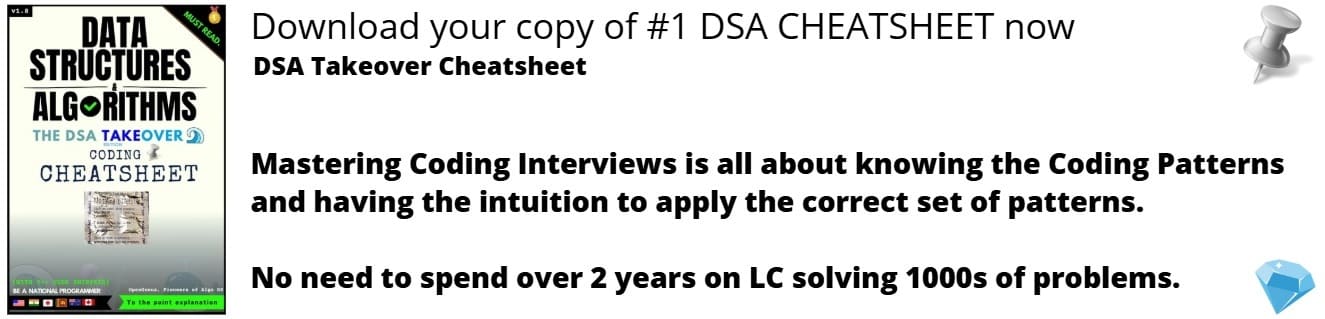
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn about the data structure Nested dictionaries in the python. Also, we will learn about creating, accessing, modifying and iterating through them.
Table of contents:
- What is a Dictionary?
- What are Nested Dictionaries?
- Different ways of creating Nested Dictionary
- Accessing the elements in a Nested Dictionary
- Adding the Nested Dictionary Items
- Updating the Nested Dictionary Items
- Merge the two Nested Dictionaries
- Removing Nested Dictionary items
- Iterating through Nested Dictionary
Dictionary
Dictionary is the unordered and mutable python data structure which stores mappings of unique keys to values.
It can also be treated as hashmaps
in C++
, or json
in JavaScript
.
Note: Dictionaries key is case sensitive.
dict1 ={'key1':'value1','key2':'value2','key3':'value3','key4':'value4'}
Here above, we declare a dictionary named dict1
which starts with curly braces({}),including all key-value pairs seperated by (,) and colon(:) seperating each key from its value.(key1, key2, key3, key4) are the respective unique keys and (value1, value2, value3, value4) are the respective values assigned to keys.
Nested Dictionary
Nested Dictionary basically means putting a dictionary inside another dictionary.
nested_dict = {'id1':'Fred','id2':'Hughes','dict2':{'id3':'Philips','id4':'Jock'}}
Here, we see that we have regular key-value pairs including a dictionary dict2
which has 2 key-value pairs describing nested_dict
as Nested Dictionary.
Different methods of creating a nested dictionary in Python
There are two ways of creating a Nested Dictionary:
- Just like normal dictionary, we can create the nested dictionary only difference being each value can be a dictionary or a single
key-value
pair.
dictA = {'student1':{'address1':'Las Vegas'},
'student2':{'address2':'Delhi'},
'student3':{'address3':'Texas'},
'student4':{'address4': 'Mumbai'}}
- Also, we can construct Nested dictionary from the constructor
dict()
. For that, we need to pass key-value pairs into the keyword argumentdict()
.
dictB = dict(Person1':{'House_number':01,'Colony':'Grape street'},
'Person2':{'House_number':033,'Colony':'Freds street'},
'Person3':{'House_number':21,'Colony':'Driver street'})
We can also create dictionary with default values for each key using fromkeys()
method.
CustID = ['cust1','cust2','cust3','cust4']
Default = {'name': '', 'Shops_visited': }
Customer = dict.fromkeys(CustID, Default)
print(Customer)
# Prints {'cust1': {'name': '', 'Shops_visited': ''},
# 'cust2': {'name': '', 'Shops_visited': ''},
# 'cust3': {'name': '', 'Shops_visited': ''},
# 'cust4': {'name': '', 'Shops_visited': ''} }
Accessing the elements in a nested dictionary
The individual keys,values can be accessed using square brackets([])
.
dictB = {'Person1':{'House_number':1,'Colony':'Grape street'},
'Person2':{'House_number':33,'Colony':'Freds street'},
'Person3':{'House_number':21,'Colony':'Driver street'}}
On this dictionary, if we want to get the House_number
of Person2
we will use print statement:
print(dictB['Person2']['House_number'])
# output is 33
If there is a reference to key that is not present in nested dictionary, then its produces an exception:
print(dictB['Person1']['city'])
This statement output will be an exception of keyerror which will be key not present in dictB
named city.Precisely:
#keyerror: 'city'
To prevent this from happening, we can use the special dictionary method get().
get()
gives the value for its respective key if they are present in the dictionary otherwise it returns None
which means this method will never raise an keyerror
.
Adding items to nested dictionares in Python
If we want to add the new item, value or key to the dictionary we can add the Dictionary related to it.
dictA = {'student1':{'address1':'Las Vegas'},
'student2':{'address2':'Delhi'},
'student3':{'address3':'Texas'}}
Suppose in dictA
, we will add a student3 we proceed as:
dictA['student4'] = {'address4':'Tokyo'}
This adds in new dictionary added in the nested dictionary.And now, dictA looks like this:
dictA = {'student1':{'address':'Las Vegas'},
'student2':{'address':'Delhi'},
'student3':{'address':'Texas'},
'student4':{'address':'Mumbai'}}
Also, we can say that the dictA
has been updated as we have added an item to it.
Suppose we want to change any certain key or value or item in nested dictionary,we refer the item by its key and corresponding value and change it to what we want.
dictA = {'student1':{'address':'Las Vegas'},
'student2':{'address':'Delhi'},
'student3':{'address':'Texas'},
'student4':{'address':'Mumbai'}}
Updating the items stored in a nested dictionary
Suppose the student3 change his address to Paris from Texas.Here, how we can do this:
dictA['student3']:{'address':'Paris'}
print(dictA)
#prints {'student1':{'address':'Las Vegas'},
'student2':{'address':'Delhi'},
'student3':{'address':'Paris'},
'student4':{'address':'Mumbai'}}
Now the data of student3
has been updated with new address Paris
.
Merging the two nested dictionaries in Python
-
The python inbuilt
update()
method can be used to merge the two nested dictionaries(the way it does is by merging keys and values of the dictionaries into one another). -
One thing to note is here, that
update()
will overwrite value of the keys which are same and henceforth clash with each other which will be overwritten.
dictV = {'employee1':{'name':'Andrew','job':'System engineer','address':'Delhi'},
'employee2':{'name':'John','job':'AI scientist','address':'hyderabad'},
'employee3'{'name':'Steph','job':'salesperson','address':'banglore'} }
dictM = { 'employee3'{'name':'Steph','job':'salesperson','address':'banglore'},
'employee4'{'name':'Nicolas','job':'Project Manager','address':'Mohali'}}
dictV.update(dictM)
print(dictV)
#printed data:
{'employee1':{'name':'Andrew','job':'Systemengineer','address':'Delhi'},
'employee2':{'name':'John','job':'AI scientist','address':'hyderabad'},
'employee3'{'name':'Steph','job':'salesperson','address':'banglore'},
'employee4'{'name':'Nicolas','job':'Project Manager','address':'Mohali'}}
- Notice, one of the
employee3
key which was doubled has been removed and we have only 4 dictionaries in nested dictionaries which tells that one repeated dictionary indictM
has been removed.
Removing the nested dictionaries items in Python
There are various ways to remove the items in nested dictionaries, some of them are using pop()
method etc.
