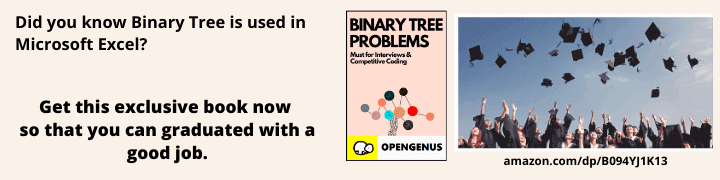
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will work on the C++ project of Typing Speed. We will learn about implementation of the complete program in C++ which will check our typing speed.
This is a good project to boost your portfolio and is a good project of intermediate level for B.Sc, M.Sc students for C++ Programming Language.
Table of content
- Introduction
- Approach of Designing
- Implementation of program for Typing speed test in c++
- Incremental feature
Introduction
It is a console based c++ program aiming to evaluate users typing speed e.g total number of letters ,total number of words,words per minute etc. Here we used dynamic arrays to allocate and deallocate memories and other properties of c++ language.
This application will show users typing speed results in different Parameters like -
- Total number of words.
- Total number of letters.
- TOTAL TIME
- Words/minute
At first page we get a introduction page where we type our name and then in 2nd screen page it takes us to typing playground where we should type paragraphs as usual and after we have typed a paragraph and press enter we get to the third screen where it shows out typing stat's as discussed above in points.
Approach of Designing -
Here we have to count the number of letters and words typed by user in a particular frame of time and then we have to save those numbers and after some calculations return the stat's of user's typing speed.
So for storing the letters we have used chracter arrays also if user wants to ommit some characters he can use backspace also so we keep our chracter array flexible to changes.moreover we did not previously defined the word limit a user can type so we implement it by using dynamic memory allocation so that size of our array can be adjusted accordingly at run time.moreover after completion of program it deallocates memory automatically ance clearing the space .
Program implementation flow -
First from main function our program calls startscreen function
startscreen() -
This function basically helps to display the start screen of our program and takes our name as input and returns to calling function .
after that our control gos to main function again. now after getting username we again call the function userStatus .
user_interface(char &curr_word, string userName) -
userName is passed as argument to it .It takes input a character which is required to start the typing speed test once user entered a valid character it returns time start as 0 second.It also calls function home screen which takes to our working page or second page where we will write our paragraph.
homescreen(string name) -
it helps to display the second screen where we type the program .It takes name as an argument and displays the top format of our second screen in our application.
Now again control goes to main function and our program calls display() function .it takes the time and other values as arguments and works as explained below.
void display(char &curr_word, char &str, int &total_letters, int &starting_time, string userName) -
This functions displays our written paragraph on screen and do so until user press enter once it press enter it calls other caclculating functions and then return the evaluation of users typing skills according to different parameters.according to different conditions it calls different functions calculations() and userStatus as their name suggest well of their functionality it is explained below.
void calculation(char &str, const char curr_word, int &total_letters, int &totalWords) and int time_span(int &starting_time) -
These two functions basically helps in calculating and counting time , words and letters related tasks as required by our program for the final output for evaluation of users typing skills. void calculation() this function basically helps to dynamically expand our strings if its capacity is full and count the number of words and letter along with it moreover it calls time_span() function which calculate time related calculations like time passes total time etc and return the value of time and then at last display() function calls userstatus function which after all calculations and working pass all stats as arguments to userStatus() function which displays them finally.
void userStatus(int totalWords, int total_letters, int seconds, string name) -
It displays our final evaluation on typing speed ranging from word count,total words ,total word/minute.
at last all of the memory which is extra created by the function as finally deallocated after the execution of program is completed.
Implementation of program for Typing speed test in c++ -
#include <bits/stdc++.h>
#include <conio.h>
using namespace std;
void space() { cout << "\n\n\n"; }
startscreen() -
This function basically helps to display the start screen of our program and takes our name as input and returns to calling function .Here name variable is used to take users name as input after that function system("cls") which clears the console screen and any output generated by it. after that it returns string name to calling function.the header file #include <bits/stdc++.h> is used because we are using string and other functions.
string startscreen()
{
char ch = 1;
string name;
space();
cout << "\t\t\t\t\t TYPING SPEED TEST IN C++ \n";
space();
cout << "\t\t\t***************************************************************************\n";
cout << "\t\t\t| CHECK YOUR TYPING SPEED AND EVALUATE YOUR PERFORMANCE " << ch << " | \n";
cout << "\t\t\t| ENTER YOUR NAME AND START TYPING => |\n";
cout << "\t\t\t***************************************************************************\n\t\t\t\t\t\t";
cin >> name;
system("cls");
return name;
}
homescreen(string name) -
it helps to display the second screen where we type the program .It takes name as an argument and displays the top format of our second screen in our application.It
basically just shows a format of our program in output along with our name as input to it.
void homescreen(string name)
{
cout<<"******************************************************************************************************************************************************************************************************************" ;
cout << "\n\n\n\t\t\t\t\t\t\t\t\t\t\t\tWELCOME " << name << " \n";
space();
cout << "\t\t\t\t\t\t \t\t\t\t\t TYPING SPEED TEST IN C++ \n\n\n";
cout<<"*****************************************************************************************************************************************************************************************************************" ;
space();
cout << "LET'S SEE OUR TYPING SPEED PERFORMANCE AND EVALUATE HOW FAST AND ACCURATE WE CAN TYPE ===> \n";
space();
}
user_interface(char &curr_word, string userName) -
userName is passed as argument to it .It takes input a character which is required to start the typing speed test once user entered a valid character it returns time start as 0 second.It also calls function home screen which takes to our working page or second page where we will write our paragraph.this function calls homescreen function by passing username which we get from user in starting by calling startscreen , thereafter the curr_word variable calls getch() function which is a part of #include<conio.h> header file so getch() reads a character from keyboard and immediately returns without storing in buffer or pressing enter.so here we want 'R' to be typed by user so as to start our program typing test hence it read until 'R' is not typed once typed it takes us to start typing test window.
int user_interface(char &curr_word, string userName)
{
homescreen(userName);
cout << "To start the Test, press enter \'R-key\' \n";
curr_word = getch();
curr_word = tolower(curr_word);
while (curr_word != 'R')
{
cout << "Please press the valid key : \n";
curr_word = getch();
}
cout << "\t\t\t YOUR TEST STARTS, start typing \n\n\n";
return time(0);
}
void userStatus(int totalWords, int total_letters, int seconds, string name) -
It displays our final evaluation on typing speed ranging from word count,total words ,total word/minute.
here it first clears the screen and thereafter it calls homescreen function and shows final results by using following variables.
- totalwords gives total words typed.
- total_letters gives total letters typed.
- seconds is used to count total seconds of time passed.It gives us time by dividing seconds by 60 i.e seconds/60 giving us total time.
- words per minute by dividing totalwords by seconds/60 .
void userStatus(int totalWords, int total_letters, int seconds, string name)
{
system("cls");
homescreen(name);
cout << "\n\t\t\t\t\t\t\t\t " << name << "'S TYPING STATS "
<< "\n\n";
cout << "\t\t\t\t\t\t\t\tTOTAL WORDS : " << totalWords << "\n";
cout << "\t\t\t\t\t\t\t\tTOTAL LETTERS : " << total_letters << "\n";
cout << "\t\t\t\t\t\t\t\tTOTAL TIME : " << seconds / 60 << ":" << seconds % 60 << "\n";
cout << "\t\t\t\t\t\t\t\tWords/minute : " << (totalWords / (seconds / 60)) << "\n\n\n";
}
int time_span(int &starting_time) -
time_span() function which calculate time related calculations like time passes total time etc . It displays total Time passed and also returns total time passed in seconds.It takes starting time in seconds as argument and currentTime variable used time() function of c standard #include<time.h> library or #include<ctime.h> library which is all can be called by <bits/stdc++.h> library.now we declared ststic variable min which didn't fade off after function call is over it keeps data until our program not executed completely.moreover if sec variable >59 seconds mnute will increase and starting time again set to default. and this function returns total seconds to the calling function.
int time_span(int &starting_time)
{
int currentTime = time(0);
int sec = currentTime - starting_time;
static int min;
if (sec > 59)
{
min++;
starting_time = time(0);
}
cout << "Time passed : " << min << " : " << sec << "\n";
return (min * 60) + sec;
}
void calculation(char &str, const char curr_word, int &total_letters, int &totalWords) -
void calculation() this function basically helps to dynamically expand our strings if its capacity is full and count the number of words and letter along with it moreover it calls time_span() function which calculate time related calculations like time passes total time etc .In this function first we check our arguments to the given condition like cur_word and total_letters below as according to their values and count so that we can resize our string Str accordingly.for resizing we first take a pointer to a character array as temp and now we copy old string to this new pointer array then we delete old string and again we re assign the old string with new one and accordingly we increase or decrease the size of totalletters variable .e.g we check for backspace by compairing cur_word value to 8 if a backspace is pressed we delete the last charcater and then again store it to string and similarly if backspace is not pressed we simply add new char to str and increase total letter value .moreover after his alteration we again check our string for totalwords and accordingly increase this variable size if it passes our conditions i,e if our string contains a space or not if previous index doesn't have space but current index have we get that user has pressed the space so we increase the totalwords count by 1 and continue.
void calculation(char *&str, const char curr_word, int &total_letters, int &totalWords)
{
if (curr_word != 8)
{
char *temp = new char[total_letters + 1];
for (int i = 0; str[i] != '\0'; i++)
{
temp[i] = str[i];
}
temp[total_letters - 1] = curr_word;
temp[total_letters] = '\0';
delete[] str;
str = temp;
total_letters++;
}
if (curr_word == 8 && total_letters != 0)
{
char *temp = new char[total_letters - 1];
for (int i = 0; i < total_letters - 1; i++)
{
temp[i] = str[i];
}
temp[total_letters - 2] = '\0';
delete[] str;
str = temp;
total_letters--;
}
for (int i = 0; str[i] != '\0'; i++)
{
if (str[i - 1] != 32 && str[i] == 32)
{
totalWords++;
}
}
}
void display(char &curr_word, char &str, int &total_letters, int &starting_time, string userName) -
This functions displays our written paragraph on screen and do so until user press enter once it press enter it calls other caclculating functions and then return the evaluation of users typing skills according to different parameters.according to different conditions it calls different functions calculations() and userStatus ().
this function simply initializes totalwords and takes cur_word as input once calls different functions from calculation,homescreen to time_span and do so until user doesn't presses enter once user press enter it comes out of do while loop and displays the final result on output screen.
main() function simply responsible for initialization and calling of different function at required time accordingly.
void display(char &curr_word, char *&str, int &total_letters, int &starting_time, string userName)
{
do
{
int totalWords = 0;
curr_word = getch();
if ((curr_word >= 32 && curr_word <= 126) || curr_word == 13 || curr_word == 8)
{
calculation(str, curr_word, total_letters, totalWords);
system("cls");
int currentTime = time(0);
homescreen(userName);
cout << "\n\nTotal Letters = " << total_letters - 1 << "\n";
cout << "\n\nTotal Words = " << totalWords << "\n";
time_span(starting_time);
space();
for (int i = 0; str[i] != '\0'; i++)
{
cout << str[i];
}
space();
space();
cout << "To END THE PROGRAM : PRESS ENTER-KEY\n";
}
else
{curr_word = getch();
}
if (curr_word == 13)
{
userStatus(totalWords, total_letters, time_span(starting_time), userName);
}
} while (curr_word != 13);
}
int main()
{
int total_letters = 1;
char *str = new char[total_letters]{'\0'};
char curr_word;
string userName = startscreen();
int starting_time = user_interface(curr_word, userName);
display(curr_word, str, total_letters, starting_time, userName);
delete[] str;
str = NULL;
return 0;
}
Output of above program -
homepage
TYPING SPEED TEST IN C++
***************************************************************************
| CHECK YOUR TYPING SPEED AND EVALUATE YOUR PERFORMANCE ☺ |
| ENTER YOUR NAME AND START TYPING => |
***************************************************************************
SHIVAM
Second page
**********************************************************************************************************************************************************
WELCOME SHIVAM
TYPING SPEED TEST IN C++
************************************************************************************************************************************************************
LET'S SEE OUR TYPING SPEED PERFORMANCE AND EVALUATE HOW FAST AND ACCURATE WE CAN TYPE ===>
Total Letters = 385
Total Words = 69
Time passed : 2 : 18
WE HAVE SEEN MANY PROJECTS ON C++ SO THIS ONE IS JUST USING DYNAMIC ARRAY IMPLEMENTATION A PROGRAM TO CHECK AND EVALUATE USERS TYPING SPEED IN C++ . I HAVE USED DIFFERENT CONCEPTS OF C++ LANGUAGES AND IT IS A COMMAND LINE PROGRAM WHICH WILL SHOW US DIFFERENT PARAMETERS OF USERS TYPING SPEED AFTER WE HAVE WRITTEN A PARAGRAPH WHICH WILL BE EVALUATED AS AN EXAMPLE OF OUR TYPING PROGRAM
To END THE PROGRAM : PRESS ENTER-KEY
final evaluation page
**********************************************************************************************************************************************************
WELCOME SHIVAM
TYPING SPEED TEST IN C++
************************************************************************************************************************************************************
LET'S SEE OUR TYPING SPEED PERFORMANCE AND EVALUATE HOW FAST AND ACCURATE WE CAN TYPE ===>
SHIVAM'S TYPING STATS
TOTAL WORDS : 69
TOTAL LETTERS : 387
TOTAL TIME : 2:39
Words/minute : 34
--------------------------------
Process exited after 173.5 seconds with return value 0
Press any key to continue . . .
Incremental Feature
Here lets have a default paragraph which we will type by seeing it and check our accuracy and speed accordingly .And we create our display() function such that it only accepts and shows the characters which are in our paragraph as it is that is now we have to type according to paragraph character by character any deviation from original paragraph and our program will not accept that chracter and did not display it on screen.
