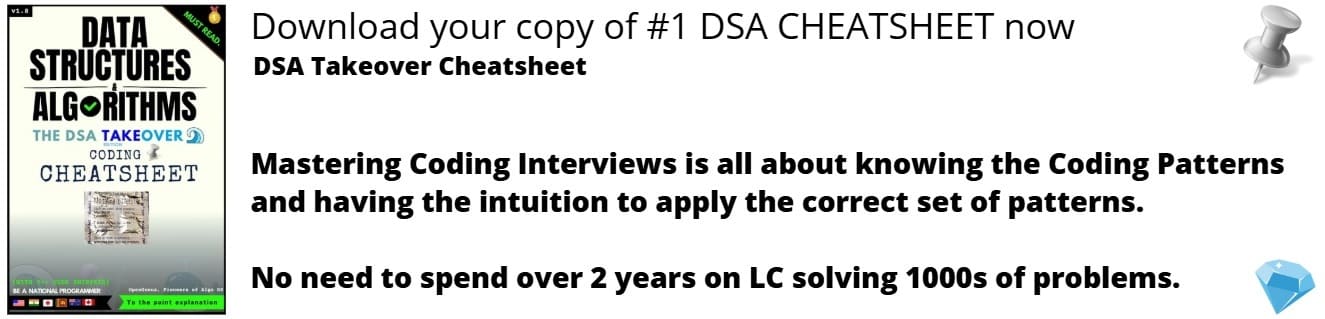
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will discuss about various types of errors that occurs in the compiler design and what are those methods with the help of which this error can be recovered in a compiler.
Table of contents:
- Introduction to Error in Compiler Design
- Types of errors
- Error recovery methods
- Summary Table
In short:
Errors | Recovery methods |
---|---|
Lexical phase errors | Panic mode |
Syntactic phase errors | Panic, statement mode, error production , global correction |
Semantic errors | Symbol table |
Prerequisite: Different Code Optimizations in Compiler Design
So let's start.
Introduction to Error in Compiler Design
Error is an operation committed by the user which definitely cause an abnormal change or problems in a program. It is very important and necessary to recover these errors as fast as you can because if they are not recovered in a proper time it will lead to a situation from where it becomes very difficult to come out. Generally in a compiler error occurs when it fails to compile a piece of code or in any algorithm which happens due to error in a code or due to some error in compiler itself. So we will first see some errors in compiler and after discussing that we will straight move towards the recovery strategies that need to be applied to recover the errors in a compiler.
In the phase of compilation or when we are compiling our code or program, the errors that we commit or an error that is committed by any user who is writing a code, are sent to the user in the form of messages or error messages. This is error handling process. Error handler performs all the functions that are supposed to be performed while detecting the errors in a compiler. Those functions are :
- Error detection: Error handler detects the error during compilation.
- Error reporting: It reports the error detected to the user in the form of error messages.
- Error recovery: It also helps in error recovery.
Types of errors
Now we will see some types of errors that occurs generally. Basically errors are of two types.
-
Compile time errors
-
Runtime errors
-
Logical errors
-
Compile time errors: These are the errors which occur while compiling a program and they occur due to incorrect syntax , like for instance when we miss a semicolon at the end of a program or when we miss putting brackets at the end of any for loop or any conditional statement. Example:
#include <stdio.h>
void main(){
int a,b;
scanf("%d %d",&a,&b);
int sum=a+b;
printf("%d",sum) //semicolon missed which will result in compile time error
}
After compiling the output will look like this:
error: expected ';' before '}' token
- Runtime errors: These are the type of errors which occurs during execution of a program. These are the errors which arise due to user putting any invalid syntax in a code or any piece of code which a normal compiler is unable to execute. Example:
#include <stdio.h>
void main(){
int a,b;
scanf("%d %d",&a,&b);
int sum=a+b;
int avg=sum/0; //we are dividing by zero which is invalid
printf("%d",avg);
}
This code will not execute as it contains an invalid syntax. Output looks like:
warning: division by zero [-Wdiv-by-zero]
avg = sum/0;
3.Logical errors: These are the types of errors in which the given code is unreachable or there is a presence of an infinite loop.
