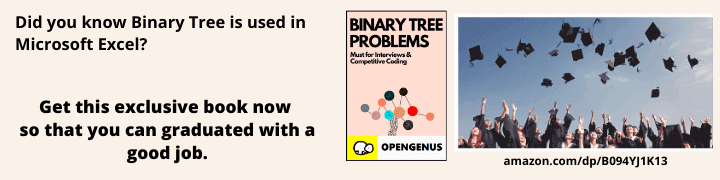
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In the early days of the web, audio playback relied on plugins like Flash. However, the introduction of the <audio>
element in HTML5 and the Web Audio API changed the game, eliminating the need for plugins and offering developers a robust toolset for creating dynamic audio experiences directly within web applications.
Table of contents:
- Core Concepts
- Practical Use Cases
- Web Audio Api Demo
- Web Audio API Features
- Key Takeaways (Web Audio API)
Core Concepts:
- AudioContext:
- At the core of the Web Audio API is the AudioContext, a vital interface that represents the audio-processing graph. To set the stage, you create an AudioContext using the following snippet:
// Taking in mind you have a button with id "startButton"
const startButton = document.getElementById('startButton');
startButton.addEventListener('click', function() {
// Create AudioContext here
const audioContext = new (window.AudioContext || window.webkitAudioContext)();
// audio processing code goes here
});
- Audio Nodes:
- Audio nodes are the building blocks of the Web Audio API. They come in various topics, such as source nodes(like
AudioBufferSourceNode
for pre-loaded audio), processing nodes(e.g,GainNode
for volume control), and destination nodes (typically the speakers or headphones).
- Audio Buffer:
- The Audio Buffer is a crucial data structure used to store and manipulate audio data. Paired with an AudioBufferSourceNode, it facilitates the playback of audio files.
Practical Use Cases:
- Audio Playback:
- Let's start with a fundamental use case- playing audio. Below is a simple example that demonstrates loading and playing an audio file
const audioContext = new(window.AudioContext || window.webAudioContext)();
const source =
audioContext.createBufferSource();
//Fetch and decode the file('path/to/audio.mp3')
.then(response => response.arrayBuffer())
.then(data => audioContext.decodeAudioData(data))
.then(data => audioContext.decodeAudioData(data))
.then(buffer =>{
source.buffer = buffer;
source.connect(audioContext.destination);
source.start();
})
.catch(error => console.error('Error loading audio:',error));
- Real Time Audio Processing:
- The Web Audio API empowers real-time processing of audio data, enabling the creation of dynamic audio applications. Consider this example of a simple real-time volume control using the GainNode:
- The Web Audio API empowers real-time processing of audio data, enabling the creation of dynamic audio applications. Consider this example of a simple real-time volume control using the GainNode:
const audioContext = new (window.AudioContext || window.webkitAudioContext)();
const source = audioContext.createBufferSource();
const gainNode = audioContext.createGain();
// Connecting nodes
source.connect(gainNode);
gainNode.connect(audioContext.destination);
// Setting initial gain value
gainNode.gain.value = 0.5;
// Updating gain dynamically
document.addEventListener('input', event => {
const { value } = event.target;
gainNode.gain.value = parseFloat(value);
});
// Play audio
source.start();
Let us create demo of the same with information we have:
HTML:
<html>
<head>
<title>Web Audio API </title>
</head>
<body>
<div class="container">
<h1>Web Audio API </h1>
<button id="startButton">Start Playback</button>
</div>
</body>
</html>
Defines a simple HTML structure with title, heading, and a button with id"startButton" which will intiate the program
CSS:
body {
font-family: 'Arial', sans-serif;
background-color: #f0f0f0;
margin: 0;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
}
.container {
text-align: center;
}
h1 {
color: #333;
}
button {
padding: 10px 20px;
font-size: 16px;
cursor: pointer;
background-color: #4caf50;
color: white;
border: none;
border-radius: 5px;
margin-top: 20px;
}
button:hover {
background-color: #45a049;
}
Applies styling to the HTML elements for a centered and visually appealing layout.
Javascript:
document.addEventListener('DOMContentLoaded', function() {
const startButton = document.getElementById('startButton');
let audioContext;
startButton.addEventListener('click', function() {
if (!audioContext) {
// Create AudioContext if not already created
audioContext = new (window.AudioContext || window.webkitAudioContext)();
}
// Create an audio buffer source
const source = audioContext.createBufferSource();
// Fetch and decode audio file
fetch('path/to/audio.mp3')
.then(response => response.arrayBuffer())
.then(data => audioContext.decodeAudioData(data))
.then(buffer => {
// Set the buffer and connecting to the output
source.buffer = buffer;
source.connect(audioContext.destination);
// Start playback
source.start();
})
.catch(error => console.error('Error loading audio:', error));
});
});
Image of the demo:
Explanation:
-
This code snippet creates a basic HTML page with a button styled using CSS. When the button is clicked, it triggers JavaScript code utilizing the Web Audio API to load and play an audio file
- In button click, it checks if an AudioContext is created and creates one if not.
- It then creates an AudioBufferSourceNode, fetches and decodes an audio file, connects it to the audio context, and starts playback.
- Replace 'path/to/audio.mp3' with the actual path to your audio file.
Web Audio API Features:
-
Modular Routing:
- The API supports modular routing, allowing arbitrary connections between different AudioNode objects. This flexibility enables developers to create complex audio functions with dynamic effects.
-
32-bit Floats:
- High dynamic range processing is achieved through 32-bit floats, ensuring accurate and detailed audio representation.
-
Sample-Accurate Playback:
- The API enables sample-accurate scheduled sound playback with low latency, ensuring precise timing.
-
Automation of Audio Parameters:
- Audio parameters can be automated, providing a means for dynamic manipulation and effects.
-
Integration with WebRTC and HTMLMediaElement:
- Seamless integration with other web technologies like WebRTC and HTMLMediaElement enhances the overall capabilities of the API.
-
Synthesis and Processing using JavaScript:
- The API allows synthesis and processing of audio directly using JavaScript, providing developers with a high degree of control.
Question
What capability does the Web Audio API offer for precise timing of sound playback?
Question
Which feature of the Web Audio API allows for modular routing and arbitrary connections between different AudioNode objects?
Key Takeaways (Web Audio API)
- Understanding the foundational elements of the Web Audio API—AudioContext, Audio Nodes, and Audio Buffer—is crucial for building dynamic audio functionality.
- The article provides practical insights into using the Web Audio API for audio playback and real-time processing. Examples include loading and playing audio files, as well as implementing real-time volume control.
- The Web Audio API offers powerful features such as modular routing, 32-bit Floats for high dynamic range processing, sample-accurate playback with low latency, automation of audio parameters, seamless integration with WebRTC and HTMLMediaElement, and the ability to synthesize and process audio directly using JavaScript..