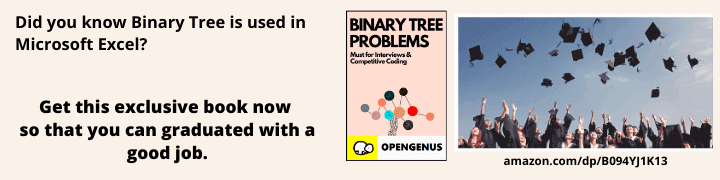
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn about cstring library function strncpy() in C++ and understand the problems with it, safe alternatives and similar functions.
Table of content
- Introduction
- How it works
- Examples
- Problem with strncpy()
- Safe alternative to strncpy()
- Similar functions like strncpy()
Introduction -
strncpy() is a built-in library function of header file <string.h>. It copies first n characters from source array to destination array.
Syntax -
Its syntax is
char *strncpy(char *destination, const char *source, size_t n);
Parameters -
- source - String to be copied to the destination array
- destination - It is a pointer to the destination array where sting to be copied
- n - It is the number of chracters we want to copy from source to destination.
How it works -
It copies the first n characters of the array pointed by source to destination.
if n is less than source length it copies first n chracters from it to the destination and it is not terminated by null charcaters '\0' .
If n is more than source length then stncpy() copies all charcters from source to destination in addition with null characters are added to the destination array till size of destination equals to n.
Return value
It returns a pointer to the destnation.
Examples -
case 1 -
If n is less than size of source array -
#include<iostream>
#include<string.h>
using namespace std;
int main()
{
char source[]="This article is about strncpy() function ";
char destination[60];
strncpy(destination,source,11);
cout<<destination<<"\n";
return 0;
}
Output -
This articl
--------------------------------
Process exited after 6.091 seconds with return value 0
Press any key to continue . . .
case 2 -
If n is more than size of source array -
#include<iostream>
#include<string.h>
using namespace std;
int main()
{
char source[]="This article is about strncpy() function ";
char destination[60];
strncpy(destination,source,strlen(source)+8);
cout<<destination<<"\n";
return 0;
}
Output -
This article is about strncpy() function
--------------------------------
Process exited after 6.101 seconds with return value 0
Press any key to continue . . .
Problem with strncpy() -
1. No null terminator -
If There is no null character in the destination array or our string is not null terminated than it can create problem with our program or code sooner or later . A not null terminated string in c++ is a risky code which anytime may break during exection of the program.It can cause segmentation fault in our program.
So strncpy() does not gurantees that our destination string is null terminated always and hence a risky code for our program.
Example -
#include <bits/stdc++.h>
using namespace std;
int main()
{
char source[20] = "Opengenus article ";
char destination[20];
strncpy(destination, source, 5);
cout<< "Copied string: \n" << destination;
return 0;
}
Output -
Copied string:
Openg EĻ@
--------------------------------
Process exited after 4.846 seconds with return value 0
Press any key to continue . . .
2. Overflow -
This function does not check of overflow i.e if we try to copy a source string to destination which has size less than source it does not throw an error and leads to undefined behaviour.
Example -
#include <bits/stdc++.h>
using namespace std;
int main()
{
char source[20] = "Opengenus article ";
char destination[5];
strncpy(destination, source, sizeof(source));
cout<< "Copied string: \n" << destination;
return 0;
}
Output -
Copied string:
Opengenus article
--------------------------------
Process exited after 4.963 seconds with return value 0
Press any key to continue . . .
Safe Alternative functions which gurantees null termination -
1. snprintf()
2. strlcpy()
Above functions gurantees that our destination string will be null terminated . They do so by copying n-1 or (destination size -1) characters to destination and can terminate the source string if necessary and at last append the null character .
Lets take an example of snprintf()-
syntax of sprintf() -
int snprintf (char *str, size_t size, const char *format, ...);
Parameters -
- str: It is a character type buffer
- size: it defines the maximum number of characters or bytes that can be stored in the buffer.
- format: In C language, the string defines a format that contains the same type of specifications as the format in printf()
- ' ⦠' : It is an optional (ā¦) parameter or argument such as like %d %f as we have seen like in printf(%d,numint) .
Return value -
It return the number of characters that have been written on the buffer of size large enough without including the null terminating character.
If characters to be written are larger than buffer size than it returns a negative value.
example -
When buffer size in snprintf() is more than string (i.e allowed size is 34)-
#include<bits/stdc++.h>
int main ()
{
char *c = "Opengenus article";
char buff[100];
int res = snprintf (buff, 34, "%s \n", c);
printf (" The given string is: %s \n the total number of stored character: %d \n", buff, res);
return 0;
}
Output -
The given string is: Opengenus article
the total number of stored character: 19
--------------------------------
Process exited after 0.5136 seconds with return value 0
Press any key to continue . . .
When buffer size in snprintf() is less than string (i.e allowed size is 11) It simply print only allowed size string in output-
#include<bits/stdc++.h>
using namespace std;
int main ()
{
char *c = "Opengenus article";
char buff[100];
int res = snprintf (buff, 11, "%s \n", c);
cout<<" The given string is: "<< buff ;
cout<<"\n the total number of stored character : "<<res;
return 0;
}
Output -
The given string is: Opengenus
the total number of stored character : 19
--------------------------------
Process exited after 0.6179 seconds with return value 0
Press any key to continue . . .
Similar functions like strncpy() -
1. strcpy() -
strcpy() is a library function in c++ used to copy one string into another .
syntax -
char* strcpy(char* destination, const char* source);
source - string which will be copied
destination - pointer to the array where source string to be copied
Return value -
It returns pointer to the destination string.
#include<bits/stdc++.h>
using namespace std;
int main ()
{
char source[]="strcpy function ";
char destination[40];
strcpy(destination, source);
cout << "source : " << source << "\ndestination: " << destination ;
return 0;
}
Output -
source : strcpy function
destination : strcpy function
--------------------------------
Process exited after 5.635 seconds with return value 0
Press any key to continue . . .
2. memcpy() -
This fynction copies n characters from source to destination .
syntax -
void *memcpy(void *destination, const void * source, size_t n)
source - pointer to the source of data to be copied to the destination array.It is typecasted to void* .
destination - It is a pointer to the destination array where source data to be copied .It is typecasted to void* .
n - It is the number of chracters we want to copy from source to destination.
Return value -
It returns pointer to the destination .
Example -
#include<bits/stdc++.h>
using namespace std;
int main () {
const char source[50] = "Opengenus article about memcpy function ";
char destination[50];
memcpy(destination, source, strlen(source)+1);
cout<<" copied string by memcpy : "<<destination;
return(0);
}
Output -
copied string by memcpy : Opengenus article about memcpy function
--------------------------------
Process exited after 5.61 seconds with return value 0
Press any key to continue . . .
With this article at OpenGenus, you must have the complete idea of using strncpy in C++ Programming Language.