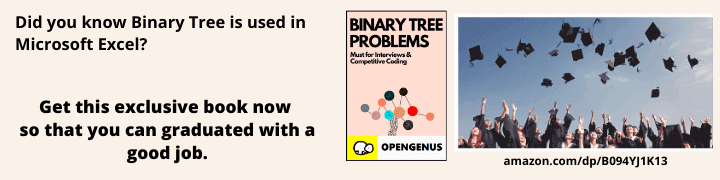
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Queue is a data structure that is considered first in first out (FIFO). This means that the element who comes to the queue first can be can get out of the queue first. Before we get to the Israeli Queue, we will briefly introduce the Priority Queue first. In this variation data structure for the queue, every element in it has a priority, and the one with the highest priority will be dequeued first.
The Israeli Queue is also a variation for the priority queue because elements in it also have priority. However, the priority is determined by their "friendship" with a friend. To understand the Israeli Queue concepts, we will explore its role in maintaining fairness and optimizing resource utilization.
-
Table of Contents
- The Need for Fair Resource Allocation
- Israeli Queue Algorithm
- Benefits for Israeli Queue Algorithm
- Implementations and Adaptations
- Real-World Application
- Conclusion
-
The Need for Fair Resource Allocation
In shared environments, such as computer networks or data centers, resources like bandwidth or processing power are limited and need to be efficiently distributed among multiple participants. Without a fair allocation mechanism, certain participants may monopolize resources, leading to suboptimal performance and potential resource starvation. The Israeli Queue addresses this issue by ensuring that each participant has an equal opportunity to access the shared resource, regardless of their priority or position in the queue.
-
Israeli Queue Algorithm
The Israeli Queue algorithm follows a simple yet effective mechanism. Participants are assigned priorities or tokens, which determine their position in the queue. The participant at the head of the queue, possessing the highest priority or token, is granted access to the resource for a predetermined time period. After this period elapses, the participant relinquishes the resource and moves to the end of the queue, allowing the next participant in line to access the resource. This process continues, cyclically allocating the resource to each participant in a round-robin fashion.
-
Common Operations and their time/space complexity
-
Enqueue: Adding an element to the end of the queue.
Time complexity: O(1) - The enqueue operation in an Israeli queue is typically a constant-time operation since it involves simply joining the queue behind the last person.
Space complexity: O(1) - The space complexity remains constant as no additional data structure is required to perform the enqueue operation. -
Dequeue: Removing the element at the front of the queue.
Time complexity: O(1) - Similar to enqueue, the dequeue operation in an Israeli queue is typically a constant-time operation. Since participants in the queue maintain their position, removing the front element involves the person at the front moving forward.
Space complexity: O(1) - The space complexity remains constant as no additional data structure is required to perform the dequeue operation. -
Size: Determining the number of elements in the queue.
Time complexity: O(n) - To determine the size of the Israeli queue, you need to traverse the entire queue and count the number of participants waiting. Therefore, the time complexity is linear and proportional to the number of participants in the queue.
Space complexity: O(1) - The space complexity remains constant as no additional data structure is required to determine the size.
-
Benefits for Israeli Queue Algorithm
The Israeli Queue offers several benefits and advantages in resource allocation scenarios. Firstly, it ensures fairness by providing equal opportunities for all participants. No participant is favored over others, preventing resource starvation and promoting a more equitable distribution. Secondly, the algorithm avoids monopolization, as participants are obliged to relinquish the resource after their allocated time, allowing others to access it. This promotes resource sharing and prevents a single entity from dominating the resource for an extended period. Additionally, the Israeli Queue is relatively simple to implement and does not require complex calculations or extensive memory usage, making it efficient and suitable for real-time systems.
-
Implementations and Adaptations
The Israeli Queue algorithm has been implemented in various contexts, including computer networks, data centers, and shared processing environments. Depending on the specific requirements and characteristics of the system, adaptations and modifications of the algorithm have been developed. For instance, the token-based priority mechanism can be tailored to allocate resources based on factors such as bandwidth availability, processing power, or fairness metrics specific to the application domain. Such adaptations enable fine-tuning of the algorithm to suit the needs of different environments.
The following is an example of the implementation from which I got the idea from the link here
class IsraeliQueue(list):
def put(self, item, friend):
if friend not in self:
raise ValueError("Friend not found")
friend_indices = [(index, f) for index, f in enumerate(self) if f.group == item.group]
if not friend_indices:
raise ValueError("No friends found in the same group")
max_index, _ = max(friend_indices, key=lambda f: f[0])
self.insert(max_index, item)
def enqueue(self, a):
for i in range(len(self)):
if type(self[i][0]) == type(a):
self[i].append(a)
break
else:
self.append([a])
def dequeue(self):
return self.pop(0)
-
Real-World Application
The Israeli Queue has found practical applications in numerous fields.
-
Bandwidth Allocation in Computer Networks:
In computer networks, the Israeli Queue algorithm has been widely applied for fair bandwidth allocation among users. By implementing the Israeli Queue, network administrators can ensure that each user receives an equitable share of the available bandwidth. This prevents any single user from monopolizing the network resources, leading to a better overall network performance and user experience. -
Resource Management in Data Centers:
Data centers, where multiple virtual machines or containers coexist, require efficient resource management to ensure optimal performance. The Israeli Queue algorithm can be employed to allocate CPU, memory, and storage resources fairly among the virtual entities. By implementing the Israeli Queue, data center administrators can prevent resource monopolization and effectively balance resource utilization across different workloads. -
Distributed Computing Systems:
Distributed computing systems, where multiple nodes collaborate to perform complex tasks, require fair resource allocation to ensure efficient execution. The Israeli Queue algorithm can be used to manage task scheduling and resource allocation in these systems. By ensuring that each node receives a fair share of the available resources, the Israeli Queue enables collaborative computing without any node being disproportionately burdened. -
Traffic Management in Transportation Networks:
Transportation networks, such as traffic intersections or road networks, face challenges in managing the flow of vehicles to avoid congestion and ensure fair access. The Israeli Queue algorithm can be adapted to allocate green signal time at intersections fairly, considering factors such as traffic volume, waiting times, and priority levels of different lanes. By implementing the Israeli Queue, traffic managers can optimize traffic flow and reduce congestion by ensuring fair access to the road network. -
Task Scheduling in Cloud Computing Environments:
Cloud computing environments rely on efficient task scheduling algorithms to allocate computing resources among different user requests or applications. The Israeli Queue algorithm can be leveraged to allocate resources fairly among these requests. By considering factors such as priority levels, resource requirements, and fairness metrics, the Israeli Queue enables cloud providers to optimize resource utilization, meet service-level agreements, and ensure equitable access to computing resources. -
Content Distribution in Peer-to-Peer Networks:
Peer-to-peer (P2P) networks, where participants share files and resources, can benefit from the Israeli Queue algorithm for fair content distribution. By employing the Israeli Queue, the network can ensure that each participant has a fair opportunity to upload and download files, preventing certain peers from dominating the network and ensuring an equitable distribution of shared content.
-
Conclusion
In shared environments where resource allocation is a challenge, the Israeli Queue algorithm provides an effective solution to ensure fairness and prevent resource starvation. By cyclically allocating resources in a round-robin fashion, participants are granted equal opportunities to access shared resources, maintaining equilibrium and optimizing performance. The Israeli Queue's principles and adaptability make it a versatile algorithm applicable to various domains.