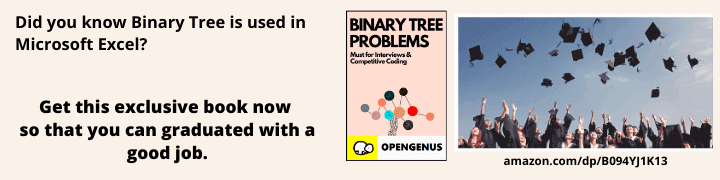
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will develop a calendar console application which generates the calendar for the present year and it can generate calendar for year that we need. It will be implemented in JAVA.
This article will guide you through the process of creating a simple calendar application in Java. The article will cover the basics of working with the Calendar class, how to format the output, and how to highlight the current date.
Audience: This article is intended for beginner to intermediate Java developers who want to learn how to create a calendar application. The article assumes that the reader has a basic understanding of Java programming concepts.
Introduction
- Calendars play a crucial role in our daily lives, helping us keep track of important dates, appointments, and events.
- With the increasing use of technology, there is a need for a simple and user-friendly calendar that can be easily accessed and used.
- The code provided above is a simple Java program that generates a calendar for a given year.
Features
- User-friendly interface: The program prompts the user to enter the year for which the calendar is to be generated, making it easy to use.
- Color coding: The program uses color coding to indicate the current day and days in the past, making it easier to identify important dates.
- Considers current date and time: The program takes into account the current date and time to accurately display the current day.
Design and Approach
Design:
- The CalendarGenerator class uses the java.util.Calendar and java.time.LocalDate classes to generate the calendar for a specified year.
- The LocalDate class is used to get the current date, extract the year, month, and day information, and check whether a specific date is the current date.
- The Calendar class is used to set the year, month, and day of the month, get the day of the week for the first day of the month, get the last day of the month, and move to the next day.
Approach:
- The CalendarGenerator class has a static method called generateCalendar that takes an integer as an argument, which represents the year for which the calendar should be generated.
- The generateCalendar method uses a for loop to iterate through each month of the year. For each month, the names of the days of the week are printed, followed by the days of the month.
- If a day is today's date, it is printed in green. If a day is in the past, it is printed in red. Otherwise, the day is printed in the default color.
Implementation
import java.util.*;
import java.time.*;
public class CalendarGenerator {
static String[] months = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"};
/**
* This method generates a calendar for a given year.
* @param year The year for which the calendar should be generated
*/
public static void generateCalendar(int year) {
LocalDate currentDate = LocalDate.now();
int currentYear = currentDate.getYear();
int currentMonth = currentDate.getMonthValue();
int currentDay = currentDate.getDayOfMonth();
Calendar calendar = Calendar.getInstance();
calendar.set(Calendar.YEAR, year);
for (int i = 0; i < 12; i++) {
System.out.println("\n" + months[i] + " " + year);
System.out.println("Sun\tMon\tTue\tWed\tThu\tFri\tSat");
calendar.set(Calendar.MONTH, i);
calendar.set(Calendar.DAY_OF_MONTH, 1);
int dayOfWeek = calendar.get(Calendar.DAY_OF_WEEK);
for (int j = Calendar.SUNDAY; j < dayOfWeek; j++) {
System.out.print("\t");
}
int lastDay = calendar.getActualMaximum(Calendar.DAY_OF_MONTH);
for (int j = 1; j <= lastDay; j++) {
if (year == currentYear && i == currentMonth - 1 && j == currentDay) {
System.out.print("\033[32m[" + j + "]\033[0m\t");
} else if (year < currentYear || (year == currentYear && i < currentMonth - 1) || (year == currentYear && i == currentMonth - 1 && j < currentDay)) {
System.out.print("\033[31m" + j + "\033[0m\t");
} else {
System.out.print(j + "\t");
}
if (calendar.get(Calendar.DAY_OF_WEEK) == Calendar.SATURDAY) {
System.out.println();
}
calendar.add(Calendar.DAY_OF_MONTH, 1);
}
}
}
public static void main(String[] args) {
Calendar calendar = Calendar.getInstance();
int year = calendar.get(Calendar.YEAR);
generateCalendar(year);
Scanner sc = new Scanner(System.in);
String YEAR;
System.out.println("\nEnter any year (YYYY): or ('q' for exit)");
while(( YEAR = sc.next()) != null){
if(YEAR.equals("q")) break;
generateCalendar(Integer.parseInt(YEAR));
System.out.println("\nEnter any year(YYYY): or ('q' for exit)");
}
sc.close();
}
}
The Above code implements a Calendar Generator in Java:
Import statements:
import java.util.*;
import java.time.*;
- In the first line, the java.util package is imported, which contains the Calendar class that is used to generate a calendar for a specified year.
