class, username, password, and connection URL, to connect to the database
- Mapping details - to map the entity classes to database tables
We can configure hibernate through Java configuration or XML configuration and the mapping details can be provided through XML or Java Annotations.
In this post, we are going to see the most commonly used hibernate annotations that we require for the mapping of Java Entity classes to the database tables.
Most Commonly used annotations
- @Entity - Marks a class as entity class
- @Table - Defines the properties of the database table
- @Column - Defines the properties of table columns
- @Id - Declares entity field as the primary key of the database table
- @GeneratedValue - Defines primary key generation strategy
- @Version - Controls versioning
- @Transient - Marks the attribute as transient
- @Temporal - Defines data format
@Entity
This annotation is the most used and required while using hibernate framework. This annotation belongs to the "javax.persistence" package. From hibernate version 5.5.0 Final the hibernate uses jakarta interfaces. So, the "@Entity" annotation belongs to "jakarta.persistence".
Note: The name of packages has changed from javax.*. * to jakarta. * . * . All the classes, interfaces, and annotations work the same way.
This annotation is used at the class level to declare a class as a persistence entity. This entity has one attribute, name, which is used to define the name of the entity. By default, the name of the entity is the same as the name of the class. Example
@Entity(name = "user")
public class User {
@Id
private Integer id;
private String name;
private String email;
//constructors, getters, and setters
}
Here, in the above example, we have declared @Entity annotation over User class. By default, the name of the entity would be User. Since we have supplied a value for the name attribute in the annotation the name changed from "User" to "user".
@Table
This is a class-level annotation that is used to define the name of the database table. By default, the name of the table is the name of the class in cameCase letters. We can change the name of the table by supplying the value of the name attribute of the annotation. Example-
@Entity(name = "user")
@Table(name = "users")
public class User {
@Id
private Integer id;
private String name;
private String email;
//constructors, getters, and setters
}
By default the name of the table would be user but, as we have supplied the value of the name attribute of the @Table attribute the name of the table in the database would be users.
Note: The default name of the table and the entity is camelCase and the PascalCase version of the name of the class. Essentially, tables and entities are not the same.
@Column
This annotation is used to define the properties of the database column. Using this annotation we can define the name, length, nullable, insertable, updatable, etc. attributes of the column. Example-
@Entity(name = "user")
@Table(name = "users")
public class User {
@Id
@Column(name = "user_id", unique = true)
private Integer id;
@Column(name = "fullname", length = "200")
private String name;
private String email;
//constructors, getters, and setters
}
@Id
This annotation is used to define the primary key of the entity in the database table. It is a required annotation that we must define in entity classes to uniquely identify the records. Example-
@Entity(name = "user")
@Table(name = "users")
public class User {
@Id
@Column(name = "user_id", unique = true)
private Integer id;
@Column(name = "fullname", length = "200")
private String name;
private String email;
//constructors, getters, and setters
}
Here, id attribute is used to uniquely identify the data records.
@GeneratedValue
This annotation is used to define the automatic key generation strategy of the primary key. If we want the primary key to be generated itself we can use this annotation. It is generally used in conjunction with @Id annotation.
It can use four generation strategies: AUTO, IDENTITY, SEQUENCE, and TABLE.
If we don't explicitly define the strategy it defaults to AUTO.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@Column(name = "fullname", length = "200")
private String name;
private String email;
//constructors, getters, and setters
}
GenerationType.IDENTITY is like auto increment.
@Version
This annotation is used to track the version of records in the database. The default value of the version is 0, initially. After that whenever we update the record we must provide the current version. After updating the record the version will increase by one. Example-
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@Column(name = "fullname", length = "200")
private String name;
private String email;
@Version
private Integer version;
//constructors, getters, and setters
}
Initially, when the table will be created the value of the version will be zero. Then whenever we update any or all of the fields of the entity the version will increase by one.
@Transient
This annotation is used to mark the class attribute as transient which means there will no column in the table with the name of the particular column. Example-
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@Column(name = "fullname", length = "200")
private String name;
private String email;
@Transient
private Integer version;
//constructors, getters, and setters
}
In the example above, as we have marked the version member as transient, in the database table only id, fullname, and email columns will be available. There will be no column for the version attribute.
@Temporal
This annotation must be specified for persistent fields or properties of type java.util.Date and java.util.Calendar. It may only be specified for fields or properties of these types. It is used to define the precision of temporal data in the database.
It can have three values DATE(only date), TIME(only time), or TIMESTAMP(date and time). Example-
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@Column(name = "fullname", length = "200")
private String name;
private String email;
@Temporal(TemporalType.DATE)
private Date dob;
//constructors, getters, and setters
}
In the example above, only the date will be persisted in the database table.
The above described are the most commonly used annotations that we use almost every time we make persistent classes. In addition to these annotations there, a lot of other annotations are available in hibernate those specific to a particular situation like annotation mapping and inheritance mapping.
- @OrderBy - Defines sorting of records
- @Lob - Defines binary large objects like imgaes
- @Embeddable - Marks a class as embeddable
- @Embedded - Marks an instance variable as embedded
- @EmbeddedId - Marks an instance variable as an embedded reference which is a composite key of the entity
- @OneToOne - Defines one to one mapping
- @OneToMany - Defines one to many mapping
- @ManyToOne - Defines many ot one mapping
- @ManyToMany - Defines many to many mapping
- @Inheritance - Defines type of inheritance mapping
- @DiscriminatorColumn - Defines discriminator column ffor an entity
- @DiscriminatorValue - Defines discriminator value for an entity etc.
Happy Coding!
Keep Exploring!