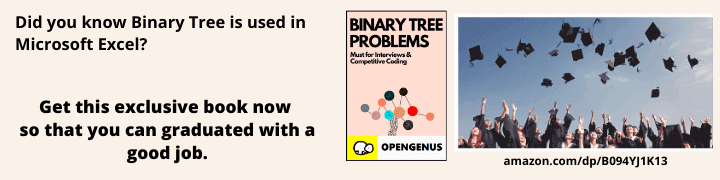
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Hello everyone in this article we are going to see how to parse a JSON response from Web API in iOS app using Swift Codable Protocol.
What is JSON
JSON stands for JavaScript Object Notation.It is an open source data interchange format. It uses human readable text to store and send data. It stores the data in key value pair similar to a Dictionary data structure.
What we are going to build
We are going to build an iOS app which uses Quotes API (type.fit/api/quotes
) which returns around 1000 quotes from the web server in JSON format.
Examining API response
- Open up the Mac terminal and run the following command
curl https://type.fit/api/quotes
This command is known as client URL which can be used to do some networking stuff through terminal here in this case we perform a HTTP GET request and the web server returns a JSON response.
Here in the response as you can see we get an array of JSON Objects from the server and each object has a text and author key along with their values.
Parsing JSON in Swift using Codable
- Open up Xcode and create a blank Xcode project
- Create a blank swift file and add the below code in it.
import Foundation
struct Quote: Codable {
let text: String?
let author: String?
}
typealias Quotes = [Quote]
Here we create a struct which act as model based on which we parse the JSON response.
- Create another swift file and add the below code
import Foundation
class NetworkService
{
static var sharedObj = NetworkService()
let QUOTES_URL = "https://type.fit/api/quotes"
let session = URLSession.init(configuration: .default)
func getQuotes(onSucess: @escaping(Quotes) -> Void)
{
let task = session.dataTask(with: URLRequest(url: URL(string: QUOTES_URL)!)) { (data, respone, error) in
DispatchQueue.main.async {
if let d = data
{
do
{
let decodeddata = try JSONDecoder().decode(Quotes.self, from: d)
print(decodeddata.count)
onSucess(decodeddata)
}
catch{
print(error.localizedDescription)
}
}
}
}
task.resume()
}
}
Here we create a Singleton class named NetWorkService which makes HTTP GET request to the webserver and fetch the JSON file,Parse the JSON file.Here we use a trailing closure to send the parsed response as a parameter to the call back function.
- Open Main.stroyboard and create an UI similar to the below design with a navigation controller,table view and a detail view controller.
- Open up the ViewController.swift file and add the following code.
import UIKit
class ViewController: UIViewController,UITableViewDelegate,UITableViewDataSource {
var selectedQuote: Quote!
@IBOutlet weak var quotesTV: UITableView!
var QuoteArray = [Quote]()
override func viewDidLoad() {
super.viewDidLoad()
quotesTV.delegate = self
quotesTV.dataSource = self
navigationController?.navigationBar.prefersLargeTitles = true
title = "Quotes"
NetworkService.sharedObj.getQuotes { (Quotes) in
self.QuoteArray = Quotes
self.quotesTV.reloadData()
}
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return QuoteArray.count - 1600
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
if let cell = tableView.dequeueReusableCell(withIdentifier: "cell")
{
cell.textLabel?.text = QuoteArray[indexPath.row].author
cell.detailTextLabel?.text = QuoteArray[indexPath.row].text
return cell
}
return UITableViewCell()
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
selectedQuote = QuoteArray[indexPath.row]
performSegue(withIdentifier: "segue", sender: self)
}
func tableView(_ tableView: UITableView, commit editingStyle: UITableViewCell.EditingStyle, forRowAt indexPath: IndexPath) {
if editingStyle == .delete
{
self.QuoteArray.remove(at: indexPath.row)
self.quotesTV.deleteRows(at: [indexPath], with: .fade)
}
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if let destinationVC = segue.destination as? QuoteVC
{
destinationVC.quote = selectedQuote
}
}
}
Here we create an IBOutlet for the table view and populate the table view with the parsed JSON response when the user selects a table view row then it will make a transition to detail view controller and display the quote along with the author name.
- Create another Swift file with base class of UIViewController and add the following code.
Whenever the user taps a row in the table view we pass the entire Quote model object to detail view controller and disply it in the label of the DetailViewController.
import UIKit
class QuoteVC: UIViewController {
var quote: Quote!
@IBOutlet weak var quotetxtview: UITextView!
@IBOutlet weak var authorlbl: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
quotetxtview.text = quote.text
authorlbl.text = "By \(quote.author ?? "No Author")"
// Do any additional setup after loading the view.
}
}
Now build and run the project in the simulator make sure you have connected your system to internet.When the app is launched it makes an HTTP GET request to web server which returns a JSON response which is parsed and displayed in the table view of the view controller.
Now when the user taps on the table view row it makes transition to next detail view controller and display the quote along with the author name.