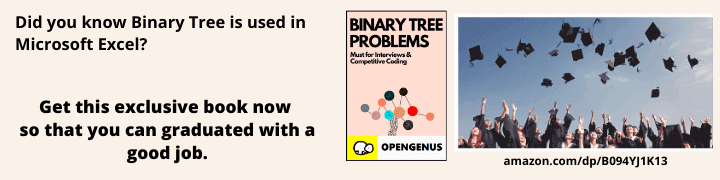
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have presented 65 Interview questions on Hibernate covering several topics including 10 Multiple choice questions (MCQs) and 55 Descriptive questions with answers.
Table of contents:
Object Relational Mapping basics
1. What is ORM?
Object-Relational Mapping (ORM) is a technique to query and manipulate data from a database using an object-oriented paradigm. The Hibernate ORM helps to automate communication between the Java application and SQL database and takes care of the mapping from Java classes to database tables, and from Java data types to SQL data types.
2. Name a few ORM frameworks based on JAVA
The most popular ORM for JAVA is Hibernate, other popular frameworks are iBATIS, DataNucleus, or TopLink by Oracle.
Hibernate basics
3. What are the benefits of Hibernate?
Hibernate maps Java classes and types into the databases, which solves problems of "paradigm mismatches" between how data is represented in application objects versus relational databases. It supports object-oriented query language Hibernate Query Language that is automatically translated into the database dialect, so there is no need to manually work with JDBC API and address database changes. For quick performance, it uses proxy objects for initialization on demand and caching, both build-in and integrated caching providers.
Hibernate is easy to integrate with other Java frameworks like Spring, and it is also possible to write implementation-independent code as Hibernate supports Java Persistence API and annotations.
Finally, it is an open-source project with over 20 years of history of development that stays up-to-date with the newest technologies. There are lots of available tutorials, easy-to-follow documentation, and a helpful community.
4. What are the disadvantages of Hibernate?
Performance cost - Hibernate generate SQL queries based on the mapping. In large projects Hibernate with the wrong settings can cause performance issues.
Startup performance - when Hibernate loads, it analyzes all entities and does a lot of pre-caching, with a total time of about 5-10-15 seconds for a not very big application.
Less control on the database level as Hibernate requires exclusive write access to the data it works with. For example, database views, stored procedures, and triggers should not cause data changes, as Hibernate is unaware of those. Also, Hibernate does not allow multiple inserts in a single query.
Learning curve - multiple configurations and annotations are an advantage and provide lots of flexibility but require time and effort to learn.
5. Can you explain the architecture of Hibernate?
Hibernate works as an additional layer between Java application and database, above the Java Database Connectivity (JDBC) API.
To isolate dependencies, Hibernate's functionality is split into several modules. Some of the important modules include:
- hibernate-core - ORM features and APIs
- hibernate-encache - integrates Encache as a second-level cache provider
- hibernate-spatial - integrates GIS data type support
- hibernate-osgi - support for running in OSGi containers
6. Java APIs used by the Hibernate architecture
By default Hibernate uses JDBC (Java Database Connectivity) API for persistence. The mechanism for dealing with physical transactions in JDBC are JDBC itself and JTA (Java Database Connectivity), Hibernate supports both of them with org.hibernate.engine.transaction.internal.jdbc.JdbcTransactionFactory
and org.hibernate.engine.transaction.internal.jta.JtaTransactionFactory
classes.
Hibernate optionally can interact with JNDI (Java Naming Directory Interface) to access the directory and naming services, in a case when the application has asked the SessionFactory to be bound to JNDI, has specified a DataSource to use by JNDI name, or is using JTA transactions and the JtaPlatform needs to do JNDI lookups for TransactionManager, UserTransaction, etc. In all cases, the calls route through a single service org.hibernate.engine.jndi.spi.JndiService
.
7. What is the difference between Hibernate over JDBC?
Behind the scenes, the Hibernate Session
class wraps a JDBC java.sql.Connection
and acts as a factory for transactions, that allow executing queries with Java entities.
In addition to SQL requests handling, Hibernate supports associations, collections, inheritance, polymorphism, caching mechanisms, and other features that JDBC requires developers to solve manually. The code with Hibernate is easier to support and scale, especially for large projects, thanks to the elimination of boiler-plate code often found in JDBC.
JDBC is still a great tool though, particularly for smaller, more straightforward applications, or when the performance plays the key role.
8. What databases Hibernate support?
Hibernate supports all popular relational databases like MySQL, PostgreSQL, Oracle, Ingres, and others.
In addition to relation databases, the OGM engine extends Hibernate functionality to support NoSQL datastores like MongoDB and Infinispan.
9. What is a dialect in Hibernate?
Although SQL is relatively standardized, each database vendor uses a subset and superset of ANSI SQL-defined syntax or the so-called database’s dialect. Hibernate handles variations across these dialects through the org.hibernate.dialect.Dialect
class and the various subclasses for each database vendor.
Hibernate can automatically detect the required for the database, for example, the MySQL5
dialect for MySQL database, and generate the SQL statements with correct syntax.
JPA basics
10. How is Hibernate related to JPA?
JPA (Java Persistence API) is a specification for the management of persistence and object/relational mapping with Java EE and Java SE. The Hibernate implements JPA, as well as Hibernate native API that provides additional functionality.
Before JPA Hibernate used XML files for mapping, now the annotations allow to specify mapping right in the Java code and thus exclude XML-related errors.
11. What are persistent classes?
Persistent classes are Java classes whose objects or instances will be stored in database tables, they implement the entities of the business problem, for example, Customer or Order. JPA requires classes to correspond to the POJO requirements, the Hibernate is not as strict, for example, for Hibernate entity Class does not need to be a top-level class.
Not all instances of a persistent class are considered to be in the persistent state though, in the JPA lifecycle instance may also be transient, detached, or removed.
12. Can you declare an Entity class as final in Hibernate?
Java Persistence specification requires that Entity class, its persistent variables, and method must not be final. Technically Hibernate can persist final classes or classes with final persistent state accessor (getter/setter) methods.
However, it is generally not a good idea as doing so will stop Hibernate from being able to generate proxies for lazy-loading the entity, as proxies are generated by extending the entity bean.
13. Do we need to make an Entity class Serializable?
There is no Hibernate requirement for entities to implement a Serializable interface, however, it is useful to make entities serializable in the prospect of future interactions: to store objects in the session, to remotely access objects via RMI, etc.
14. What will happen if we don’t have a no-args constructor in the JPA Entity bean?
Jakarta Persistence requires embeddable classes to follow Java Bean conventions, part of this is the definition of a non-arg constructor.
When Hibernate creates an instance of entities using reflection it uses the Class.newInstance()
method (usually on get() or load() methods), which requires a no-argument constructor to create an instance, this is effectively equivalent to the new Entity()
. This method throws InstantiationException
if there are no no-argument constructor in the Entity class, that's why it's not necessary, but is better to provide a no-argument constructor.
The default constructors, getters, and setters take a lot of space in the code, the better practice is to hide them with tools like Lombok that provide annotations @NoArgsConstructor
, @Getter
, and @Setter
.
15. What are the states of an object in Hibernate (of an entity bean)?
In the context of Hibernate's Session, objects can be in one of four possible states: persistent (managed), new (transient), detached, and removed.
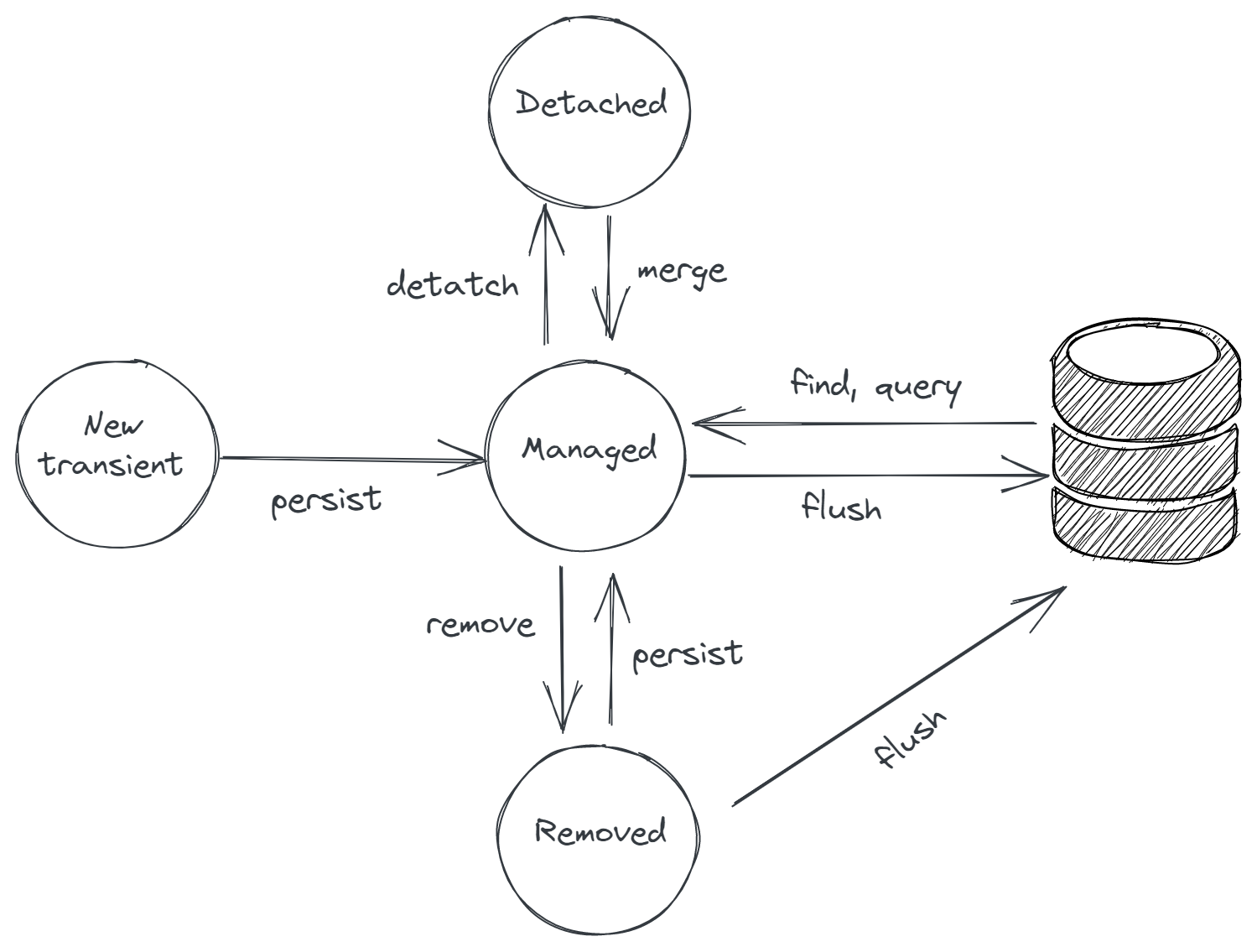
A newly created object is not associated with the Hibernate Session (Persistent context) and is not mapped to the database yet. An object associated with a session is in the persistent state, and all changes to that object are going to be detected and propagated to the database during the Session flush-time. Once the currently running session is closed, all the previously managed entities become detached, so none of the changes is tracked and synchronized to the database. The entity in the removed state is scheduled for deletion from the database, the actual DELETE SQL statement is executed later, during session flush-time.
16. What is automatic dirty checking in Hibernate?
Hibernate performs an inspection of all persistent entities associated with a session context when the session is flushed and compares each entity’s property values to a saved snapshot of the last known state of an entity in regards to the database (typically the last read or write). If there were some changes, Hibernate automatically generates UPDATE statements to propagate data to the database. This mechanism is called automatic dirty checking, with it, there is no need to explicitly call session save() or update() methods.
The problem is that for large entities comparing states each time may be time-consuming, also by default UPDATE() statements rewrite values for all fields which is also bad for performance.
One of the optimizations is to apply @DynamicUpdate
so that UPDATE statements will include only fields that have changes. It is also recommended to mark as @Immutable
immutable entities to turn off dirty checking for them.
Setting up a Hibernate project
17. How to make a project with Hibernate?
- Create a new project and add Hibernate dependency (Maven/Gradle)
- Add Hibernate parameters (with configuration file or in Code)
- Map Java Classes to database tables
- Create queries to work with data
- Process query results
- Show results in GUI/Console interface
18. What is a Configuration File?
A Configuration File defines pluggable strategies that Hibernate uses for various purposes: from database connection properties and mappings to the query settings and bytecode enhancement, the full list of possible configurations is pretty large.
The file is either hibernate.cfg.xml
for the full Hibernate functionality, or the persistence.xml
for JPA - complied functionality. The minimal configurations should include properties to connect to the database and list mapping classes or XML mapping templates:
- For desktop-based projects:
<property name="connection.url">
<property name="connection.username">
<property name="connection.password">
- For web applications (application server JNDI DataSource):
<property name="hibernate.connection.datasource">
- Mappings:
<mapping class = "full-class-name.Entity"/>
Hibernate also supports in-code configuration with org.hibernate.cfg.Configuration
object, but this method is semi-deprecated, and managing configurations in a separate file is easier, so I prefer to stick with the hibernate.cfg.xml
option.
19. Have you used the function addClass? Why or why not?
Function addClass()
was used with the Configuration
object to add a reference to the resource classes during the bootstrapping. Now, this is considered a Legacy Bootstrapping process, and in addition, I prefer storing all Hibernate settings in a separate configuration file.
20. What is Hibernate Connection Pool
Hibernate maintains services such as second connection pools, level caches, and transaction system integrations across all Sessions in the SessionFactories. The Connection Pool is a group of connection objects that are used and reused to run database connections on request, which reduces the overhead of opening a JDBC connection.
The good practice is to limit the number of connections in the connection pool so the database server is not overwhelmed by too many connections. For the built-in connection pool, the <property name="connection.pool_size">10</property>
property indicates the maximum number of connections. The catch here is to be careful and make sure the connections are closed and returned to the pool, leaking the connections will eventually starve the pool out and hang the application.
The built-in connection pool is not supported for use in a production system, instead, Hibernate supports integration with such mechanisms as c3p0, Hikari, or Proxool.
21. Does Hibernate allow you to connect multiple databases in one Java application?
Yes, each of the database connections will need a separate configuration file and separate SessionFactory
. For example, mysql-hibernate.cfg.xml
and postgres-hibernate.cfg.xml
, both specified in configure(String resourceName)
method of the StandardServiceRegistryBuilder
.
22. What are the three key interfaces offered by Hibernate?
The session, SessionFactory, and Transaction are three key interfaces offered by Hibernate.
SessionFactory (org.hibernate.SessionFactory
) is a thread-safe heavy object that contains Hibernate configurations and allows to create sessions with the database. Working with multiple databases will require multiple SessionFactory objects.
Session (org.hibernate.Session
) object is created by SessionFactory. It represents a physical connection to a database, as the java.sql.Connection
JDBC is hidden underneath. Additionally, Session holds the persistence context of the application domain model (first level cache).
Transaction (org.hibernate.Transaction
) object is created by Session. It is a single-threaded, short-lived object optionally used to set physical transaction boundaries. A Transaction provides the data it takes from the Java application to the database as a Query, and it should be removed after it is completed.
23. Is Hibernate Factory a thread-safe object?
Yes, SessionFactory is a thread-safe and immutable object. As this is a heavyweight object, it is usually created during application start-up and is kept for later use.
24. Is Hibernate Session a thread-safe object?
No, Session is not a thread-safe object, many threads can't access it simultaneously. It is designed to be a single-threaded and short-lived object that conceptually models a "Unit of Work" with the database.
The Hibernate has configuration option <property name="hibernate.current_session_context_class">thread</property>
defines strategy (thread/JTA/managed or a custom CurrentSessionContext
class) for the scoping of the current session. Value thread
(ThreadLocalSessionContext
) for this property applyes one session - one database transaction programming model.
25. What are differences between openSession and getCurrentSession?
SessionFactory.openSession()
method always opens a new session that should be closed once the operations are finished.
SessionFactory.getCurrentSession()
method obtains the current session, an instance of Session implicitly associated with some context defined in the hibernate.current_session_context_class
Hibernate configuration property. The current session automatically flushes and closes when the transaction ends and in a single-threaded environment can be a faster option than an openSession() method.
26. How is a Transaction committed/rolledback in Hibernate?
Hibernate has a Transaction interface that defines the unit of work. A transaction is associated with a Session and is usually instantiated by a call to Session.beginTransaction()
. Transaction.commit()
flushes the associated Session and ends the unit of work, Transaction.rollback()
forces this transaction to roll back.
27. What is the difference between Flush() and Commit()?
commit()
method will make data stored in the database permanent. There is no way you can roll back the transaction once the commit() succeeds.
flush()
method synchronizes the state of the persistence context with the underlying database, which is useful to send small chunks of data to the database to make sure the persistent context does not run out of memory. Flushed changes can be rolled back.
The flush mode of the current session defines when the flush operation will happen: by default, AUTO
flush mode flushes the session only if necessary, there are also modes to ALWAYS
flush the session before every query, delay the flush until COMMIT
or to explicitly call flush in MANUAL
mode.
Mapping
28. Can you list down important annotations used for Hibernate mapping?
@Entity
- marks a class as entity beans, each entity instance corresponds to the database table row.@Table
- used with entity beans to define the corresponding table name in the database, for example, name or unique constraints. Additional tables can be specified with the@SecondaryTables
annotation.@Column
- defines the properties of database table columns.@Id
- declares the entity field as the primary key of the database table. For auto-generated id values, the@GeneratedValue
annotation can be applied in conjunction with the @Id annotation, with optional parameters to specify the primary key generator and primary key generation strategy.@OneToMany
,@ManyToOne
,@OneToOne
, and@ManyToMany
to specify association rules.@Transient
- marks the field as not persistent.- JPA
@Cacheable
or Hibernate@Cache
specify that entity should be cached.
