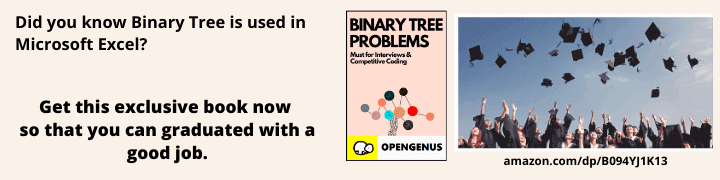
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article on OpenGenus, we will explore an introduction to building modern web applications using Ember JS.
Table of contents:
- Introduction
- Features of Ember JS
- Installation
- Building your First App
- Quiz
Introduction
Ember JS is an open-source JavaScript framework for developing web applications. It follows the Model-View-ViewModel (MVVM) architectural pattern and reduces the need for manual configuration and decision-making. Ember JS is used to increase the productivity and decrease the development duration of building a complex web application.
Use Cases
- Ember JS is used to build Single Page Applications(SPA) and Progressive Web Applications(PWA). Ember framework allows developers to create dynamic web applications.
- Ember JS can also be used for complex web applications that require a complete framework rather than a library. Ember can manage the complexity of large applications.
- Ember JS can be used when a large team of developers are involved in building the web application.
- Ember JS can be used when the projects require a built-in testing mechanism with scalability and maintainability.
Alternatives
-
React
: React is also a popular javascript library for building component based Single Page Applications(SPA). It is a library and uses a wide range of third-party libraries for various functionalities. -
Angular
: Angular is a full-fledged framework like Ember JS and provides a set of tools for building complex web applications, including data binding, dependency injection and a CLI. -
Vue JS
: Vue JS is a simple javascript framework that is easy to integrate into existing applications. Vue is suitable for building small and mdeium sized applications.
The choice between Ember and other frameworks/libraries depend on various factors like the project size, development team, flexibility, long-term needs and speed. It is important to evaluate the pros and cons of each framework/library before making a decision.
Features of Ember JS
Ember CLI
: It comes bundled with Ember JS and provides various commands to develop, build and test web applications.Components
: They are reusable UI elements that are fundamental building blocks of Ember JS applications.Routing
: Allows developers to define the structure and navigation of web applications.Services
: They provide a way to share data and state across different parts of the application.EmberData
: It provides a way to interact and manage data from external APIs and databases.Ember Inspector
: A browser extension to debug the web application live with various set of development tools.
Installation
To install Ember JS for all operating systems one need to have Node JS installed.
Windows and macOS
: Download the Node JS installer from the official website and follow the installation instructions.Linux
: Use the package manager of your distribution to install Node JS.
sudo apt update
sudo apt install nodejs npm
After successful installation of Node JS, one can install Ember JS with a single command using npm (Node Package Manager).
npm install -g ember-cli
Building your First App
Introduction
We will be developing a web application using Ember JS that will demonstrate the various features of the Ember JS:
-
Component
: A simple component which will be used to display the title and an array of features(strings). -
Route
: A route that will be used to access the above component. -
Service
: A service that will be injected into the component to provide the array of features in the specified route.
When the specified route is accesed by the user the component will be displayed with one part displaying features from an array in the route and other displaying the same features from an array in the service with a remove button for each feature. When the button is clicked the feature is removed from the array in the service.
Initialize App
- You can create a new Ember JS application using:
ember new ember-js --lang en
-
This command will create a new directory called
ember-js
and the--lang en
option sets the app's primary language to English. The application will include a development server, a template compilation, JavaScript and CSS minification and modern features via Babel. -
Change into the project directory using the command:
cd ember-js
- You can run the application using the command:
ember serve
- Open http://localhost:4200 in your browser to see the Ember JS welcome page.
Edit Template
- We can start by editing the application template that is currently being loaded. Open
app/templates/application.hbs
in your editor and change it to:
<h1>Welcome To Ember JS</h1>
{{outlet}}
- Ember detects the changes and reloads the page in the background automatically. The
{{outlet}}
defines that any route will be rendered in that place. Ember JS uses the handlebars template library to modify the app's user interface.
Create Route
- To create a new route in the application:
ember generate route my-route
-
The command creates a template to be displayed when the user visits
/my-route
with a route object and an entry into the applications routes. -
Open
app/templates/my-route.hbs
and add:
{{page-title "My-Route"}}
<h2>Features of Ember JS</h2>
- Open http://localhost:4200/my-route to see the contents inside the h2 tag in the
my-route.hbs
template right below the contents inside the h1 tag from theapplication.hbs
template. To add some data to render in themy-route
template editapp/routes/my-route.js
:
import Route from '@ember/routing/route';
export default class MyRouteRoute extends Route {
model() {
return ['Ember CLI', 'Components', 'Routes', 'Services', 'Ember Data', 'Ember Inspector'];
}
}
- The route's model() method returns data to the template. To convert the array of strings into HTML add
app/templates/my-route.hbs
:
{{page-title "My-Route"}}
<h2>Features of Ember JS</h2>
<ul>
{{#each @model as |feature|}}
<li>{{feature}}</li>
{{/each}}
</ul>
- The each helper is used to loop over each item in the array that is being returned from the model hook. Each item in the array will be rendered one by one. Each feature will be in the li (list) tag enclosed in a single ul (unordered list) tag.
Create Component
- Components are used when same UI elements appear multiple times in the application. The components can be called multiple times with different properties rather than repeating same lines of code multiple times. You can create new component named
List
using:
ember generate component list
- Edit the
List
component's templateapp/components/list.hbs
:
<h2>{{@title}}</h2>
<ul>
{{#each @feature as |feature|}}
<li>{{feature}}</li>
{{/each}}
</ul>
-
@title argument - defines the title
-
@feature argument - array of features
-
Replace the contents of
app/templates/my-route.hbs
with our new componentized version:
{{page-title "My-Route"}}
<h2>List of Features</h2>
<List
@title="Features"
@feature={{@model}}
/>
- A single component can be used in multiple pages to display the features with lesser number of lines and it reduces unnecessary load in the application.
Create Service
- You can create a new service named
FeatureService
by using the command:
ember generate service feature-service
- The command will create the
FeatureService
service. Services are also ember objects with own properties and methods.TheFeatureService
serviceapp/services/feature-service.js
manages a features array that represents the features:
import { A } from '@ember/array';
import Service from '@ember/service';
export default class FeatureServiceService extends Service {
features = A([
'Ember CLI',
'Components',
'Routes',
'Services',
'Ember Data',
'Ember Inspector',
]);
remove(feature) {
this.features.removeObject(feature);
}
}
-
The
A
is an utility provided by Ember JS to create arrays that are used to trigger automatic updates to the components when modified. A features array is created using theA
function and a remove() method that uses removeObject method from the array to remove an item. -
Inject the service into any object (component or service) to access using the
@ember/service
module. There are two ways to use this decorator. Either invoke it with no arguments, or pass it the registered name of the service. Add theFeatureService
service toapp/components/list.js
:
import Component from '@glimmer/component';
import { service } from '@ember/service';
export default class ListComponent extends Component {
@service featureService;
}
- We can add a remove action to the list component
app/components/list.js
:
import Component from '@glimmer/component';
import { service } from '@ember/service';
import { action } from '@ember/object';
export default class ListComponent extends Component {
@service('feature-service') data;
@action
remove(feature) {
this.data.remove(feature);
}
}
-
The
@action
decorator defines the remove action for the component. When triggered, this action calls the remove method on the injected data service, passing the feature as an argument. -
The service can also be used in a template after being injected into a component. Once injected into a component, a service can also be used in the template
app/components/list.hbs
:
<h2>{{@title}}</h2>
<ul>
{{#each @feature as |feature|}}
<li>{{feature}}</li>
{{/each}}
</ul>
<ul>
{{#each this.data.features as |feature|}}
<li>
{{feature}}
<button type="button" {{on "click" (fn this.remove feature)}}>Remove</button>
</li>
{{/each}}
</ul>
- The
data
service is being used to get data from the features. When the button is clicked, the corresponding feature will be removed from the array.