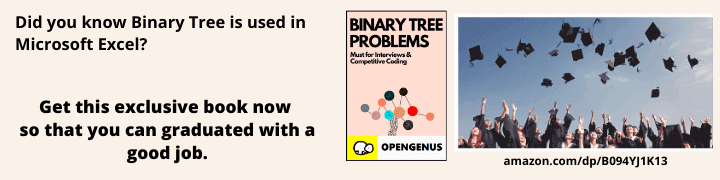
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have explained different ways of Sorting in Ruby Programming Language. This include sorting an array or hash using in-build method, sorting custom objects and implementing custom sorting algorithms like Selection Sort.
Table of Contents
- Introduction
- Sorting an array / Hash
- Sorting custom objects
- Selection sort algorithm
- Conclusion
Introduction
Sorting is a method for reorganizing data into a specific order, such as alphabetical, numerical, by length, etc. Sorting in Ruby is like most other sorting methods in other languages having its own built-in methods, a few of which we are going to introduce to you.
Sorting an array / hash
For ruby sorting an array is easy just use a simple .sort
method and you have what ever it is you want to sort done in ascending order.
numsArray = [10, 3, 56, 5, 24]
numsArray.sort #=> [3, 5, 10, 24, 56]
The sort method will return a new array, if you want to alter the original array you can use the sort! method which is much faster in terms of performance.If you want more control over your sort method(choosing how you want the array sorted) you can use the sort_by method.
stringArray = ["Ruby", "JavaScript", "Python"]
stringArray.sort_by{|stringArray| stringArray.length}
#=> ["Ruby", "Python", "JavaScript"]
As you can see the sort_by method accepts a block(information between {}) it is able to sort the array by the information given inside the block. You can also sort in reverse order by adding a negative sign or using the reverse method after sorting
stringArray.sort_by{|stringArray| stringArray.length}.reverse()
or
stringArray.sort_by{|stringArray| -stringArray.length}
would give the same result.
#=> ["JavaScript", "Python", "Ruby"]
To sort a hash you would use the same methods, the only difference is when a hash is sorted it becomes an array, you would simply have to use the to_h method to convert it back to a hash.
hash ={shoes:100, shirts:500, pants:20, socks:10}
hash.sort_by {|hash| hash.last}.to_h
#=> {socks:10, pants:20, shoes:100, shirts:500}
In this example the last method sorts the hash by using the value so we get the sorted hash in ascending order 10, 20, 100, 500.
Sorting custom objects
To sort custom objects in Ruby, you can define a custom sorting method that compares the objects based on specific attributes or criteria.
Here's an example of how you can sort an array of custom objects based on a specific attribute:
class Person
attr_accessor :name, :age
def initialize(name, age)
@name = name
@age = age
end
def to_s
"#{@name} (#{@age})"
end
end
# Create an array of Person objects
people = [
Person.new("John", 30),
Person.new("Jane", 25),
Person.new("Bob", 45),
Person.new("Alice", 20)
]
# Sort the array based on age in ascending order
people.sort! { |a, b| a.age <=> b.age }
# Print out the sorted array
people.each { |person| puts person.to_s }
In this example, we define a Person class with name and age attributes. We create an array of Person objects, and then sort the array based on the age attribute using the sort! method. The sorting method takes two objects a and b, and returns -1, 0, or 1 depending on whether a is less than, equal to, or greater than b respectively.
We use the spaceship operator <=> to compare the age attributes of the Person objects in the sorting method. The sorted array is then printed out using the each method.
You can modify the sorting method to sort the objects based on other attributes or criteria.
Selection sort algorithm
Selection sort is the process of taking the smallest element in the array and placing it at the begining of the array until the array is sorted. Here is an example
def selection_sort(arr)
n = arr.length
for i in 0..n-2
min_idx = i
for j in i+1..n-1
if arr[j] < arr[min_idx]
min_idx = j
end
end
arr[i], arr[min_idx] = arr[min_idx], arr[i]
end
return arr
end
# Example usage
arr = [64, 25, 12, 22, 11]
sorted_arr = selection_sort(arr)
puts sorted_arr.inspect #=> [11, 12, 22, 25, 64]
In this example, selection_sort takes an array as input, and sorts the elements of the array in ascending order using the selection sort algorithm.
The outer loop iterates over the array from the first element to the second-to-last element. The inner loop searches for the smallest element in the remaining unsorted portion of the array, and updates the index of the minimum element. After the inner loop completes, the smallest element is swapped with the first unsorted element in the outer loop. This process is repeated until the entire array is sorted.
In the example usage, we create an array with unsorted integers, and call selection_sort to sort the array in ascending order. Finally, we print out the sorted array using puts and inspect.
Conclusion
Sorting with Ruby is very easy with it's built in system. You can sort an array in any way you desire array.sort
array.sort!
array.sort_by
. Have fun sorting your next array!!!