Hibernate uses JPA annotations and its own annotations, most of which has a set of optional parameters, the good news is that the Hibernate has a detailed user guide and a well-commented open-source code to find references whenever needed.
29. How many types of association mapping are possible in Hibernate?
Association mappings model a relationship between two database tables as attributes in the application domain model. Hibernate supports @OneToMany
, @ManyToOne
, @OneToOne
, and @ManyToMany
associations used in the database modeling, a total of four types.
In addition to that, Hibernate also provides @Any
and @NotFound
annotations for special cases of polymorphic associations or when foreign keys at the database level are not enforced.
30. What are different collection types available in Hibernate?
Hibernate supports mapping collections (java.util.Collection
and java.util.Map
) in a variety of ways, including the @ManyToOne
and @ManyToMany
associations.
In general, Hibernate supports the following ways to handle the collection:
- ARRAY
- BAG (may contain duplicate entries and has no defined ordering), ID_BAG
- LIST (the ordering of a list is by default not maintained)
- SET, ORDERED_SET (ordered by a SQL), SORTED_SET (sorted according to a Java Comparator)
- MAP, ORDERED_MAP, SORTED_MAP
By default, Hibernate interprets the defined type of the collection and automatically classifies it into one of these groups, for example, the Java array is in the ARRAY group, andjava.util.Set
is SET in Hibernate.
In addition, Hibernate provides its implementations of the Java collection types. They wrap an underlying collection and provide support for things like lazy loading, for example, PersistentArrayHolder
, PersistentBag
, and PersistentList
.
31. Can you explain derived properties?
By definition, derived properties are properties whose default value is calculated from a defined expression. And Hibernate extends JPA functionality by providing automatic mapping of any database computed value as a virtual read-only column with @Formula
annotation.
For example, @Formula(value = "monthlyPayment * 12"). In the current version of Hibernate, @Formula
annotation accepts only native SQL, which should be considered regarding database transferability.
32. Do you know whether Hibernate supports polymorphism?
Yes, Hibernate supports polymorphism. With Polymorphic queries, Hibernate will fetch all subclasses belonging to the base entity type. For example, a query for base class Person will fetch its subclasses, Student and Teacher. This behavior can be changed with the @Polymorphism(type = PolymorphismType.EXPLICIT)
annotation on the subclass, which instructs Hibernate to skip this subclass in the polymorphic query unless explicitly called.
For modeling polymorphic associations, Hibernate supports different inheritance mapping strategies: mapped superclass, table per class, single table, and joined strategies. In addition, there are Hibernate-specific @Any
and @ManyToAny
annotations that define a polymorphic association to classes from multiple tables.
33. What inheritance mapping strategies are available in Hibernate?
Although relational database systems don’t provide support for inheritance, Hibernate provides several strategies to leverage this object-oriented trait onto domain model entities:
@MappedSuperclass
- inheritance is implemented in the domain model only without reflecting it in the database schema@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
(default strategy) - all subclasses are mapped to only one database table@Inheritance(strategy = InheritanceType.JOINED)
- each subclass can is mapped to its own table, also called table-per-subclass.@Inheritance(strategy = InheritanceType.TABLE_PER_CLASS)
- map only the concrete classes of an inheritance hierarchy to tables, also called table-per-concrete-class strategy
Database Queries
34. How many ways can an object be fetched from Hibernate’s database?
There are three different ways to fetch objects from Hibernate’s database:
- Criteria API
- HQL
- Standard (Native) SQL
The result and performance of all approaches are similar in the latest versions of the Hibernate, the difference is in the syntax - what the developer is most experienced and comfortable with.
And, if the task is to fetch an entity by id, the Hibernate can perform a direct entity fetch with the session.find()
method.
35. Tell me about the Criteria in Hibernate
Criteria queries are a programmatic, type-safe way to express a query. Essentially criteria is an object graph, where each part of the graph represents an increasing more atomic part of the query.
The first step with criteria queries is building a graph with jakarta.persistence.criteria.CriteriaBuilder
. The next step is to obtain a jakarta.persistence.criteria.CriteriaQuery
, where the desired criteria query is constructed by navigating from the root and adding restrictions. CriteriaQuery is then used as a parameter for the createQuery()
method similar to the String with HQL or SQL request.
