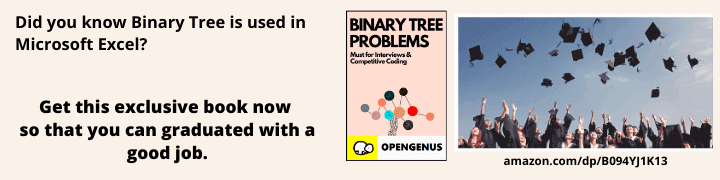
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 10 minutes
In this article, we will go through the basics of shell scripting, understand how to take input and produce output, working with variable, some basic arithmetic and other concepts.
We will cover the following topics:
- Hello World Code
- Variable Declaration
- Taking input from User
- Standard Input and Output example
- Unsetting variables
- Special Variables
- Command line arguments in Shell
- Basic Arithmetic Operation
Shell Scripting comprises of two terms:
Shell: In Linux OS, Shell is the interface between CPU kernel and Linux Actor (Users like humans, machine etc.)
Linux Shell provides interface via a command line with $ prompt for secondary users and # prompt for root or primary users.
Note: Shell is an interpreter, thus if we specify a set of codes in shell, it will execute all of them one by one even if an intermediate command gives an error/exception.
Linux shell can be of following types:
- Bourne Shell (sh)
- Bourne Again Shell (bash)
- Korn Shell (ksh)
- Turbo Shell (tcsh)
- C-Shell (csh) etc.
Script: A script in general is a set of code that automatically executes.
Scripts can be invoked in following ways:
- using triggers
- using schedulers like Crontab, Anacron, at etc.
- Manual Invocation
Some of the most popular scripting languages are Python, JavaScript and Shell Scripts.
Shell Scripts: Shell scripts are set of codes written in Linux shell to perform following tasks:
- Automate the workflow
- Perform batch tasks
- Perform repetitive tasks
- Schedule the tasks using Schedulers like Cron etc.
General Workflow for creating and executing a Shell Script
- Create a shell script using one of the text editors in Linux like Gedit, nano, vi etc.
Here, we use Gedit text editor.
Open the Shell by searching it or by using shortcut keys leftctrl+alt+T.
Type the following:
gedit <filename>.sh
Choose a filename with extension .sh for shell scripts. The Shell transfers control to Gedit tool that opens up filename.sh file.
- Write the code for the current shell script.
Note: The shell scripts begin with the following command:
#!/bin/bash
It has following significance:
- # symbol acts as the pre-processor directive to indicate the compiler that shell code starts with it.
- ! symbol specifies compiler to include the following specified file in the shell.
- /bin/bash is the location of the bash which is used for compiling the shell script.
- Exit the text editor and return to the Shell.
- Provide the shell script with executable permission using following command.
chmod +x {filename}.sh
Here,
- chmod is a linux keyword for changing the file permissions.
- +x Makes the specified file executable.
- Execute the shell script using the following command:
./{filename.sh}
1. Hello World Code
- echo keyword is used to print the standard output to the shell terminal.
echo "{message}"
- echo command includes an inbuilt default linefeed at the end.
- Note: Shell does not require the usage of ; at end of each instruction if we specify different instructions in separate lines but it is a good practise to specify ; as it makes it easier to debug the code later and makes it more understandable.
Code:
#!/bin/bash echo "Hello World";
Output:
Here,
- When the script was executed without being made into an executable using the chmod command, Bash gave an error specifying permission denied.
Thus, it is necessary to make the Shell Script executable. - cat is a Linux command that is used to display the content of files.
cat {filename}.{ext}
2. Variable Declaration
Syntax:
{variablename}={variable value}
- There must be no spacing between variable name, assignment symbol (=) and variable value else bash would not recognize the instruction.
- Variable name may include: Alphabets (a-z),(A-Z), underscore
(_)
and digits (0-9) but they cannot start with a numeric digit. - Variable name cannot contain any special character like
*
,!
etc. as they have special meaning in Linux. - Variable name cannot be a defined keyword in Linux like chmod, sudo etc.
- Variables in Shell Scripting are not data types as in programming language but only act as a label to a specified value. They do not have a data type.
Fetching the value of the variable:
$variablename
$ symbol is used to access the value of the variable name.
Generally, the value assigned to a variable may be changed using the assignment operator which has same syntax as in variable declaration.
{variable name}={variable value}
Creating a readonly variable:
Readonly variables can be created using the readonly keyword before the variable declaration.
readonly {variable name}={variable value}
Their value,once defined, cannot be updated.
