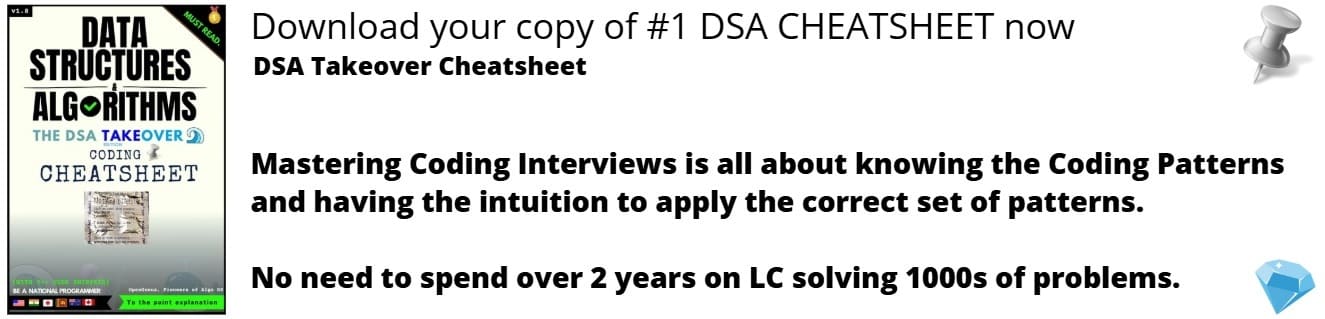
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
// Table of contents
Introduction
When it comes to web development, two of the most popular communication protocols are WebSocket and REST. While they both facilitate communication between a client and server, they have distinct differences in terms of their structure, performance, and use cases. In this article at OpenGenus, we will compare WebSocket and REST and explore their advantages and disadvantages.
WebSockets
WebSocket is a protocol that enables bi-directional, real-time communication between a client and server. It is designed to facilitate communication between a browser and a server, but it can also be used for other types of clients. The WebSocket protocol is supported by most modern browsers and is used extensively in applications that require real-time data updates.
WebSocket is a persistent connection between the client and server, which means that once the connection is established, the client and server can communicate in both directions without the need for multiple requests and responses. This makes WebSocket very efficient for applications that require frequent updates of data, such as chat applications, real-time analytics, and multiplayer games.
WebSocket uses a single TCP connection that is kept open for the duration of the communication session. This eliminates the overhead of establishing multiple TCP connections for each request, which is a common practice in RESTful APIs. WebSocket also supports full-duplex communication, which means that data can be sent and received simultaneously in both directions.
REST
REST (Representational State Transfer) is an architectural style that defines a set of constraints and principles for designing web services. It is a stateless, client-server protocol that uses HTTP for communication. REST is the most common way of building web APIs, and it has become the de facto standard for many web services.
RESTful APIs use a request-response model, where the client sends a request to the server, and the server responds with the requested data. RESTful APIs are resource-oriented, meaning that each resource is identified by a unique URL, and clients can use HTTP verbs to perform CRUD (Create, Read, Update, Delete) operations on these resources.
RESTful APIs use a stateless communication model, which means that the server does not maintain any client state. Instead, each request from the client must contain all the information necessary to fulfill the request. This makes RESTful APIs scalable and easy to cache, but it can also result in more network overhead for applications that require frequent updates.
Implementations
WebSocket Implementation
Here is an example of a WebSocket client that connects to a Websocket server and sends a message:
const socket = new WebSocket('ws://localhost:3000');
socket.addEventListener('open', function (event) {
socket.send('Hello Server!');
});
socket.addEventListener('message', function (event) {
console.log('Server says: ', event.data);
});
socket.addEventListener('close', function (event) {
console.log('WebSocket connection closed.');
});
Example of REST
RESTful API implementation
Below is an example a RESTful API endpoint that retrieves a list of products:
GET /api/products HTTP/1.1
Host: example.com
Accept: application/json
HTTP/1.1 200 OK
Content-Type: application/json
{
"products": [
{
"id": 1,
"name": "Product 1",
"price": 9.99
},
{
"id": 2,
"name": "Product 2",
"price": 19.99
}
]
}
Comparison: WebSockets vs REST
Now that we have an understanding of WebSocket and REST, let's compare the two protocols.
- Performance
- Use cases
- Security
- Scalability
- Development complexity
- Performance
WebSocket is generally more performant than REST because it uses a persistent connection and supports full-duplex communication. This means that there is less overhead for establishing connections and sending data, and real-time data updates can be sent immediately without the need for polling. REST, on the other hand, requires multiple requests and responses to transfer data, which can result in more network overhead. However, REST is still very efficient for applications that do not require real-time data updates.
- Use Cases
WebSocket is best suited for applications that require real-time communication, such as chat applications, real-time analytics, and multiplayer games. REST is more suitable for applications that require data retrieval and manipulation, such as e-commerce sites, social media platforms, and news sites.
- Security
WebSocket and REST both support secure communication using SSL/TLS. However, WebSocket can be more susceptible to security vulnerabilities because it uses a persistent connection, which means that a compromised connection could potentially allow an attacker to access real-time data updates.
REST, on the other hand, uses a request-response model, which means that each request must be authorized before the server can respond with the requested data. This makes REST more secure than WebSocket for applications that require authorization and authentication.
- Scalability
RESTful APIs are very scalable because they use a stateless communication model and can be easily cached. This means that multiple clients can make requests to the same endpoint, and the server can respond with the same data without having to generate a new response each time.
WebSocket, on the other hand, can be more difficult to scale because it requires a persistent connection for each client. This means that the server must maintain a separate connection for each client, which can become problematic as the number of clients increases.
- Development Complexity
WebSocket and REST both require different development approaches. WebSocket requires a specialized server that can handle real-time communication, while REST can be implemented using any HTTP server.
WebSocket also requires a different programming paradigm than REST. With WebSocket, the server must listen for incoming messages and send updates proactively, while with REST, the server only responds to client requests.
Conclusion
WebSocket and REST are both widely used communication protocols for web applications. WebSocket is best suited for applications that require real-time data updates, while REST is more suitable for applications that require data retrieval and manipulation.
WebSocket is generally more performant than REST and supports full-duplex communication, but it can be more difficult to scale and is more susceptible to security vulnerabilities.
REST is very scalable and easy to cache, but it requires multiple requests and responses to transfer data and may not be suitable for real-time applications.
Ultimately, the choice between WebSocket and REST depends on the specific requirements of the application. For applications that require real-time communication, WebSocket is the obvious choice, while for applications that require data retrieval and manipulation, REST is the better option.