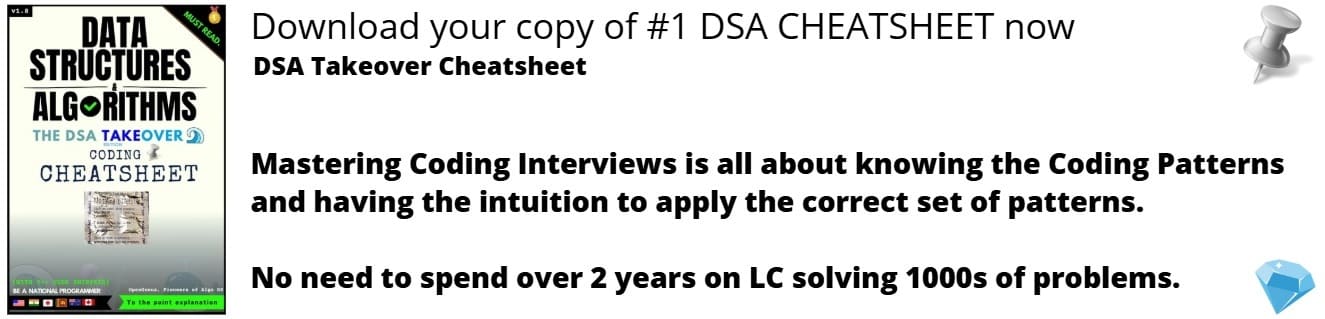
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
This article describes the fundamental parts of schemas and how to create one.
GraphQL schemas are what GraphQL servers use to describe your data. The scheme defines a tree of types with fields that a data store then fills. They also specify what queries and clients can use mutations.
Contents:
- What is GraphQL
- Type language
- Schema definition language (SDL)
- Type system
- Fields
- Lists
- Field nullability
- scalar
- object
- enum
- union
- Beyond
What is GraphQL
GraphQL is a query language for your API and a server-side runtime for executing queries using a type system that you define for your data. GraphQL isn't tied to any specific database or storage engine and is supplied by your existing code and data.
Type language
GraphQL can be used with any language. They can as they don't rely on anyone specific language's syntax. It uses it's own simple language to define the data. GraghQL is defined with the schema definition language (SDL) shown below. This allows it to easily work with many different languages.
Schema definition language (SDL)
Schema definition language is an intuitive way of creating a legible and well-structured way of defining data.
An example of a schema for a student and a school.
type Student {
name: String
school: School
}
type School {
name: String
students: [Student]
}
Schemas define the collection of data types and the relations between such defined types. Here, each student has a school they attend, and the school has a list of students who attend it.
However, the schema doesn't care how it got the data or how it may be stored. It doesn't care about the implementation.
The power of explicitly defining what data they want can significantly reduce load and optimize the query.
Type system
Let's start with an example:
The query
{
character{
name
appearsIn
}
}
First we select the character field
Then in the character field we select both name, and the appearsIn field
The shape of a GraphQL query closely matches the result. It allows you to be able to predict what the query will return without knowing much about the server. But you want to know what you can ask for. That's where the schema comes in.
The below is an example of what could be returned. The returned data is by default in the JSON format.
The JSON result
{
"character": {
"hero": {
"name": "HULK",
"appearsIn": [
"MARVELCOMICS",
"THEINCREDIBLEHULK",
"THEINCREDIBLEHULKRETURNS"
]
}
}
}
Due to the nature of the GraphQL queries, you can predict what you should be getting in return. So you can quickly just work with that without much difficulty nearly instantly after creating your query and testing that everything is working and fluid.
Fields
Fields are the structure for features for a schema type that you may define.
type Student {
name: String
school: School
}
type School {
name: String
students: [Student]
}
In this example, the fields for the student type are 'name' and 'school.'
When creating a schema type, you define at least one field, maybe more.
Here is an example with the student having a couple more fields. The extra fields are grade which is an Int, and gender which is a String.
type Student {
name: String
school: School
grade: Int
gender: String
}
type School {
name: String
students: [Student]
}
The field returns data, and the types of data returned are scalar, object, enum, and union.
Lists
Fields can also return lists specified by putting [] around the field return type.
You would want to use this when you want to return a list for example a list of people attending a party rather than looking at each letter and seeing if they said yes or no it would be nicer to have it in a list so you know who is coming.
type Author {
name: String
books: [Book] # A list of Books
}
Field nullability
The default setting is to all for the schema to all the return of null values, but it can be disabled with an exclamation mark '!'
An example of when you may want to do it is one for debugging, but another would be if returning null is causing problems in your program and so you have it be caught here instead.
type Author {
name: String! # Cannot return null
books: [Book] # A list of Books
}
If a null value is returned for a non-nullable field, the server will throw an error.
Scalar
Scalar types are the primitive types that you are likely used to. Such as Int, Float, String, Boolean, and ID.
- Int: A signed 32-bit integer
- Float: A signed double-precision floating-point value
- String: A UTF‐8 character sequence
- Boolean: true or false
- ID(serialized as a String): A unique identifier
Examples:
type Robot {
name: String
producer: Producer # Object
serial-code: ID
condition: Float
power-on: Boolean
}
type Producer {
name: String
robots: [String] # List of the robots made by this producer
}
Custom scalar types can be made. To learn more, check out the official documentation linked at the bottom.
Object
Object types are the most common type in GraphQL schemas. They contain collections of fields, each of which has its type.
They are the data representation for things and their characteristics
The below is an example showing of two object being defined and also being used as return types for some of the fields.
type Student { # How you define an object
name: String
school: School # A field that returns an object
}
type School {
name: String
students: [Student] # Returns a list of objects
}
Enum
Short for enumeration, an enum variable type can be throughout programming. The idea is that instead of using an int to represent a set of values, a type with a restricted set of values is used instead.
Enums allows you to define a new layer of abstraction by giving the ability to create a new type to use in your program. This allows you to think more pratically about what you are making, but improves the quality of the code as it harder to make a mistake with enum as it gives syntax highlighting.
One example with the RGB colors.
enum AllowedColor {
RED
GREEN
BLUE
}
Another example could be done with stages of a game
enum Stage {
INTRODUCTION
MIDDLEPHASE
END
}
They are best used when the user may only pick from a given list like the colors red, green, and blue.
Union
Similar to the enum type, but you can use object types in the union with a specified list. It allows for a field to return more than one type of object like shown in the below example with books and movies.
union Media = Book | Movie
type Query {
allMedia: [Media] # This list can include both Book and Movie objects
}
union SearchResult = Book | Author
type Book {
title: String!
}
type Author {
name: String!
}
Beyond
Learn more with the official documentation docs or through a popular platform supporting graphql Apollo Apollo Graphql docs.