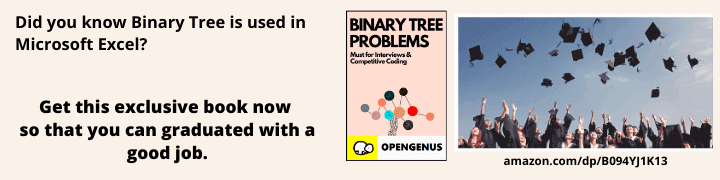
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 15 minutes | Coding Time: 10 minutes
A counter is basically a part of collections module and is a subclass of a dictionary which is used to keep a track off elements and their count. In Python, elements are stored as =Dict= keys and their counts are stored as dict values. These count elements can be positive, zero or negative integers. Simply speaking, if you add the value ‘hello’ thrice in the container, it will remember that you added it thrice. So, Counter counts hashable objects in Python.
A counter class has no restrictions on its keys and values i.e. one can store anything in the value field.
Creating Python Counter Objects :
Counters support three basic types of initialization. It can be called with iterable items or dictionary containing keys and counts or keyword args like mapping.
For creating a new empty counter:
counter = Counter()
print(counter)
--> Counter()
For creating a new counter with values:
counter = Counter(['a','b','c','a','a'])
print(counter)
--> Counter({'a':3, 'b':2, 'c':1})
For creating a new counter with iterable arguments:
counter = Counter('abcdac')
print(counter)
--> Counter({'a':2, 'b':1, 'c': 2, 'd':1})
For creating a new counter with Dictionary arguments:
word = {'blue': 1, 'white': 3, 'black': 2}
counter = Counter(word)
print(counter)
--> Counter({'white': 3, 'black': 2, 'blue': 1})
For creating a new counter with List arguments:
list = ['Black', 'Blue', 'Blue', 'Red']
counter = Counter(list)
print(counter)
--> Counter({'Blue': 2, 'Black': 1, 'Red': 1})
Non-numeric data can also be used for counter values.
Accessing Counters in Python
Counters are accessed like dictionaries. If any key is absent, it does not raise any KeyValue error, instead it shows the count as zero.
c = collections.Counter('abcdefacc')
for letter in 'abcz':
print (letter, c[letter])
--> a 2
b 1
c 3
z 0
Getting Count of Elements in a Counter
We easily count the number of elements in a counter like shown in the example below. For a non-existing key, it will return zero.
c = Counter({'a': 5, 'b': 3, 'c': 6})
c1 = counter['b']
print(c1)
--> 3
Setting Count of Elements in a Counter
We can set the count of an element in the counter as shown in the example below. For a non-existing key, it will get added to the counter.
c = Counter({'a': 5, 'b': 3, 'c': 6})
c['b']=0
print(c)
--> Counter({'a': 5, 'b': 0, 'c': 6})
Deleting an element from a Counter
Any element can easily be removed or deleted from a counter object by using =del=.
c= Counter( {'A':3,'B':1,'C':5})
print(c)
del c['B']
print(c)
--> Counter({'C':5, 'A':3, 'B':1})
Counter({'C':5,'A':3})
Python Counter Methods
Counter objects support three methods apart from those available to all dictionaries. Namely, elements(), most_common() and subtract().
elements()
This method returns the list of elements in the counter.
Only elements with positive counts are returned, if it is less than one, it gets ignored.
c=Counter({'red':2, 'blue':-3, 'green':0})
e= c.elements()
for value in e:
print(value)
--> red
red
The code above will print 'red' twice. This is because it's countg is 2. The other elements- 'blue' and 'green' will be ignored as they do not have a positive value like 'red'.
most_common(n)
This method returns the most common elements from the counter from most common to least common.
If the value of 'n' is not given, it returns all the elements in the counter.
c=Counter({'red':2, 'blue':-1, 'green':0})
m= c.most_common(1)
print(m)
--> [('red' , 2)]
The code above has the output [('red' , 2)] as 'red' is the most common element in the counter with a value of 2.
subtract()
Counter subtract() method is used to subtract element counts from another mapping (counter) or iterable. Inputs and outputs may be zero or negative.
counter = Counter('abcabacaa')
print(counter)
c = Counter('abc')
counter.subtract(c)
print(counter)
--> Counter({'a':5, 'b':2, 'c':2})
Counter({'a':4, 'b':1, 'c':1})
In the above code, using subtract() we get the output Counter({'a':4, 'b':1, 'c':1}) after subtracting 'abc' from the initial Counter containing elements 'abcabacaa'.
update()
This method is used to add counts from another mapping or counter.
counter = Counter('abcabacaa')
print(counter)
c = Counter('abc')
counter.update(c)
print(counter)
--> Counter({'a':5, 'b':2, 'c':2})
Counter({'a':6, 'b':3, 'c':3})
In the code above, update() is used to add 'abc' counts to the counter containing 'abcabacaa' elements. Hence, we get Counter({'a':6, 'b':3, 'c':3}) as the output.
fromkeys(iterable)
This class method is not implemented for Counter objects.
Mathematical operations on Python Counters
c = Counter(a=3, b=1)
d = Counter(a=1, b=2)
c + d # add two counters together: c[x] + d[x]
Counter({'a': 4, 'b': 3})
c - d # subtract (keeping only positive counts)
Counter({'a': 2})
c & d # intersection: min(c[x], d[x])
Counter({'a': 1, 'b': 1})
c | d # union: max(c[x], d[x])
Counter({'a': 3, 'b': 2})
Miscellaneous operations on Python Counters :
sum(c.values()) # total of all counts
c.clear() # reset all counts
list(c) # list unique elements
set(c) # convert to a set
dict(c) # convert to a regular dictionary
c.items() # convert to a list of (elem, cnt) pairs
Counter(dict(list_of_pairs)) # convert from a list of (elem, cnt) pairs
c.most_common()[:-n-1:-1] # n least common elements
c += Counter() # remove zero and negative counts
Implementation:
Program that demonstrates accessing of Counter elements
from collections import Counter
# Create a list
z = ['blue', 'red', 'blue', 'yellow', 'blue', 'red']
col_count = Counter(z)
print(col_count)
col = ['blue','red','yellow','green']
# Here green is not in col_count
# so count of green will be zero
for color in col:
print (color, col_count[color])
Output
Counter({'blue':3, 'red':2, 'yellow': 1})
blue 3
red 2
yellow 1
green 0
Applications
Counter() creates a hash-map for data containers which is very helpful than manual processing of the elements. It has high processing and functioning tools and can work with a wide range of data. In general, we can conclude that counter objects and its functions are used for primarily for processing large amounts of data.
Question:
Which of the following cannot be implemented for Counter objects?
With this article at OpenGenus, you must have the complete idea of Counter Objects in Python. Enjoy.