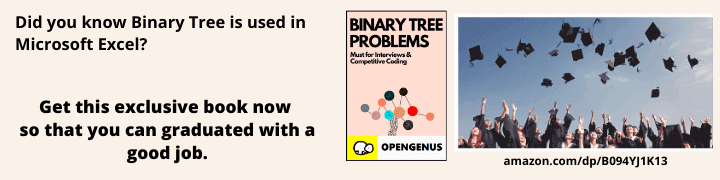
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the idea of ifstream in C++ and explained how it is used with C++ code examples.
A stream is a sequence of data(bytes) and is used for the transportation of this data. It works as a medium to bring data into a program from a source or to send data from the program to a specific destination. The source can be a file, an input device, and the same can be said for the destination. We have the following two types of streams:
- Input Stream: A stream which takes data from a file or input device and brings it into the program, is called as input stream.
- Output Stream: A stream which takes data from the program and send it to a file or output device is called as output stream.
Table of Content:
- Introduction
- input file stream
- Hierarchy of Stream Classes
- Advantages of ifstream classes
- Member functions of ifstream class
- Conclusion
Introduction:
We have commonly used the iostream standard library, which provides the cin and cout methods for reading streams from standard input and writing streams to standard output, respectively.
Now if we have to read streams from and write streams to files. This requires the use of another standard library fstream in C++ , which defines three new data types:
Data Types | Description | |
---|---|---|
ofstream | This data type represents the output file stream, which is used to create files and write information to them. | |
ifstream | This data type represents the input file stream, which is used to read information from the file. | |
fstream | This data type usually represents a file stream and has both ofstream and ifstream functions, which means it can create files, write information to files and read information from files. |
For file handling in C++, the header files iostream and fstream must be included in the C++ source code file.
#include <fstream>
#include <iostream>
using namespace std ;
Input file stream
In C++, the ifstream class is used to realize the file reading operation.
Syntax:
ifstream object_name.open(“file_name”);
Note : When you open file using ifstream class then file is default opened for reading. If you open file using ofstream class then file is default opened for writing purpose.
Implementation:
Let's say we have a text file as follows and named as 'myfile.txt'. Its contents are:
I love programming at OpenGenus.
Here's the basic implemented code:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
std::ifstream ifs("myfile.txt");
if (!ifs) {
std::cerr << "Failed to open file" << std::endl;
std::exit(1);
}
std::string buf;
std::getline(ifs, buf);
if (!ifs) {
std::cerr << "Failed to load"<< std::endl;
std::exit(1);
}
std::cout << buf << std::endl;
}
Output:
I love programming at OpenGenus.
Explanation:
- First we create the object of ifstream directly.
- The process of creating this object automatically calls the open method. this method open the file for us reading.
- The std::getline function specifies the input stream for receiving the data from file.
- The character string for one line received is stored in the variable specified.
- To close the file we use close() with stream object.Not closing streams can cause memory leaks.
Hierarchy of Stream Classes
Several stream classes commonly used in C++ and their interrelationships are shown:
- istream is the stream class used for input, and cin is the object of this class.
- ostream is the stream class used for output, and cout is the object of this class.
- ifstream is a class for reading data from a file.
- ofstream is the class used to write data to a file.
- iostream is a class that can be used for both input and output.
- fstream is a class that can both read data from a file and write data to a file.
Member functions of ifstream class
gcount()
streamsize gcount() const;1
Returns the number of characters read by the last input operation. This function is inherited from istream class.
get()
Read a character, encounter '\n', also take it out from the stream. This function is inherited from istream class.
#include <fstream.h>
int main() {
ifstream in("MyFile.txt");
char c;
while(!in.eof())
{
c = in.get();
cout << c;
}
cout << endl;
return 0;
}
getline()
Read a line into a character array. This function is inherited from istream class.
#include <fstream.h>
int main() {
ifstream in("MyFile.txt");
char Target[300];
in.getline(Target, sizeof Target);
cout << Target << "\n";
return 0;
}
peek()
Returns the next character in the input stream and leaves it in the input stream as the starting point for the next read. The return value is an integer ascii code value, which can be converted into characters with char(c). This function is inherited from istream class.
Advantages of ifstream classes
- Provides the input functionality
- inherits functions like get(), getline() from istream
- Opens file with default read mode.
Conclusion
In this article at OpenGenus, we learn about the ifstream class and how to open a file for reading using it in a code.