Example of Criteria:
// Get an instance of CriteriaBuilder from the session or entity manager
CriteriaBuilder builder = entityManager.getCriteriaBuilder();
// Construcn a new criteria query
CriteriaQuery<Entity> criteria = builder.createQuery(Entity.class);
// A root type in the from clause
Root<Entity> root = criteria.from(Entity.class);
// Specify the items that is to be returned in the query result
criteria.select(root);
criteria.where(builder.equal(root.get("name"), "Name123"));
// Optionally - select fields (columns) to be fetched - id and name
Selection[] selection = {root.get("id"), root.get("name")};
criteria.select(builder.construct(Entity.class, selection));
// Run query
List<Person> entities = entityManager.createQuery(criteria).getResultList();
Note: with a combination of Selection and Criteria it is possible to fetch only chosen fields from the database and improve the performance of the requests, especially if the database stores heavy data types.
36. What are the metamodel classes?
In criteria queries or a dynamic entity graph, the entity classes and their attributes can be referenced with Strings, for example, a.get("firstName")
.
The metamodel classes provide a type-safe option to refer to entity classes, for example, a.get(Author_.firstName)
. The metamodel classes are usually automatically generated by the Metamodel Generators like hibernate-jpamodelgen
.
37. Tell me about HQL in Hibernate
Hibernate Query Language (HQL) and its subset Jakarta Persistence Query Language (JPQL) are object-oriented query languages based on SQL that provide an abstraction from the database language.
Example of HQL:
List<Person> persons = session.createQuery(
"from Person", Person.class) // Person refers to Java class Person
.getResultList();
38. Explain about Query interface in Hibernate?
To execute a query, an instance of the jakarta.persistence.Query
interface is required. This interface is inherited by the jakarta.persistence.TypedQuery<X>
interface that controls the execution of the TypedQueries, which in turn is inherited by the org.hibernate.query.Query<R>
Hibernate interface that represents a criteria query or a query written in HQL. These Query contracts mix the ability to perform selections as well mutations.
Session has multiple methods to create queries: createQuery()
, createNamedQuery()
, createMutationQuery()
etc.
39. What are Named queries in Hibernate?
A query may be provided to Hibernate as either an inline query when the text of the query is passed as a string to the session at runtime, or as a named query, where the query is specified in an annotation or XML file, and identified by name at runtime.
// Add named queries as annotations for Entity Classes
@Entity
@NamedQuery(
name = "get_entity_by_name",
query = "select e from Entity e where name like :name"
)
public class Entity {...}
// Use name queries in other places
Query query = session.createNamedQuery("get_entity_by_name", Entity.class);
query.setParameter("name", "%"+"a"+"%");
List<Entity> entities = query.getResultList();
Named queries allow to group query statements at a single location in the project and call them by names when needed, thus making code cleaner to read.
40. Can you execute native SQL in Hibernate?
Yes, Hibernate also allows specifying manually written SQL (including stored procedures) for all create, update, delete, and retrieve operations. This helps to utilize database-specific features or to simplify migration from the JDBC project.
Execution of native SQL queries is controlled via the NativeQuery
interface, which is obtained by calling the Session.createNativeQuery()
method.
// Run SQL query and fetch only id and name columns from the database
List<Object[]> persons = session.createNativeQuery(
"SELECT id, name FROM Person") // Person refers to database table
.list();
41. Explain the difference between get() and load() methods.
Both Session.get()
and Session.load()
methods fetch entity from the database by the id
.
The difference is that the get()
method returns an instance of an entity, or a null if there is no persistent instance with the given id. If the instance is already associated with the session, it returns that instance. And the load()
(or getReference()
since Hibernate 6) method returns only a reference to the persistent instance, the actual initialization is done on demand - when a non-id method is accessed. So, getReference()
is faster because of lazy loading, but the object should exist in the database when the proxy entity is initialized.
42. Explain the difference between update() and merge() methods.
Both session.update()
and session.merge()
methods are used to change the state of the detached entity instance and return it in current persistence context.
The difference is that the update()
method throws an error if the persistence context already has an entity with the same id, and the merge() method copies the state of the given object onto the persistent object with the same identifier. It is recommended to use the merge()
method, as in the newest versions of Hibernate update()
is deprecated.
43. What is difference between save(), saveOrUpdate() and persist() methods?
Historically Hibernate had different methods to add transient entities to the persistent context of the session, for example, the save()
method is used to persist a transient instance by first assigning a generated id. Whether this operation cascades on the mapping entities depends on the CascadeType annotation.
Similar to save()
, saveOrUpdate()
method either save()
or update()
instance depending if the data is present in the database. Since Hibernate 6 use of all these methods is deprecated, so it is a good practice to use persist()
and merge()
methods instead.
session.persist()
method also makes a transient instance persistent, however, it can generate an id value later, at a flush time, and should be called inside the transaction to save changes to the database.
