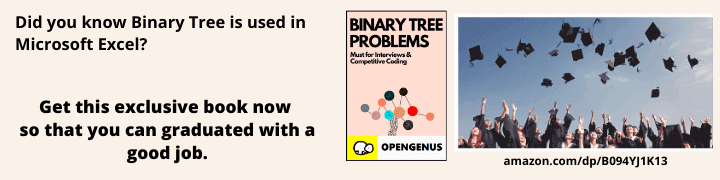
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have covered 2 important assembly instructions namely MOVSX and MOVSXD which is used to move a data considering sign bits. It is an alternative to MOVZX.
Table of contents:
- MOVSX - Move with Sign Extend
- C program using MOVSX as intrinsic
- MOVSXD - Move with Sign Extend Doubleword
MOVSX - Move with Sign Extend
MOVSX stands for "Move with Sign Extend".
MOVSX is an assembly instruction that is used to copy data from a source operand to a destination operand where the sign occupies the extra bits in the destination. If the source operand stored a negative number, then 1 is stored in the extra bits. For positive number, 0 is stored in the extra bits.
MOVSX instruction is available on all x86 and x86-64 processors.
Following is an example of using MOVSX in Assembly code:
mov al, -5 ; store -5 in the al register
movsx eax, al ; zero-extend al to eax
Example:
-5 is stored in AL register (8 bits) and it is stored as 2s complement. The content of AL register will be 11111011.
After movsx operation, the value of eax will be: 11111111111111111111111111111011
The last 8 bits are same as AL and all other 24 bits are 1 as AL stores a negative number.
If AL stored 5, then content of AL = 00000101
After the movsx operation, the value of eax will be: 00000000000000000000000000000101
The last 8 bits are same as AL and all other 24 bits are 0 as AL stores a positive number.
C program using MOVSX as intrinsic
The intrinsic for MOVSX is _mm_cvtsepi16_epi32
. It takes a 16 bit data and converts to a 32 bit data with sign extension.
Following is the complete C program using MOVSX as intrinsic:
#include <stdio.h>
#include <immintrin.h>
int main() {
short a = -32767;
int b = _mm_cvtsepi16_epi32(_mm_cvtsi32_si128(a));
printf("%d\n", b);
return 0;
}
MOVSXD - Move with Sign Extend Doubleword
MOVSXD stands for "Move with Sign Extend Doubleword".
MOVSXD is an x86-64 instruction that is same as MOVSX in functionality and moves a doubleword (32 bits) or quadword (64 bits) from a source operand to destination operand. MOVSX operates on 16 bit data for a system with 16 bit word.
Following is an example of using MOVSX in Assembly code:
mov al, -12 ; store -12 in the al register
movsxd eax, al ; zero-extend al to eax
With this article at OpenGenus, you must have the complete idea of MOVSX and MOVSXD assembly instructions.