- In the second line, the java.time package is imported, which contains the LocalDate class that is used to get the current date.
Definition of the CalendarGenerator class:
public class CalendarGenerator {
// An array to store the names of months
static String[] months = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"};
- The CalendarGenerator class is defined. The months array stores the names of the months that will be used to display the calendar.
Definition of the generateCalendar method:
/**
* This method generates a calendar for a given year.
* @param year The year for which the calendar should be generated
*/
public static void generateCalendar(int year) {
// Get the current date and extract year, month, and day information from it
LocalDate currentDate = LocalDate.now();
int currentYear = currentDate.getYear();
int currentMonth = currentDate.getMonthValue();
int currentDay = currentDate.getDayOfMonth();
// Get a Calendar instance set to the specified year
Calendar calendar = Calendar.getInstance();
calendar.set(Calendar.YEAR, year);
- The above code is defining a method called "generateCalendar" that takes an integer "year" as input.
- The first step of the method is to get the current date and extract information about the year, month, and day from it using the LocalDate class in Java.
- The extracted information is stored in the variables "currentYear", "currentMonth", and "currentDay".
- Next, a Calendar instance is created by calling Calendar.getInstance(), and the year is set to the input year using the calendar.set method.
- The Calendar instance will be used later in the code to manage and manipulate the dates.
Loop through each Month:
// Loop through each month
for (int i = 0; i < 12; i++) {
System.out.println("\n" + months[i] + " " + year);
System.out.println("Sun\tMon\tTue\tWed\tThu\tFri\tSat");
// Set the calendar to the current month
calendar.set(Calendar.MONTH, i);
calendar.set(Calendar.DAY_OF_MONTH, 1);
// Get the day of the week for the first day of the month
int dayOfWeek = calendar.get(Calendar.DAY_OF_WEEK);
// Loop through the days of the week until we reach the first day of the month
for (int j = Calendar.SUNDAY; j < dayOfWeek; j++) {
System.out.print("\t");
}
// Get the last day of the month
int lastDay = calendar.getActualMaximum(Calendar.DAY_OF_MONTH);
It performs the following operations:
- The code uses a for loop to loop through each month of the year. The loop variable i starts from 0 and goes up to 11, representing the 12 months of the year.
- Within the loop, it prints the name of the month and the year. For example, if i is 0, it would print "January 2023".
- The code uses another for loop to print the days of the week (Sunday to Saturday) that act as headings for the calendar.
- The code then sets the calendar to the current month being processed in the loop.
- This is done by calling the set method of the Calendar object and passing in Calendar.MONTH and i as arguments.
- The code then sets the day of the month to 1, which is the first day of the current month, by calling the set method of the Calendar object and passing in Calendar.DAY_OF_MONTH and 1 as arguments.
- The code gets the day of the week for the first day of the month by calling the get method of the Calendar object and passing in Calendar.DAY_OF_WEEK as an argument. This value is stored in the dayOfWeek variable.
- The code then uses another for loop to print tabs until it reaches the day of the week of the first day of the month. This is done to align the first day of the month with the corresponding day of the week heading.
- The code gets the last day of the month by calling the getActualMaximum method of the Calendar object and passing in Calendar.DAY_OF_MONTH as an argument. This value is stored in the lastDay variable.
Loop through each Day:
// Loop through each day of the month
for (int j = 1; j <= lastDay; j++) {
// If the current date is today, print it in green
if (year == currentYear && i == currentMonth - 1 && j == currentDay) {
System.out.print("\033[32m[" + j + "]\033[0m\t");
// If the current date is in the past, print it in red
} else if (year < currentYear || (year == currentYear && i < currentMonth - 1) || (year == currentYear && i == currentMonth - 1 && j < currentDay)) {
System.out.print("\033[31m" + j + "\033[0m\t");
// Otherwise, print it in the default color
} else {
System.out.print(j + "\t");
}
// If the day is Saturday, start a new line
if (calendar.get(Calendar.DAY_OF_WEEK) == Calendar.SATURDAY) {
System.out.println();
}
// Move to the next day
calendar.add(Calendar.DAY_OF_MONTH, 1);
}
}
The above code is a nested for loop that loops through each day of the month.
- For each day, it checks if the day is the current day.
- If it is, it prints the day with a green color.
- If the day is in the past, it prints the day in red.
- If the day is not the current day or in the past, it prints the day in the default color.
- The code also checks if the current day is a Saturday. If it is, it starts a new line to ensure that the calendar is formatted correctly.
- Finally, it moves to the next day by using the calendar.add method, which adds a specified amount of time to the calendar object.
Definition of main() method:
public static void main(String[] args) {
// creates an instance of the Calendar class
Calendar calendar = Calendar.getInstance();
// and retrieves the current year
int year = calendar.get(Calendar.YEAR);
// calls the generateCalendar method and passes the current year as an argument.
generateCalendar(year);
// creates a Scanner object
Scanner sc = new Scanner(System.in);
String YEAR;
// asks the user to enter a year
System.out.println("\nEnter any year (YYYY): or ('q' for exit)");
// while loop will continue until the user enters 'q' or the input is null
while(( YEAR = sc.next()) != null){
if(YEAR.equals("q")) break;
// call the generateCalendar method and pass the entered year as an argument.
generateCalendar(Integer.parseInt(YEAR));
System.out.println("\nEnter any year(YYYY): or ('q' for exit)");
}
// Scanner object is closed to prevent resource leaks
sc.close();
}
- The main method of the program creates a Calendar instance and retrieves the current year.
- It then calls the generateCalendar method and passes the current year as an argument.
- It then creates a Scanner object and asks the user to enter a year.
- The program continues to prompt the user to enter a year until the user enters 'q' or the input is null.
- If the user enters a year, the generateCalendar method is called and passed the entered year as an argument.
- The Scanner object is closed at the end of the program to prevent resource leaks.
Look at the example Output
- It is generated on 14-02-2023.
Further Improvements
- Exception handling: The code does not include any exception handling. For example, if the user enters an invalid year or non-integer input, an exception will be thrown. You should add try-catch blocks to handle these exceptions.
- Code reuse: The code could be made more reusable by breaking it up into smaller methods. For example, the code for generating the calendar could be separated from the code for user input and interaction.
- Another improvement could be to add the ability to display the calendar for multiple years on a single page.
- Adding a GUI (Graphical User Interface) can greatly improve the user experience of this Java application.
Conclusion
- In conclusion, the CalendarGenerator Java application is a useful tool for generating calendars for any given year.
- It uses the java.util and java.time libraries to retrieve the current date, and allows the user to enter a year of their choice.
- The program then generates a calendar for that year, with the current date highlighted in green, past dates in red, and future dates in the default color.
- The code uses a Calendar instance and loops through each month to print the name of the month, the days of the week, and the dates.
- The last day of the month is calculated and used to determine when to start a new line.
- The program continues to run until the user enters "q" or the input is null.
With this article at OpenGenus, you must have the complete idea of how to develop a Calendar application in Java.