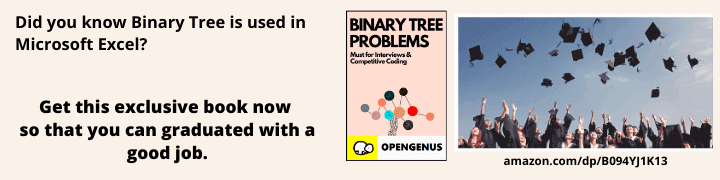
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
An Array is a continuous memory allocation of the group of like-typed variables that are referred to by a common name. In this article, we have focused on 2D array in Java which is a variant of the usual 1D array by introducing a new dimension.
In short, it is defined as:
int[][] arr = new int[10][20];
// Other methods further in this
// article at OpenGenus
For example, for storing 10 integers, 40 bytes of continuous memory space is allocated for storing these integers in the form of an array. This is because each integer occupies 4 byte of memory in java. Each integer value is stored at a particular index of the array formed.
So, we do not need to create different variables and remember their names for storing multiple values of same datatype.
Arrays help in accessing address of each value of a datatype if we have address of the start. So, it makes ACCESSING and STORING data easy.
There are mainly two common variations of Arrays, namely :
- 1-D Array (or commonly called Array)
- Multidimensional Arrays
2-D Array is the simplest form of a Multidimensional array. Now, we are going to explore 2-D arrays in a greater detail.
2-D Arrays
2-D Arrays can be seen as an array of a 1-D array. They are often called matrices.
For our better undestanding, lets take up an example. If we have to make a 8 * 8 chessboard (matrix), then we can accomplish this task in 2 ways.
Way 1 : Here, we can simply use 1-D arrays. But the problem is that now we need to manually create many references i.e. a different reference for each row (row 0, row1, row2 and so on).
Or we can even create an array of 64 size (as there are 8 cells, so 8 * 8 = 64). So, suppose we have to go to ist row in jnd column, we have to manually calculate our desitination cell via (i * 8 + j). This task is quite tedious and time consuming.
Way 2 : A better way to do this is by using 2-D Arrays where we can easily access all the elements and there is no need to do calculations.
Declaration of a 2-D Array
Indirect Method
public class TwoDimensionalArray{
public static void main(String args[]) {
int[][] arr = new int[10][20];
}
}
where 1. int is data_type of the array
2. arr is the array_name
3. 10 is the number of rows in our array
4. 20 is the number of columns in our array
Direct Method
public class TwoDimensionalArray{
public static void main(String args[]) {
int[][] arr = {{1, 2}, {3, 4}};
}
}
where arr is our 2-D array with 2 rows and 2 columns and
1 2
3 4
as elements.
Initializing and Accessing an element of a 2-D Array
public class TwoDimensionalArray{
public static void main(String args[]) {
int[][] arr = new int[3][2];
arr[2][0] = 1;
System.out.println("arr[2][0] = " + arr[2][0]);
}
}
OUTPUT
arr[2][0] = 1
Here, our 2-D array named arr's 3rd row and 1st column has been initialized to 1.
Note: In arrays if size of array is N. Its index will be from 0 to N-1. Therefore, for row_index 2, actual row number is 2+1 = 3.
How are 2-D arrays stored?
2-D arrays are stored internally as a collection of 1-D arrays. When we write
int input[][] = new int[3][4]
This means our 2-D array input points towards a Master Array which is an 1-D array in itself and stores the address of 1-D arrays which stores our matrix's elements. So, here we will have 3 indices of master array and each index stores the address of another 1-D array. Now, this 1-D array has 4 indices which stores the values of corresponding element in our 2-D array.
This can be further explained as each element of the outer array has a reference to each inner array. The picture below shows a 2D array that has 3 rows and 7 columns. Notice that the array indices start at 0 and end at the length -1.
Creating and printing a user defined 2-D Arrays
For accessing rows and columns of 2-D Arrays :
If our 2-D Array is named arr,
then, number of rows = arr.length
and number of columns = arr[0].length
import java.util.Scanner;
public class TwoDimensionalArray{
// Creating a user defined 2-D Array
public static int[][] createarray(){
Scanner s = new Scanner(System.in);
// Taking number of rows and columns in input from user
System.out.println("Enter number of rows");
int rows = s.nextInt();
System.out.println("Enter number of columns");
int columns = s.nextInt();
// Declaring an 2-D Array
int[][] arr = new int[rows][columns];
System.out.println("Enter element of desired matrix");
for (int i = 0; i <arr.length; i++){
for (int j = 0 ; j < arr[0].length; j++){
arr[i][j] = s.nextInt();
}
}
return arr;
}
// Printing a 2-D array
public static void printarray (){
int[][] arr = createarray();
for (int i = 0; i <arr.length; i++){
for (int j = 0 ; j < arr[0].length; j++){
System.out.print(arr[i][j] + " ");
}
System.out.println();
}
}
public static void main(String args[]) {
printarray();
}
}
INPUT
Enter number of rows 3
Enter number of columns 3
Enter element of desired matrix
2 3 2
1 2 3
4 2 3
OUTPUT
2 3 2
1 2 3
4 2 3
Quick Revision
Guess the output of the following questions!
Question 1
public class TwoDimensionalArray{
public static void main(String args[]) {
int[][] arr = {{1, 2}, {3, 4}};
System.out.println(arr);
}
}
Answer: Address of arr gets printed
Question 2
public class TwoDimensionalArray{
public static void main(String args[]) {
int[][] arr = {{1, 2}, {3, 4}};
System.out.println(arr.length);
}
}
Answer: 2 as arr.length denotes number of rows in our 2D array.
Question 3
public class TwoDimensionalArray{
public static void main(String args[]) {
int[][] arr = new int[10][20];
System.out.println(arr[0][0]);
}
}
Answer: 0 as by default each element of our 2D array is initialised by 0.
With this article at OpenGenus, you must have a complete idea of 2D array in Java. Enjoy.