Therefore all features of program are same except void displayingSentences(char ¤tLetter, char &str, int &totalLetters, int &startTime, string userName) and homescreen() functions which will now display a default paragraph to type for users since we have added a default paragraph in it and the display() function only let those chracters serial wise in our output window which are in our program. lets have a look at our display and homescreen function now.
homescreen() -
string s;
void homescreen(string name)
{
cout<<"**********************************************************************************************************************************************************" ;
cout << "\n\n\n\t\t\t\t\t\t\t\t WELCOME " << name << " \n";
space();
cout << "\t\t\t\t\t\t \t\t TYPING SPEED TEST IN C++ \n\n\n";
cout<<"************************************************************************************************************************************************************" ;
space();
s= " This is console based c++ program for the typing test .this is an example paragraph .A cryptocurrency is a virtual or digital currency that is highly secured by cryptography or encryption techniques which makes it nearly impossibleto counterfeit such cryptocurrency Cryptocurrency is an alternative means of exchange and the transactionsof crypto are highly secured using cryptography. Crypto is electronicmoney or we can say that it is a type of virtual money. The power ofgoverning or controlling the distribution of Cryptocurrency does not liein the hands of the government or any single authority.\n";
cout<<s;
space();
}
display() -
void display(char &curr_word, char *&str, int &total_letters, int &starting_time, string userName)
{
int i=0;
do
{
int totalWords = 0;
curr_word = getch();
if(s[i++]==curr_word){
if ((curr_word >= 32 && curr_word <= 126) || curr_word == 13 || curr_word == 8)
{
calculation(str, curr_word, total_letters, totalWords);
system("cls");
int currentTime = time(0);
homescreen(userName);
cout << "\n\nTotal Letters = " << total_letters - 1 << "\n";
cout << "\n\nTotal Words = " << totalWords << "\n";
time_span(starting_time);
space();
for (int i = 0; str[i] != '\0'; i++)
{
cout << str[i];
}
space();
space();
cout << "To END THE PROGRAM : PRESS ENTER-KEY\n";
}
else
{curr_word = getch();
}
if (curr_word == 13)
{
userStatus(totalWords, total_letters, time_span(starting_time), userName);
}
}
else i--;
}while (curr_word != 13);
}
int main()
{
int total_letters = 1;
char *str = new char[total_letters]{'\0'};
char curr_word;
string userName = startscreen();
int starting_time = user_interface(curr_word, userName);
display(curr_word, str, total_letters, starting_time, userName);
delete[] str;
str = NULL;
return 0;
}
Second page
**********************************************************************************************************************************************************
WELCOME shivam
TYPING SPEED TEST IN C++
************************************************************************************************************************************************************
This is console based c++ program for the typing test .this is an example paragraph .A cryptocurrency is a virtual or digital currency that is highly secured by cryptography or encryption techniques which makes it nearly impossibleto counterfeit such cryptocurrency Cryptocurrency is an alternative means of exchange and the transactionsof crypto are highly secured using cryptography. Crypto is electronicmoney or we can say that it is a type of virtual money. The power ofgoverning or controlling the distribution of Cryptocurrency does not liein the hands of the government or any single authority.
Total Letters = 583
Total Words = 93
Time passed : 4 : 49
This is console based c++ program for the typing test .this is an example paragraph .A cryptocurrency is a virtual or digital currency that is highly secured by cryptography or encryption techniques which makes it nearly impossibleto counterfeit such cryptocurrency Cryptocurrency is an alternative means of exchange and the transactionsof crypto are highly secured using cryptography. Crypto is electronicmoney or we can say that it is a type of virtual money. The power ofgoverning or controlling the distribution of Cryptocurrency does not liein the hands of the government or any single authority.
To END THE PROGRAM : PRESS ENTER-KEY
final evaluation page
**********************************************************************************************************************************************************
WELCOME shivam
TYPING SPEED TEST IN C++
************************************************************************************************************************************************************
This is console based c++ program for the typing test .this is an example paragraph .A cryptocurrency is a virtual or digital currency that is highly secured by cryptography or encryption techniques which makes it nearly impossibleto counterfeit such cryptocurrency Cryptocurrency is an alternative means of exchange and the transactionsof crypto are highly secured using cryptography. Crypto is electronicmoney or we can say that it is a type of virtual money. The power ofgoverning or controlling the distribution of Cryptocurrency does not liein the hands of the government or any single authority.
shivam'S TYPING STATS
TOTAL WORDS : 93
TOTAL LETTERS : 585
TOTAL TIME : 5:0
Words/minute : 18
--------------------------------
Process exited after 315.9 seconds with return value 0
Press any key to continue . . .
A brief program flow is given by below diagram .
From above program we get an idea of how we can use different functions and properties of c++ language to design an application.
With this article at OpenGenus, you must have the complete idea of working on the project of Typing Speed in C++ Programming Language.