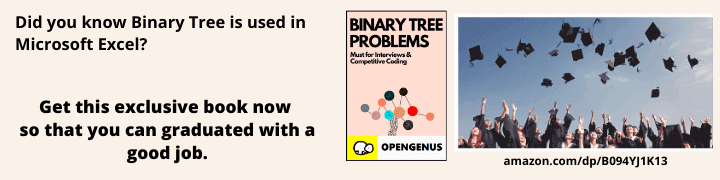
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will be discussing about "Bus Error In C++" and SIGBUS signal in detail.
Contents:
- Introduction to Bus Error
- Main causes of Bus Errors
- Non-existent address
- Unaligned access
- Paging errors
- Program Code
- Explanation
- Quiz
Introduction
When a process attempts to access memory that the CPU cannot physically address, hardware will raise an error to alert the operating system which is known as a bus error.
The bus error also known as SIGBUS is typically signal 10. The acronym SIGBUS stands for "bus error."
In other words, "bus error" means trying to access memory that does not exist.
For Example
if you attempt to access a 12G address but only have 8G of memory.
Or
if you exceed the limit of usable memory.
The following factors affect bus error:
1 . Non-existent address
2 . Unaligned access
3 . Paging errors
1 . Non-existent address
The software gives the CPU instructions to read from or write to a particular physical memory address.
The CPU then assigns this physical address to its address bus and instructs all other hardware to respond in a certain way.
If no response is received from hardware, then the CPU raises an exception that the physical address is unknown or non-existent.
2. Unaligned access
In most CPUs, each memory address corresponds to a single 8-bit byte.
In general, CPUs can access individual bytes from each memory address, but cannot access larger units unless they are "aligned" to a specific boundary.
trying to read a multi-byte value (such as an int, 32-bits) from an odd address generated a bus error.
A 16-bit alignment requirement for multi-byte access would mean that addresses 0, 4, 8, 12, and so on would be aligned and therefore accessible, but all other addresses are unaligned.
A 32-bit alignment requirement for multi-byte access would mean that addresses 0, 4, 8, 12, and so on would be aligned and therefore accessible, but all other addresses are unaligned.
3. Paging errors
When virtual memory pages cannot be paged in or newly created memory is physically allocated because the disc is full, FreeBSD, Linux, and Solaris can raise a bus fault.
Bus error can also result from the operating system's failure to back a virtual page with virtual memory at least on Linux.
Program Code 1
#include <signal.h>
int main(void)
{
raise(SIGBUS);
return 0;
}
Output
Bus error
Explanation:
In this case, it raise a bus error.
Program Code 2
#include <signal.h>
int main()
{
char *value = 0;
*value = 'X';
}
Output
Bus error
Explanation:
In this case, it raise a bus error.This program is tested on PowerPC Mac .
On modern hardware, it results segmentation fault instead of a bus error.
Program Code 3
#include<stdio.h>
#include<string.h>
int main()
{
char *c="Hello World";
printf("\n Normal String = : %s\n",c);
int i,j;
char temp;
int len=strlen(c);
//print Reverse String
for(i=0,j=len-1;i<=j;i++,j--)
{
temp=c[i];
c[i]=c[j];
c[j]=temp;
}
printf("\n reversed string is : %s\n\n",c);
}
Output
Normal String = : Hello World
Bus Error
Explanation:
In this case,char *str = "Hello Wolrd" is treated as literal. It is the same as const char *str , a constant to string pointer, and attempting to modify a constant string is not enabled.As as a result it raise a bus error.
Program Code 4
#include<stdio.h>
#include<string.h>
int main(void)
{
char *str;
str="Hello World";
printf("\n Normal String = : %s\n",str);
int i,j;
char temp;
int len=strlen(str);
char *ptr=NULL;
ptr=malloc(sizeof(char)*(len));
ptr=strcpy(ptr,str);
for (i=0, j=len-1; i<=j; i++, j--)
{
temp=ptr[i];
ptr[i]=ptr[j];
ptr[j]=temp;
}
printf("Reverse String = : %s\n",ptr);
}
Output
Normal String = : Hello World
Reverse String = : dlroW olleH
Explanation:
In this case ,we used malloc() and created a copy of the original string. here ,ptr is not of the 'const char *' type (constant) , so modifiying will not raise any errors. As a result, the reverse string is printed.