Performance
44. Tell me what you know about Hibernate caching.
As an intermediary between application and database that is responsible for managing database queries, Hibernate ORM includes flexible support for caching: there are build-in caching tools as well as API for third-parties caching products.
First level cache - is automatically supported by the hibernate cache also called persistence context that is associated with the session object and thus can not be accessed after the session is closed.
Second level cache - is an optional cache bound to the SessionFactory life-cycle, thus allowing to share cache between session objects. By default second-level cache is turned off, to start the second-level caching the Hibernate needs to connect with the caching provider that supports API defined in JSR 107: JCACHE - Java Temporary Caching API Specification, or, in earlier versions of Hibernate, to directly connect with Ehcache.
45. What is Query cache in Hibernate?
Hibernate can cache entities and collections of entities. Aside from that, Hibernate can cache results of frequently executed queries with fixed parameter values, but this is disabled by default because of an overheard to keep track when query results become invalidated. Query caching is enabled with the property hibernate.cache.use_query_cache
in the configuration file and requires a second-level cache. To make query cacheable, both to save to cache and search in the cache, the Query.setCacheable(true)
method is used.
46. Explain concurrency strategies.
A concurrency strategy is a mediator, which is responsible for storing items of data in the cache and retrieving them from the cache.
Hibernate defines the following cache concurrency strategies to use with the @Cache
annotation:
- READ_ONLY - to cache reference data that is never updated
- READ_WRITE - caches data that is sometimes updated while maintaining the semantics of the "read committed" isolation level, and uses soft locks on the cache.
- NONSTRICT_READ_WRITE - caches data that is sometimes updated without ever locking the cache, thus there are time windows after changes and before commits when stale data can be obtained from the cache.
- TRANSACTIONAL - a "synchronous" concurrency strategy that commits changes to both cache and database in the same transaction.
47. What is lazy loading in Hibernate?
Hibernate fetches data from the database either in eager or lazy mode. The first fetches the data immediately, and the latter fetches data only on demand. By default, associations @OneToOne
and @ManyToOne
use FetchType.EAGER
and @OneToMany
and @ManyToMany
use FetchType.Lazy
loading. With lazy loading, instead of the object, a proxy is created, which is an object that doesn't contain all of the data needed but knows how to get it.
Lazy loading saves the cost of preloading/prefilling all the entities in a large dataset beforehand while there is a high chance that not all entities will be needed. The default behavior can be adjusted by changing FetchType
, but with the EAGER
loading one should be especially careful with the n+1 problem.
48. What is Hibernate Proxy?
Hibernate uses generated proxy classes to support lazy loading. The proxy class is a subclass of the entity class that implements the HibernateProxy
interface. Since version 5.3, Hibernate uses Byte Buddy to generate proxies at runtime.
On accessing one of the proxy fields or methods Hibernate generates a SELECT statement to load an entity from the database. There is also a static Hibernate.unproxy()
method to convert proxy to a Real Entity Object.
49. What is the Hibernate n+1 problem?
The n+1 problem means that the Hibernate generates 1 query to fetch a parent object, and n queries to fetch all associated objects. The larger the value of N, the more queries will be executed, and the larger the performance impact.
The possible solutions are applying the JOIN FETCH clause to queries or, more flexible, applying a Dynamic Entity Graph.
50. How can you log SQL queries executed by Hibernate?
The first option to show SQL queries is to set up <property name="hibernate.show_sql">true</property>
in the configuration file, so Hibernate automatically prints all queries to the terminal. The problem with this approach is that Hibernate does not show the parameters passed to the queries.
The second option is more work-consuming at the start but pays off in the long run - setting up the logging settings. There are different loggers like Apache Log4j 2 that can automatically catch Hibernate messages on different levels(DEBUG/ERROR/INFO etc.) and for different classes and print it to the console or log file. To show SQL statements, for example, org.hibernate.SQL
should be logged, and the binding parameters are shown in the org.hibernate.orm.jdbc.bind
.
51. Is there a way to reduce getters and setters?
Hibernate requires that entity classes must have getters and setters methods for each of the fields, combined with constructors this code takes a lot of place in Java class and becomes hard to read. Such template-like code can be hidden behind annotations, for example, the Lombok project provides annotations @Getter
, @Setter
, @NoArgConstructor
and works well with Hibernate.
52. Define Hibernate’s validator framework
Hibernate Validator framework allows to express and validate application constraints. For example, adding the @NotNull
constraint will make sure that the field has value, and @Size(min = 2, max = 14)
will check the length of the String.
