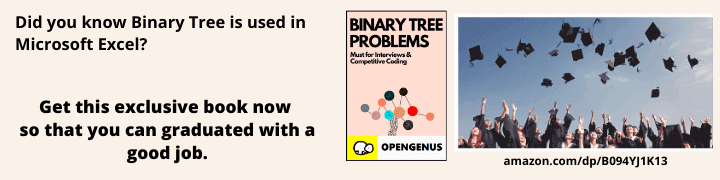
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the concept of the OpenMP directive #
pragma omp parallel along with C++ code examples.
Table of contents:
- pragma omp parallel
- When to use omp parallel?
Pre-requisite:
- Understand how to use OpenMP to parallelize C++ code from the basics.
pragma omp parallel
By default, one thread is assigned to a program during execution which is known as master thread.
Team is a group of threads available to the program execution. Initially, it has one thread that is the master thread. At the end of the execution, the program will have one thread that is the same master thread.
In between, master thread can be split into multiple threads which can be used within the program by different code segments to run in parallel.
This process of increasing the number of threads in Team is done when this OpenMP directive is defined.
#pragma omp parallel
Following is a C++ code example using pragma omp parallel:
#include <stdio.h>
int main() {
#pragma omp parallel
{
int x = 1;
int y = x + 2;
printf("Hello\n");
}
return 0;
}
Compile and run this C++ code as follows:
gcc -o code code.cpp -fopenmp
./code
When to use omp parallel?
One should use this OpenMP directive as follows:
- Add this OpenMP directive "pragma omp parallel" in your program to create a new team of threads. If this is not used, then there will be only one thread (master thread) so other OpenMP directives will not work.
- If you are not an expert, enclose your code with pragma omp parallel and OpenMP will distribute the work across multiple threads accordingly.
pragma omp parallel
{
// this code snippet will
// run in parallel ...
}
- On several occasion, this will create race condition within the code as OpenMP is not aware of the logic within the code. This may result in wrong output and slower execution. To fix this, use other OpenMP directives in addition to this like:
#pragma omp for
#pragma omp critical
In short, pragma omp parallel is the most basic way to parallelize a code. With this article at OpenGenus, you must have the complete idea of "pragma omp parallel".