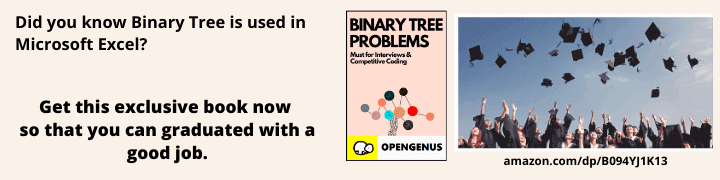
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 35 minutes | Coding time: 15 minutes
The Push API gives web applications the ability to receive messages pushed to them from a server, whether or not the web app is in the foreground, or even currently loaded, on a user agent. This lets developers deliver asynchronous notifications and updates to users that opt in, resulting in better engagement with timely new content.
Let's see some feature of Push API:
- The Push API allows a web application to communicate with a user agent asynchronously.
- An application server can send a push message at any time, even when a web application or user agent is inactive.
- For an app to receive push messages, it has to have an active service worker.
- The push service ensures reliable and efficient delivery to the user agent.
Components of Push API
- Application server: The term application server refers to server-side components of a web application.
- Push message: A push message is data sent to a web application from an application server.
- Push subscription: A push subscription is a message delivery context established between the user agent and the push service on behalf of a web application.
- Push service: The term push service refers to a system that allows application servers to send push messages to a web application. A push service serves the push endpoint or endpoints for the push subscriptions it serves.
- Permission : The term express permission refers to an act by the user, e.g. via user interface or host device platform features, via which the user approves the use of the Push API by the web application.
How push framework works?
A push message is sent from an application server to a web application as follows:
- The application server requests that the push service deliver a push message.This request uses the push endpoint included in the push subscription.
- The push service delivers the message to a specific user agent, identifying the push endpoint in the message.
- The user agent identifies the intended Service Worker and activates it as necessary, and delivers the push message to the Service Worker.
Below is the Diagram which shows how push API works:
Let's Understand the process:
- Check if user is Subscribed and update the UI accordingly
- If the user is not subscribed,prompt them to subsribe
- When user grants permission for push on client side,they are subscribed them to the browser push service.
- This creates special subscription object that contains an endpoint url for the push service which is different for each browser along with a public key.
- Now the object to sent to server and it is saved in the server.
- Next time when the user requests then theis object is checked and updated if there is need of any update.
Service Workers
Service worker is a client side programmable proxy between our app or website and outside world or server.
- It acts as a caching agent
- It is used to handle push messaging
- It is also used to store content for offline use.
Service worker registration
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('service-worker.js')
.then(registration => {
console.log('Service Worker is registered', registration);
})
.catch(err => {
console.error('Registration failed:', err);
});
});
}
- Add this code to your index html file or main javascript file.
- Create a new file service-worker.js
- First if check for the compatibility of service worker in your browser
- '.register' registers the service worker in the background.
U'll see something like this:-
Open the application tab which confirms that the service worker is registered:-
function askPermission() {
return new Promise(function(resolve, reject) {
const permissionResult = Notification.requestPermission(function(result) {
resolve(result);
});
if (permissionResult) {
permissionResult.then(resolve, reject);
}
})
.then(function(permissionResult) {
if (permissionResult !== 'granted') {
throw new Error('We weren\'t granted permission.');
}
});
}
In the above code, the important snippet of code is the call to Notification.requestPermission(). This method will display a prompt to the user:-
What if we block the permission,try to decline the permission and see the console:-
You can handle the denied permission by popping out dailog box or anything.
const title = 'Simple Title';
const options = {
body: 'Simple piece of body text.\nSecond line of body text :)'
};
registration.showNotification(title, options);
If we run the following script then we will see notification like this:-
Add this code to service-worker.js:
console.log("service-worker loaded");
self.addEventListener('push',e=>{
const title = 'Simple Title';
const options = {
body: 'Web push testing'
};
registration.showNotification(title, options);
});
U will see something like this: