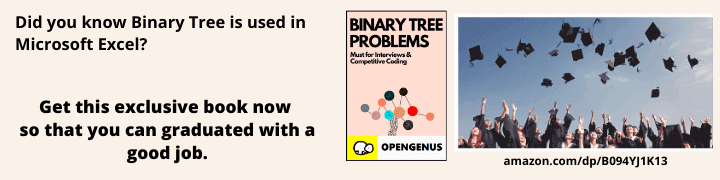
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In the world of web development, ECMAScript plays a crucial role as the standardized scripting language that powers modern web browsers. ECMAScript, often referred to as ES, is the official name for the language specification that popular implementations such as JavaScript are based on.
In this article at OpenGenus, we will explore the fundamental concepts of ECMAScript, its history, features, and its significance in web development. Whether you're a newbie or a seasoned developer, this article will provide you with a comprehensive understanding of ECMAScript and its role in building dynamic and interactive web applications.
Table of Contents
- History of ECMAScript
- ECMAScript vs JavaScript
- Key Features of ECMAScript
- ECMAScript Versions and Compatibility
- Working with ECMAScript: Browsers and Runtimes
- ES6 and Beyond: New Features and Syntax Enhancements
- ECMAScript Tools and Resources
- Conclusion
1. History of ECMAScript
ECMAScript traces its roots back to the mid-1990s when the web was gaining momentum. JavaScript, developed by Brendan Eich at Netscape Communications, became the de facto scripting language for web browsers. In an effort to standardize the language, the European Computer Manufacturers Association (ECMA) created a committee to define a language specification, resulting in the birth of ECMAScript in 1997.
Over the years, ECMAScript has evolved through multiple versions, each introducing new features and improvements. These versions include ES2 (1998), ES3 (1999), ES4 (abandoned), ES5 (2009), ES6/ES2015 (2015), ES2016, ES2017, ES2018, ES2019, ES2020, ES2021, and the latest, ES2022. The adoption of ECMAScript by different browser vendors has been instrumental in driving its growth and widespread usage.
2. ECMAScript vs JavaScript
ECMAScript and JavaScript are often used interchangeably, but it's important to understand the distinction between them. ECMAScript is the standardized scripting language, while JavaScript is the most popular implementation of that standard. JavaScript is built on top of ECMAScript and extends its capabilities with additional features and APIs.
One widely known implementation of ECMAScript is the V8 engine developed by Google. V8 is used in Google Chrome and several other browsers, providing high-performance execution of JavaScript code.
Sample Code Snippets
To give you a practical understanding of ECMAScript, let's explore some code snippets:
// Variable Declaration
let message = "Hello, ECMAScript!";
console.log(message);
// Arrow Function
const multiply = (a, b) => {
return a * b;
};
console.log(multiply(5, 3));
// Array Manipulation
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(num => num * 2);
console.log(doubledNumbers);
// Asynchronous Programming with Promises
function fetchData() {
return new Promise((resolve, reject
) => {
setTimeout(() => {
resolve("Data fetched successfully!");
}, 2000);
});
}
fetchData().then(data => {
console.log(data);
});
3. Key Features of ECMAScript
ECMAScript provides a rich set of features that enable developers to build powerful web applications. Some key features of ECMAScript include:
- Syntax and Data Types: ECMAScript provides a flexible syntax and supports various data types such as numbers, strings, booleans, objects, arrays, and more.
- Functions and Closures: Functions are first-class citizens in ECMAScript, allowing developers to create reusable and modular code. Closures provide the ability to capture and maintain access to variables within a specific scope.
- Prototypes and Object-Oriented Programming: ECMAScript follows a prototype-based object model, allowing objects to inherit properties and behavior from other objects. It enables developers to implement object-oriented programming patterns.
- Asynchronous Programming: ECMAScript provides features like Promises and async/await syntax, which simplify asynchronous programming and help handle asynchronous operations efficiently.
- Modules: ECMAScript introduced a standardized module system that allows developers to organize code into reusable and encapsulated modules. Modules enhance code maintainability and support the creation of large-scale applications.
- Arrow Functions: ES6 introduced arrow functions, a concise syntax for writing anonymous functions. They provide lexical scoping of the
this
keyword and offer a shorter syntax for one-liner functions.
These are just a few of the many features that ECMAScript offers, making it a versatile language for web development.
4. ECMAScript Versions and Compatibility
As mentioned earlier, ECMAScript has undergone several revisions and updates, each bringing new features and enhancements. However, not all browsers and runtimes immediately adopt the latest ECMAScript version, which poses compatibility challenges for developers.
To address this, tools like Babel have gained popularity. Babel is a JavaScript compiler that allows developers to write code using the latest ECMAScript syntax and then transpile it into an older version compatible with a wider range of browsers and environments.
Additionally, feature detection and polyfills can be used to ensure that specific language features are available and properly supported by the target environment.
5. Working with ECMAScript: Browsers and Runtimes
ECMAScript is primarily executed in web browsers, but it is also used in server-side environments such as Node.js. Web browsers provide an ECMAScript runtime environment known as the JavaScript engine. Popular JavaScript engines include V8 (used by Chrome and Node.js), SpiderMonkey (used by Firefox), and JavaScriptCore (used by Safari).
These engines parse and execute ECMAScript code, optimizing it for performance. They handle tasks such as memory management, garbage collection, and just-in-time (JIT) compilation.
Developers leverage ECMAScript to build interactive web applications by manipulating the Document Object Model (DOM) to modify and update web page content dynamically. ECMAScript's ability to respond to user interactions, manipulate data, and make HTTP requests allows developers to create rich and interactive user experiences.
6. ES6 and Beyond: New Features and Syntax Enhancements
ECMAScript 6, also known as ES2015, was a significant milestone that introduced numerous new features and syntax enhancements. Some of the key additions in ES6 include:
- Block-scoped Variables: The
let
andconst
keywords provide block-level scoping, reducing the need for relying solely on function-scoped variables (var
). - Arrow Functions: As mentioned earlier, arrow functions offer a concise syntax for writing functions and provide lexical scoping of
this
. - Classes: ES6 introduced a class syntax, enabling developers to define classes and use classical
inheritance and object-oriented programming concepts.
- Modules: The ES6 module system offers a standardized way to define and import modules, promoting code organization and reusability.
Since ES6, ECMAScript has been releasing new versions annually, introducing various features and enhancements. It's important for developers to stay up to date with these changes to leverage the latest language capabilities.
7. ECMAScript Tools and Resources
To enhance your ECMAScript development experience, various tools and resources are available:
- Babel: A JavaScript compiler that converts modern ECMAScript code into backward-compatible versions.
- ESLint: A widely used linter that helps enforce coding style guidelines and detect potential issues in ECMAScript code.
- MDN Web Docs: An authoritative resource that provides detailed documentation, guides, and reference materials on ECMAScript and web technologies.
- ECMAScript Compatibility Tables: These tables show the level of support for ECMAScript features across different browsers and environments.
8. Conclusion
ECMAScript, the language specification underlying JavaScript and other popular scripting languages, plays a vital role in modern web development. From its inception to the latest ECMAScript versions, this article has provided an overview of the language's history, key features, compatibility, and tools available to developers. Understanding ECMAScript is essential for building interactive and dynamic web applications. As the language continues to evolve, staying up to date with new features and syntax enhancements becomes increasingly important. Embrace ECMAScript, and embark on a journey of creating cutting-edge web experiences.