- By using the key , we can remove that key and its respective value using
pop()
method.
dictV = {'employee1':{'name':'Andrew','job':'Systemengineer','address':'Delhi'},
'employee2':{'name':'John','job':'AI scientist','address':'hyderabad'},
'employee3'{'name':'Steph','job':'salesperson','address':'banglore'},
'employee4'{'name':'Nicolas','job':'Project Manager','address':'Mohali'}}
popped_item = dictV.pop('employee1')
print(dictV)
print(popped_item)
#Prints:
# {'employee2':{'name':'John','job':'AIscientist','address':'hyderabad'},
# 'employee3'{'name':'Steph','job':'salesperson','address':'banglore'},
# 'employee4'{'name':'Nicolas','job':'Project Manager','address':'Mohali'}}
#popped_item: {'employee1':'name':'Andrew','job':'Systemengineer','address':'Delhi'}
- Suppose, we dont need the popped value for any purpose, we can remove it by
del
method.
dictV = {'employee1':{'name':'Andrew','job':'Systemengineer','address':'Delhi'},
'employee2':{'name':'John','job':'AI scientist','address':'hyderabad'},
'employee3'{'name':'Steph','job':'salesperson','address':'banglore'},
'employee4'{'name':'Nicolas','job':'Project Manager','address':'Mohali'}}
del.dictV['employee1']
print(dictV)
#prints:
{'employee2':{'name':'John','job':'AIscientist','address':'hyderabad'},
'employee3':{'name':'Steph','job':'salesperson','address':'banglore'},
'employee4':{'name':'Nicolas','job':'Project Manager','address':'Mohali'}}
- If we want to pop the last item in the nested dictionary , we can use a
popitem()
to do the same.
dictV = {'employee1':{'name':'Andrew','job':'Systemengineer','address':'Delhi'},
'employee2':{'name':'John','job':'AI scientist','address':'hyderabad'},
'employee3':{'name':'Steph','job':'salesperson','address':'banglore'},
'employee4':{'name':'Nicolas','job':'Project Manager','address':'Mohali'}}
poped_last = dictV.popitem()
Here, we will now print the output of dictV
and poped_item
.We expect the output to be dictV
excluding the employee4
key(also its corresponding value will be removed as well) and poped_last
will be that poped last item having key employee4
.
Output:
print(dictV)
print(poped_last)
#prints:
{'employee1':{'name':'Andrew','job':'Systemengineer','address':'Delhi'},
'employee2':{'name':'John','job':'AI scientist','address':'hyderabad'},
'employee3'{'name':'Steph','job':'salesperson','address':'banglore'}}
{'employee4'{'name':'Nicolas','job':'Project Manager','address':'Mohali'}
Iterating through nested dictionaries in python
All values in nested dictionaries can be iterated withn the help of for loop
mostly being nested one.
dictV = {'employee1':{'name':'Andrew','job':'Systemengineer','address':'Delhi'},
'employee2':{'name':'John','job':'AI scientist','address':'hyderabad'},
'employee3':{'name':'Steph','job':'salesperson','address':'banglore'},
'employee4':{'name':'Nicolas','job':'Project Manager','address':'Mohali'}}
for id in dictV:
print('Employee ID-',id)
for key in dictV[id]:
print(key +':', dictV[id][key])
Here, we get through loop and searching for id which tells us the employee id of employees.Then, when we second for loop we will print out the values corresponding
to that key , hence giving us the required print statement.
here is the output of above code:
#output
'''
Employee ID- employee1
name: Andrew
job: Systemengineer
address: Delhi
Employee ID- employee2
name: John
job: AI scientist
address: hyderabad
Employee ID- employee3
name: Steph
job: salesperson
address: banglore
Employee ID- employee4
name: Nicolas
job: Project Manager
address: Mohali
'''
With this article at OpenGenus, you must have the complete idea of Nested Dictionary in Python.