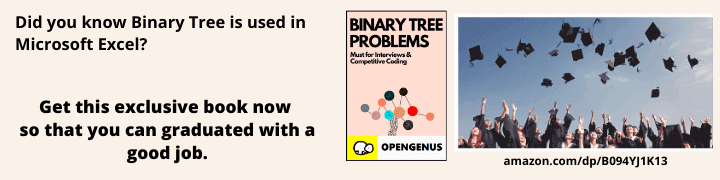
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 15 minutes | Coding time: 2 minutes
for loop is an entry controlled loop that is widely used in Java programming language.
Loop is a programming concept which is natively supported by nearly all programming languages. Loops are used to repeat a particular coding task where each repetition follows a particular pattern which can be incorporated in a code. Example of such a task is printing integers from 1 to 10. The repetition is that we are printing integers and the pattern is that we start from 1 and increment by 1 each step. In this article, we will take a look at loops in Java.
The concept of loops comes into picture when we need to implement/print a particular block/statement more than one time till a particluar condition is specified. We have different types of loops :
-
Entry Controlled loop : Where the entry of the loop is controlled that is we have a condition at the starting of the loop. Example : while loop
-
Exit Controlled loop : In this type of loop the exit from the loop is controlled that is we have a condition at the end of the loop. Example : do..while loop
In this particular section we are going to discuss for loop
for loop
Syntax :
for (initializationStatement; testExpression; updateStatement)
{
// codes
}
Where :
-
initializationStatement is the statement where we to initialize the loop counter to some value. Example : int i=0;
-
testExpression in this expression we have to test the condition. If the condition is to true then we will execute the body of loop otherwise we will exit from the for loop. Example : i<=10;
-
updateStatement after executing loop body this statement is encountered and is incremented/decremented depending on the statement. Example : i++
Order of the excution of for loop
-
First we intialize the loop counter in Java one have to declare the variable before using it or it can be declared as well as intialized in the intializationStatement as well.
-
Then the condition is checked i.e testExpression is evaluated.
-
After this we enter the loop and print/execute the statement(s)/block.
-
Then the value of the loop counter in incremented/decremented depending on the updateStatement.
-
Now we will repeat Step 2-4 till the testExpression condition is violated.
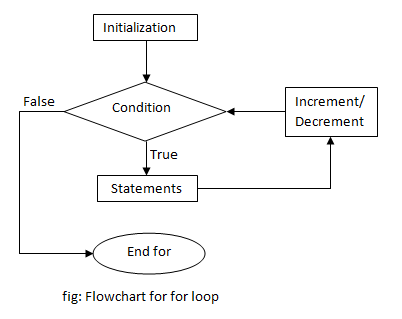
Example
In this example, we will write a program to print "OpenGenus" five times with number from 1 to 5 with it.
Answer :
class for_loop
{
public static void main (String[] args)
{
for(int i=1; i<=5; i++)
{
System.out.println("OpenGenus "+i);
}
}
}
Points to remember
- It is not compulsory to specify initializationStatement or testExpression or UpdateStatement during declaration of for loop.
Example:
class for_loop
{
public static void main (String[] args)
{
int i=2;
// Without Initialization Statement
for(; i<=5; i++)
{
System.out.println("OpenGenus "+i);
}
// Without Test expression
for(i=1; ;i++)
{
System.out.println("OpenGenus "+i);
if(i==5)
break;
}
// Without Update Statement
for(i=4; i<=5;)
{
System.out.println("OpenGenus "+i);
i++;
}
}
}
- You can declare updatStatement inside the block also.
Example :
class for_loop
{
public static void main (String[] args)
{
int i=2;
// With Update Statement inside block
for(i=4; i<=5;)
{
System.out.println("OpenGenus "+i);
i++;
}
}
}
- One can include more than one initializationStatement or testExpression or updateStatement during declaration of for loop this can be done with the help of logical operations such as AND(&&) / OR(||).
class for_loop
{
public static void main (String[] args)
{
for(int i=4, j=2; i<=6 && j>=0; i++, j--)
{
System.out.println("OpenGenus "+i +" "+ j);
}
}
}
-
You should always declare the loop with small case i.e for and not FOR/For/FoR...etc as Java is a case sensitive langauage and this true for all other loops as well
-
Infinite loop
To create any inifinite loop, you need to ensure that the test condition never evaluates to false.
Example:
class for_loop
{
public static void main (String[] args)
{
// Infinite loop
for(;;)
{
}
}
}