Now we will the see the different types of compile time errors.
1.Lexical phase errors
2.Syntactic phase errors
3.Semantic errors
1.Lexical phase errors: These errors occurs during lexical phase and during the execution of a program. Lexical error occurs when some sequence of characters does not match the pattern of any token. These errors can be due to spelling errors or due to appearance of any illegal character. Example:
#include <stdio.h>
void main(){
int a,b;
scanf("%d %d",&a,&b);
int sum=a+b;
printf("%d",sum);**@**
}
The above code produces a lexical error due to presence of an illegal character after printf statement(in bolder part).
2.Syntactic phase errors: These errors occurs during syntax phase and during the execution of a program. These errors arise due to parenthesis unbalancing or when some operators are missing. Example:
#include <stdio.h>
void main(){
int a,b;
scanf("%d %d",&a,&b);
int sum=a+b;
if(sum **=** 200){
printf("%d",sum);
}
else{
break;
}
}
The above code produces a syntactic or syntax error because in the if statement we require the use of == but we are using = .
3.Semantic errors: These errors occurs during semantic analysis phase are detected during compile time of a program. These errors occurs due to wrong use of operators,variables or when there are undeclared variables. Example:
#include <stdio.h>
void main(){
int a;
scanf("%d",&a);
int sum=a+**b**;
printf("%d",sum);
}
The above code produces a semantic error because b variable was not declared.
Now after having a clear understanding of the types of errors we will see some error recovery strategies in a compiler.
Error recovery methods
Different Error recovery methods in Compiler Design are:
- Panic mode recovery
- Statement mode recovery
- Error productions
- Global correction
- Using Symbol table
Let us dive deeper in each type.
Panic mode error recovery
1.Panic mode recovery: This is the most basic and easiest way of error recovery. In this as soon as the parser detects an error anywhere in the program it immediately discard the rest of the remaining part of code from that particular place where error is found by not processing it.
Advantage:
a. It is the most basic method and very easy to implement.
b. It never goes in an infinite loop.
Disadvantage:
a. A huge amount of input is discarded without processing that and it does not detect any more errors as it is not processing it further.
Lexical phase errors and syntactic phase errors are recovered by panic mode recovery.
Statement mode error recovery
-
Statement mode recovery: In this method as soon as parser detects an error it performs recovery by correcting the remaining input so that remaining input statement allow parser move further. In this correction includes removing extra semicolons etc.
Syntactic phase errors are recovered by statement mode recovery.
Error productions
-
Error productions: If a compiler designer has some knowledge of errors that are very common then these type of errors are recovered by increasing the grammar with error production that produce incorrect constructs. It is very difficult and problematic to maintain.
Syntactic phase errors are recovered by error productions.
Global corrections
-
Global correction: In this method the parser judge or considers the full program and try to find the closest matching of this which is free from errors. When an incorrect statement is tackled it creates a parse tree for closest error free statement or a piece of code. The biggest problem in this method is that it has a very high complexity( both time and space) due to which it is not implemented in practical purposes.
Syntactic phase errors are recovered by global correction.
Using Symbol table
- In semantic errors, errors are recovered by using a symbol table for corresponding identifier and if data types of two operands are incompatible , automatically type conversion is done by the compiler.
Consider this case:
String data1 = "2";
int data2 = "5";
int data3 = data1 + data2;
This results in a Parse tree like:
(+) (int + int)
/ \
/ \
/ \
data1 (string) data2 (int)
data2 should be corrected by converting it into a string and then, the two strings are appended. This statement has an error but compiler makes assumptions and resolves it.
Summary Table
Errors | Recovery methods |
---|---|
Lexical phase errors | Panic mode |
Syntactic phase errors | Panic, statement mode, error production , global correction |
Semantic errors | Symbol table |
So now we have seen some error recovery methods with the help of which you can easily recover all types of errors that we have discussed above. So now I would like to conclude this article by hoping that you all will definitely try to learn this topic by taking the help of this article.
Thank you.