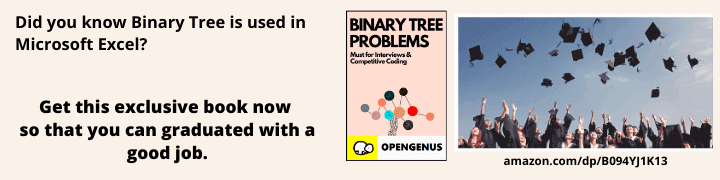
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will look at how to write a Python script that will create a gist using GitHub API.
- Github Gists are a way to share code with others. Gists are git repositories- they can be cloned or forked.
- They can be public or secret.
- A public gist is visible to everyone and can be searchable. The author if created many gists, it's visible by going to list all gists on the gist homepage.
- A secret gist is invisible and can only be viewed by people to whom a URL is shared. It's non searchable and not easily found.
- Once a gist is created as public it can't be modified to secret.
- Because gists are repositories too, one can view the commit history and the changes made to those files.
- One can use REST API to create, read, update and delete the gists. We can view a public gist without any authentication i.e anonymously. But, to create a list we need to have been signed-in on github.
- whenever a response for a particular file in a gist is given we need to check for a key in the response called 'truncated'. If it's true, it means that the limit of the file exceeds 1MB. If it's smaller than that we get the complete content as the response.
- For files which are greater than 1MB we can simply get the complete content by a get request. For files greater than 10MB we need to clone the gist via git_pull_url.
- Out of all the hundred different things you can do with the API, we are going to create a gist using python by using requests module.
- Requests module helps in sending HTTP requests using python.
- Every request sent by the user receives a response. The response is an object. It contains key-value pairs which describe the contents, headers, time elapsed, encoding, history etc.
- To install Requests module, we need to use pip, which is a package installer and also manages it.
- Type in the command as below in the terminal and hit enter.
pip install Requests
- This installs the python module required.
- To create a gist we do the following
import requests
import json
GITHUB_API="https://api.github.com"
API_TOKEN=''
'''use your personal access token and don't forget to add gist permissions'''
#make a request URL
url=GITHUB_API+"/gists"
print ("Request URL: {}".format(url))
#print headers,parameters,payload
headers={
'Authorization':'token {}'.format(API_TOKEN)
}
params={
'scope':'gist'
}
payload={
"description":"Test Gist for OpenGenus",
"public":True,
"files":
{
"python requests module":
{
"content": '''Python requests has 3 parameters:
1)Request URL\n
2)Header Fields\n
3)Parameter \n
4)Request body'''
}
}
}
print(headers,params,payload)
#make a post request
res=requests.post(url,headers=headers,params=params,data=json.dumps(payload))
#print response and also convert into JSON format
print(res)
print(res.status_code)
print(res.url)
print(res.text)
json_content=json.loads(res.text)
json_formatted_str = json.dumps(json_content, indent=4)
print(json_formatted_str)
print(json_content['url'],json_content['id'])
Output when the code gets executed is:
There will be a gist created with description, filename and contents.
Explanation:
We are importing requests module and json(this can be installed using pip install json). To create a request we need to remember some important things.
A request should have a URL, a header, parameters and the body/data. We are using post request to send the required information about what should be the description, file name and its contents. We are taking help from json because whenever we receive a response or are writing an object we receive/write it in a string format.
Whenever a gist is created the response object has the contents,id,url and other important information. This information can be used to get the gists using the get method to the api using a gist id.
The script for getting a post is similar to that of creating,
import requests
import json
GITHUB_API="https://api.github.com"
API_TOKEN=''
'''use your personal access token and don't forget to add gist permissions'''
gist_id=''
'id of the gist which you want to access'
#make a request URL
url=GITHUB_API+"/gists/{}".format(gist_id)
print ("Request URL: {}".format(url))
res=requests.get(url)
print(res)
print(res.status_code)
print(res.url)
print(res.text)
json_content=json.loads(res.text)
json_formatted_str = json.dumps(json_content, indent=4)
print(json_formatted_str)
print(json_content['url'],json_content['id'])
With this article at OpenGenus, you must have the complete idea of how to create a gist using a Python script.