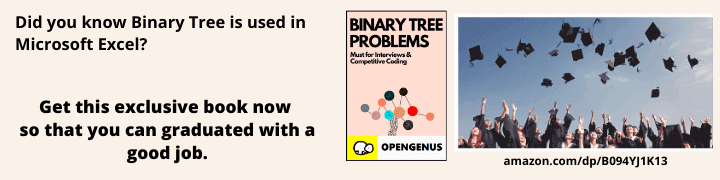
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article on OpenGenus, we will explore an introduction to building static web applications using Svelte framework.
Table of contents:
- Introduction
- Features of Svelte
- Installation
- Building your First App
- Quiz
Introduction
Svelte is an open-source frontend framework created by Rich Harris and is currently maintained and developed by a large group of developers. The main advantage is that it shifts a lot of the work that happens at runtime to compile-time, resulting in more efficient code. This, in turn, minimizes the need for runtime libraries, making the final application lightweight. Svelte follows a component-based architecture where components are defined using a combination of HTML, CSS, and JavaScript in a single file. Svelte has built-in support for animations, transitions, and server-side rendering, making it an excellent choice for developing fast, high-performance, visually appealing modern web interfaces.
Use Cases
- Svelte can be used for developing Single Page Applications(SPA), where the entire application is contained within a single HTML page.
- Svelte's efficient rendering capacity makes it the prime choice for developing high-performance applications.
- Svelte's performance and simplicity make it a great choice for creating real-time dashboards, data visualization tools, and interactive games.
- Svelte's component-based architecture makes it well-suited for creating reusable UI component libraries.
Advantages of Svelte
Performance
: Svelte's compilation approach shifts the workload to build time, resulting in more optimized and efficient runtime code compared to React, Angular, and Vue.Bundle Size
: Svelte's output generally exhibits smaller bundle sizes in contrast to applications built with React, Angular, and Vue. This leads to quicker loading times for users.Virtual DOM
: Unlike React, Svelte does not rely on a virtual DOM for updating the User Interface(UI).Build Process
: Svelte's compilation process streamlines the build pipeline and minimizes runtime overhead. This optimization culminates in enhanced performance compared to React, Angular, and Vue.
Choosing between Svelte and other frameworks/libraries depends on factors like application complexity, customization needs, future scalability, and time-to-market considerations. It is important to evaluate the pros and cons of each framework/library before making a decision.
Features of Svelte
Compiler
: Svelte's compilation approach shifts work to build time, resulting in optimized runtime code for better performance and smaller bundle sizes compared to other frameworks.Scope
: Svelte offers scoped styles for encapsulated component styling, promoting modular design and ease of maintenance.Transition
: Svelte includes transitions that can be easily applied to elements, creating smooth animations.SSR
: Svelte supports SSR(Server-Side Rendering) for improved initial load times.Reactivity
: Svelte's reactive system updates the real DOM directly, omitting the need for a virtual DOM.
Installation
To install Svelte for all operating systems one need to have Node JS installed.
Windows and macOS
: Download the Node JS installer from the official website and follow the installation instructions.Linux
: Use the package manager of your distribution to install Node JS.
sudo apt update
sudo apt install nodejs npm
After successful installation of Node JS, open your terminal or command prompt and run the following command to install the Svelte project template globally.
npm install -g degit
Building your First App
Introduction
We are going to create a simple svelte web application where we can create a TODO using a Form
svelte compnent and can view and delete it using a List
svelte component, and store initial data using svelte store with transitions.
Initialize App
- Run the following command to create a new Svelte app using the template.
degit sveltejs/template svelte-app
- Change your current directory to the newly created app directory.
cd svelte-app
- Inside the svelte-app directory, install the project dependencies using npm(Node Package Manager).
npm install
- Once the dependencies are installed, you can start the development server using the following command.
npm run dev
-
Open http://localhost:8080 in your browser to see the Svelte welcome page. As you make changes to your Svelte components, the app will automatically update in the browser.
-
You can start building your Svelte app by editing the components in the src directory. The entry point for your app is typically
src/main.js
.
Edit main.js
This file is the entry point of the Svelte application, where you initialize your Svelte app and attach it to the DOM.
import App from './App.svelte'
const app = new App({
target: document.body
})
export default app
- The above code initializes the application by importing the
App
component from App.svelte. It then creates an instance of this component, configuring it to render its output into thedocument.body
. The resulting app instance is then exported, enabling potential interaction with the Svelte application instance in future.
Create store.js
import { writable } from 'svelte/store'
export const DataStore = writable([
{
id: 1,
title: 'Default',
description: 'Default data from Svelte store'
}
])
- The above code defines a Svelte store named
DataStore
using the writable store from the svelte/store module. The store is exported to make it accessible to other parts of the application, where it can be modified. TheDataStore
holds an initial value, which is an array containing a single object with id, title, and description properties.
Edit App.svelte
<script>
import Form from "./Form.svelte"
import List from "./List.svelte"
let AppName = 'TODO'
</script>
<main>
<div class="form-container">
<Form {AppName} />
</div>
<div class="list-container">
<List />
</div>
</main>
<style>
.form-container {
padding-bottom: 20px
}
.list-container {
padding-top: 20px
}
</style>
-
script
: It imports theForm
andList
components from separate files and initializes a reactive variable namedAppName
with an initial value. -
main
: This HTML container holds the main content, encapsulating theForm
component with the AppName prop(property) and theList
component. -
style
: The CSS styles defined here apply spacing adjustments to the div elements.
Create Form.svelte
- The
Form
component is used to create a TODO with a title, description and a random id for access.
<script>
import { DataStore } from './store'
export let AppName
let title = ''
let description = ''
const handleSubmit = () => {
const newData = {
id: Math.random(),
title,
description
}
DataStore.update((currentData) => {
return [newData, ...currentData]
})
title = ''
description = ''
}
</script>
<div class="card">
<header>
<h2>Welcome to {AppName} App</h2>
</header>
<form on:submit|preventDefault={handleSubmit}>
<div class="input-group">
<input type="text" bind:value = {title} required placeholder="Title">
<input type="text" bind:value = {description} required placeholder="Description">
<button type="submit">Create</button>
</div>
</form>
</div>
<style>
.card {
background-color: #f9f9f9;
color: #333;
border-radius: 15px;
padding: 30px 40px;
margin: 20px 0;
box-shadow: 0px 2px 4px rgba(0, 0, 0, 0.1);
max-width: 400px;
margin: auto;
}
header h2 {
font-size: 24px;
font-weight: 600;
text-align: center;
margin-bottom: 20px;
}
.input-group {
display: flex;
flex-direction: column;
gap: 15px;
}
input {
border: 1px solid #ccc;
padding: 10px;
font-size: 16px;
border-radius: 5px;
}
button[type="submit"] {
background-color: #333;
color: #fff;
border: none;
padding: 8px 15px;
border-radius: 5px;
cursor: pointer;
font-size: 14px;
transition: background-color 0.3s ease;
}
button[type="submit"]:hover {
background-color: #555;
}
</style>
-
script
: The script section imports theDataStore
from the store.js file and exposes the AppName prop(property) for the component. It also initializes title and description reactive variables, and defines a handleSubmit function to update the store and clear input fields upon form submission. -
card
: The div represents a card element containing the component's content. It includes a header displaying the AppName, a form with input fields for title and description, and a button to submit the form. Theon:submit|preventDefault={handleSubmit}
attribute prevents the default form submission behavior. -
on:submit
: This attribute is an event listener in Svelte that triggers a function when the associated form is submitted. -
style
: The card class styles the card container, header h2 styles the header text, input-group styles the input container, input styles the input fields, and button[type="submit"] styles the submit button.
Create List.svelte
- The
List
component is used to display the list of TODOs with an option to delete a TODO from the store with custom transition effects.
<script>
import {DataStore} from './store'
import { fade, scale } from 'svelte/transition'
const handleDelete = (dataID) => {
DataStore.update((currentData) => {
return currentData.filter(data => data.id != dataID)
})
}
</script>
{#each $DataStore as data (data.id)}
<div in:scale out:fade="{{ duration: 500 }}" class="list-item">
<div class='card'>
<div class="title">
{data.title}
</div>
<button class="close" on:click={() => handleDelete(data.id)}>X</button>
<p class="text-display">
{data.description}
</p>
</div>
</div>
{/each}
<style>
.list-item {
margin-bottom: 20px;
}
.card {
background-color: #f9f9f9;
color: #333;
border-radius: 15px;
padding: 30px 40px;
margin: 20px 0;
box-shadow: 0px 2px 4px rgba(0, 0, 0, 0.1);
max-width: 600px;
margin: auto;
position: relative;
}
.title {
font-size: 22px;
font-weight: 600;
text-align: center;
margin-bottom: 10px;
}
.close {
position: absolute;
top: 10px;
right: 10px;
background: none;
border: none;
color: rgb(150, 150, 150);
font-size: 20px;
cursor: pointer;
}
@media (max-width: 600px) {
.card {
padding: 20px;
}
.title {
font-size: 18px;
}
.close {
top: 5px;
right: 5px;
font-size: 18px;
}
}
</style>
-
script
: This section imports theDataStore
from store.js file and the transition effectsfade and scale
from Svelte's transition module. The handleDelete function is defined to remove items from theDataStore
when the delete button is clicked. -
Each block
: This block iterates through each item in theDataStore
and assigns each item to the variable data. The (data.id) is used as a unique identifier for each iteration. -
Transition
: Thein:scale and out:fade
transitions are applied to smoothly animate entering and exiting elements. The duration property controls the animation speed. -
style
: It defines styles for the list items and the cards with media query for adjusting styles for smaller screens.
Output
Building the app
- When you're ready to deploy your app, you can create a production-ready build by running the following command.
npm run build
- This will create optimized files in the
public
directory that you can deploy to a web server.
Local Hosting
- The
serve
package is a command-line tool that allows you to serve static files from a local directory. Install the serve package globally using NPM(Node Package Manager).
npm install -g serve
- Navigate to the public directory.
cd public
- Start the server using the following command.
serve
- This will start a server at a specific URL (e.g., http://localhost:3000) where you can see your built Svelte app.