In general, data validation is an essential part of any application, but the question is on what layer to validate data to apply minimum repeating validation operations. The idea behind Hibernate's Validator framework is to bundle validation logic directly into the domain model, cluttering domain classes with validation code which is metadata about the class itself.
The Validator is not a part of the Hibernate core and should be set up independently.
53. What are the design patterns used in Hibernate?
Design Patterns denote the best computer programming practices in object-oriented software development, the Hibernate framework applies a variety of design patterns:
- Domain Model Pattern - an object model of the domain that incorporates both data and behavior, which can be seen in Entity beans.
- Proxy Design Pattern - provides a surrogate or placeholder for another object to control access to it, applied for lazy loading
- Factory Design Pattern - defines an interface for creating an object, but lets subclasses decide which class to instantiate, for example, SessionFactory is an interface that is implemented by
SessionFactoryDelegatingImpl Class
, but can also be implemented by a custom class. - Query Object Pattern - a database query is represented by an object, as in Hibernate Criteria API and the modern JPA2 Criteria API.
- Data Mapper Pattern - a layer of Mappers that moves data between objects and a database while keeping them independent of each other and the mapper itself
- Unit of Work - transaction object represents a single unit of work with the database
54. Is Hibernate prone to SQL injection attacks?
Hibernate does not grant immunity to SQL Injection, one can misuse the API as they please, and HQL in this term is not very different from native SQL.
However, some of the good practices help to avoid SQL injection attacks:
- All communications with the database should occure inside a database transaction - between methods like
Session.beginTransaction()
andTransaction.commit()
. - The parameters should not be directly passed to the queries, but with the
query.setParameter()
method instead, this is called Parameterized Queries. - Apply input validation.
- If an error happens during the session, the catch block should have
rollback()
for the transaction.
55. What are some Hibernate framework best practices you should follow?
The Hibernate is easy to start with but has a lot of advanced features and practices to learn to improve the performance and robustness of the application. Some of the best practices to follow that I know about are:
- Avoid deprecated methods, as there might be security and maintenance issues in the future.
- Log and analyze all queries during development
- Prefer Data Access Object design pattern for exposing the different methods that can be used with entities.
- Specify natural identifier in addition to the surrogate key, for example, ISBN and id for Book.
- Check the primary key field access, if it’s generated at the database layer then you should not be able to change it at the application layer.
- Prefer lazy fetching for associations.
- Use native SQL only when the specific database features are required, in other cases abstract from the database dialect with HQL or criteria queries.
- Use JPA Metamodel when working with Criteria API
- Use Parameterized Queries
- Organize named queries and consolidate them in a single place for easy debugging, if they are entity-specific, they can be stored in the Entity Class as static Strings.
- Avoid rare association mappings
@ManyToMany
, or unidirectional@OneToMany
associations, especially ifMany
assumes large numbers. - For collections, try to avoid arrays as Hibernate does not support lazy-loading for arrays, and mappings as a
@ElementCollection
are not very efficient. - If you have to sort the collection, consider ordering it on the database layer rather than sorting it using Collection API
- If an error happens during the session, the catch block should have rollback() for the transaction.
- If a specific entity is immutable, it is good practice to mark it with the
@Immutable
annotation to turn off dirty checking. - Limit the number of connections in the connection pool so the database server is not overwhelmed by too many connections
- Use SQL scripts to create the database schema, not the Hibernate autogenerated schema.
- Hibernate can access field values either directly or via getters and setters. The access strategy for the entity is defined by the placement of the
@Id
annotation - either before the id field or before the id getter. So, if you want the Hibernate to use setters (for example, they have a specific behavior defined), then place@Id
before the getter method.
When it comes to performance optimization of the Hibernate application, the strategies are applying data caching, SQL optimization, and Session management.
Multiple choice questions
1. What inheritance mapping strategy is not present in JPA?
2. Can you use JOINS in Hibernate?
3. What object is required to create SessionFactory in Hibernate?
4. What design pattern is applied for Hibernate lazy loading?
5. What method returns connection from session?
6. What level of transaction isolation is the highest?
7. What annotation should be used for a Class that is a part of another Entity Class?
8. Which among the below is held by the Session object?
9. Which cascading types are not supported by Hibernate?
10. Some Hibernate projects use Class_.java files in addition to Class.java, what are they?
Each of the questions is provided with a concise explanation aimed at Hibernate 6 and also touched on ORM and JPA theory. Additional topics to consider before an interview are the integration of Hibernate with other popular frameworks like Spring. Note that the best way to shine in the interview is to supply theoretical answers with the mentioning of your experience on how you applied that features in real projects.
With this article at OpenGenus, you must have good practice with Interview questions on Hibernate.