Code:
#!/bin/bash # creating a normal variable var1="Variable 1"; # No spacing between variable name and value else error echo $var1; # Changing value of variable 1 var1="Variable 1 updated"; echo $var1; # Creating readonly variable readonly var2="Readonly var"; echo $var2; var2="Can I change?"; echo $var2;
Output:
Here, the second last line of the output specifies an error condition because the code tried to update a readonly variable which is not allowed.
3. Taking input from User
Reading input from the users using the read keyword
read {variable name}
It reads a value from the standard input from the user until a readline is specified and assigns the value to the variable.
Reading input from user by giving a prompt:
read -p "prompt message" {variablename}
- -p tag is used to send a prompt message to the user.
- prompt message is specified in double quotes following the -p tag.
Reading silent input from the user:
read -s {variable name}
This is a secure input method where the user input does not show up on the display screen while entering the input.
Code:
#!/bin/bash # This is how you add comments # Reading variable without promptings users read var1 echo "$var1" # Reading variable by prompting user read -p "Enter a value: " var2 echo "$var2" # Reading a silent variable by prompting user read -sp "Enter a value: " var3 echo "" # By default it has an endline attached echo "$var3"
Output:
- We can add comments in Shell Script using
#
symbol.
# This is a comment and it will be ignored by bash interpreter.
- We can take a silent input from the user by using a prompt using following command:
read -sp "prompt message" {variable name}
4. Standard Input and Output example
Standard Input: Use the read keyword to take input from the user.
Standard Output: Use the echo keyword to display the output to the user.
Code:
#!/bin/bash echo "Hello $(whoami)! I am your assistant!" read -p "Enter your real name: " name echo "Hello $name"
- whoami is a Linux keyword having the machine name as the assigned value. Bash can access system variables like whoami using the $ symbol by placing inbuilt variables in braces ().
$(system variable)
Output:
5. Unsetting variables
Variables can be deleted from the memory using the unset keyword.
unset {variable name}
Note: We cannot delete the readonly variables.
Code:
#!/bin/bash var1="Variable to be deleted!"; echo "$var1"; unset var1; echo "Printing unset variable: $var1"; # Trying unset keyword on readonly readonly var2="Readonly var"; echo $var2; unset var2; echo $var2;
Output:
- Null value is printed if the unset variable is specified in output using echo.
- Unsetting a readonly variables gives an error in Standard Error as readonly variables cannot be deleted.
6. Special Variables
Linux defines some special values that can be accessed using the $
operator.
$0
File Name.${0-9}
Command line arguments$$
PID (Process Id) of Current Shell$!
PID of last background command$?
Exit ID of last command etc.
Code:
#!/bin/bash echo "Current File Name: $0" echo "Process number of current Shell: $$" echo "Process Number of last background command: $!" echo "Exit ID of last executed command: $?"
Output:
7. Command line arguments in Shell
Command line arguments are list of space separated arguments specified when the shell script is manually executed.
./{filename}.sh {argument1} "{argument 2 with spacing in between (needs double quotes)}" {argument3} ...
Shell treates the command line arguments using following special variables:
${0-9}
specifies the command line argument where
$0
is the filename of the current shell script$k
is the kth command line argument
$#
is the number of command line arguments passed.$*
specifies all the arguments as a single value.$@
specifies all the arguments in space separated list.
Code:
#!/bin/bash echo "Command Line Arguments" echo "File name: $0"; echo "First Argument: $1"; echo "Second Argument: $2"; echo "Number of Arguments sent: $#"; echo "All arguments in same quotes: $*"; echo "All arguments in individual quotes: $@";
Output:
8. Basic Arithmetic Operations
Basic mathematical operations can be done using following command:
Syntax:
$((value1{operation}value2))
or
$(($variable1{operation}$variable2))
Example:
echo "$((10+20))"
It will give result as 30.
Code:
#!/bin/bash echo "Arithmetic Operations on Shell"; read -p "Enter an Real value: " val1 read -p "Enter another Real value: " val2 echo "Addition: $(($val1+$val2))" echo "Subtraction: $(($val1-$val2))" echo "Multiplication: $(($val1*$val2))" div=$(($val1/$val2)) echo "Division: ${div}"
Note: Division operation specified above gives integer output only by ignoring the decimal part.
Example: 10/3 will give 3 rather than 3.3
Output: